Question
Write a program in C that can storing and maintaining a linked list , complete the linkedlist.c and use main.c to test it. Initialize a
Write a program in C that can storing and maintaining a linked list, complete the linkedlist.c and use main.c to test it.
Initialize a single node from a passed string (2 mark)
Add a "push" function to add a node to the front of the list (2 marks)
Add a "pop" function to remove the first node of a linked list and return a pointer to said node (2 marks)
Deleting Nodes (2 marks)
--Delete a single node and free any allocated memory from inside the node (1 mark)
--Delete te entire linked list, delete all nodes iteratively (1 mark)
Reverse order of the linked list (4 marks)
It is recommended that you write the program with a "bottom up" approach. This just means developing the smallest functions of the program first. This gives you the benefit of testing and debugging the most simple functions rather than trying to find errors in larger more complicated code. Modular programming also forces developers to plan solutions in depth and be more analytical about the expectations of data passed. This is unlike procedural programming, where many developers jump into development without a plan.
This is not mandatory, but here is an example of a bottom up approach,
Start by setting up the linked list testing. You will need choose how you will store/read strings into the linked list (i.e. command line arguments, hard coded strings, from a file). --Write and test the newNode function to copy a string into a new node. --Write the code to print a single node. --Write code to delete a node. --Add nodes (push) to a list and repeat steps 3 and 4 for a list. --Now that you know it's all working, complete the reverseOrder function. Hint: NULL is your friend. Take advantage of setting pointers to NULL before allocating memory to point to, using NULL is normally helpful in the future.
lab07.h
/***** * Standard Libraries *****/
#include
/***** * Self-defined Data Structures *****/
/* The listNode data type for storing entries in a linked list */ typedef struct listNode Node; struct listNode { char *data; Node *next; };
/***** * Function Prototypes for linkedList.c *****/
/***** Mandatory Functions *****/
/* Mandatory: Frees all allocated memory associated with the list pointers iteratively */ void deleteList(Node **list);
/* Mandatory: Frees all allocated memory associated with a single node */ void deleteNode(Node **toDelete);
/* Mandatory: Allocates memory for a new string and returns a pointer to the memory */ Node *newNode(char *string);
/* Mandatory: Removes a node from the front of a list and returns a pointer to said node */ Node *pop(Node **list);
/* Mandatory: Adds a node to the front of a list */ void push(Node **list, Node *toAdd);
/* Mandatory: Return a list of pointers in order */ void reverseOrder(Node **list);
/***** Optional Functions *****/
/* Optional: Prints the string stored in a single node */ void printNode(Node *singleNode);
/* Optional: Prints an entire linked list. Nodes are printed from first to last. */ void printLinkedList(Node *linkedList);
linkedList.c
#include "lab07.h"
/***** * Mandatory Functions *****/
/* Mandatory: Frees all allocated memory associated with the list pointers iteratively */ void deleteList(Node **list) {
}
/* Mandatory: Frees all allocated memory associated with a single node */ void deleteNode(Node **toDelete) { }
/* Mandatory: Allocates memory for a new string and returns a pointer to the memory. */ Node *newNode(char *string) {
}
/* Mandatory: Removes a node from the front of a list */ Node *pop(Node **list) {
}
/* Mandatory: Adds a node to the front of a list */ void push(Node **list, Node *toAdd) {
}
/* Mandatory: Return a list of pointers in order */ void reverseOrder(Node **list) { /* Hint: your solution should not need to allocate memory */ /* remember push and pop... */
}
/***** * Optional Functions *****/
/* Optional: Prints the string stored in a single node */ void printNode(Node *singleNode) { }
/* Optional: Prints an entire linked list. Nodes are printed from first to last. */ void printLinkedList(Node *linkedList) {
}
main.c
#include "lab07.h"
int main(int argc, char *argv[]) { return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
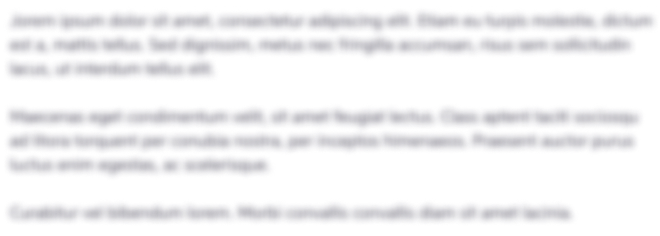
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started