Question
write a program in JAVA that simulates a bookstore database. You will need to write 3 classes Media.java (an abstract class) Book.java (subclass of
- write a program in JAVA that simulates a bookstore database.
- You will need to write 3 classes
- Media.java (an abstract class)
- Book.java (subclass of Media.java)
- TwiceSoldTales.java (bookstore)
- Also, one interface (in addition to the Comparable interface):
- Catalogue.java
- You will also be working with an input file of data about some books.
- Make a new project folder in Eclipse to store the above 3 classes.
- Next, place a new textfile named books.txt into your project folder, and copy and paste the below data into this file:
- Make sure the file is placed in the overall project folder, not under the src folder
The Time in Between Maria Duenas 9.86 43-453-44 2 Bleak House Charles Dickens 8.99 35-678-97 4 Rebecca Daphne Dumaurier 5.50 32-423-82 5 A Room with a View E.M. Forster 7.50 11-778-89 3 Outlander Diana Galbadon 19.95 54-665-65 7 Jane Eyre Charlotte Bronte 7.90 23-456-74 4 The Hunger Games Suzanne Collins 6.90 42-323-22 10 The Woman in White Wilkie Collins 10.75 32-567-89 2 A Face like Glass Frances Hardinge 15.95 44-554-43 1 Lady Audley's Secret Mary Elizabeth Braddon 5.50 12-121-34 1 Murder on the Orient Express Agatha Christie 2.99 98-375-83 8 Middlemarch George Elliot 12.50 12-567-43 4 Our Souls at Night Kent Haruf 11.99 78-474-89 2 Fangirl Rainbow Rowell 10.79 24-137-25 6 Ramona Blue Julie Murphy 9.99 93-283-11 4 The Rosie Project Graeme Simsion 14.99 82-389-31 5 A Is for Alibi Sue Grafton 4.55 34-323-21 3 Still Life Louise Penny 18.99 33-443-22 5 Flight Behavior Barbara Kingsolver 9.98 56-382-34 4
- Next, copy and paste the below starter code into your files and implement the methods according to the comments provided.
Media.java:
- Please do not add any new methods to this class. Instead, write the methods whose signatures are provided.
/** * Media.java * @author
* @author
* CIS 36B, Lab 7 */ public abstract class Media { private String title; private double price; private int numCopies; /** * Default constructor for the Media * class. Calls the three argument * constructor, passing in "title unknown", * 0.0 and 0 */ public Media() { } /** * Constructor for the Media class * @param title the media title * @param price the sale price * @param numCopies the number of copies * currently in stock */ public Media(String title, double price, int numCopies) { } /** * Returns the title of the media * @return the title */ public String getTitle() {
return "";
} /** * Returns the price of the media * @return the price */ public double getPrice() {
return 0.0;
} /** * Returns the current number of * copies available * @return the number of copies */ public int getNumCopies() {
return 0;
} /** * Returns whether there are more than * 0 copies of the media * @return whether any copies are * available to purchase */ public boolean availableToPurchase() {
return false; } /** * Sets the price to be a new price * @param price the price of the media */ public void setPrice(double price) { } /** * Determines how to increase the price * each time a copy gets sold (and media * gets rarer) */ public abstract void updatePrice(); /** * Decrements the number of copies * when a media gets sold */ public void decreaseCopies() { }
/**
* Updates the number of copies
* @param newCopies the number of * copies to add to numCopies */ public void updateNumCopies(int newCopies) { numCopies += newCopies;
}
}
Book.java:
- Note, consult the Oracle documentation on DecimalFormat(Links to an external site.) for more information.
- Please do not add any new methods to this class. Instead, write the methods whose signatures are provided.
- Note that you will need to update the signature of the class to implement the Comparable interface (-5 points for not making this update)
/** * Book.java * @author
* @author
* CIS 36B, Lab 7 */
import java.text.DecimalFormat;
public class Book extends Media { //update this signature! private String author; private String isbn; private static int numBooks = 0; /** * Default constructor for Book. * Calls the 5 argument constructor * Sets title default to "title unknown" * Sets author default to "author unknown"
* Sets price to 0.0
* Sets isbn to "0"
*/ public Book() { } /** * Two argument constructor for the Book * class. Calls the 5 argument constructor * Sets price to a default of 0.0, * isbn to a default of "0" and numCopies * to a default of 0 * @param title the book's title * @param author the book's author */ public Book(String title, String author) { } /** * Constructor for book. Calls media constructor * @param title the book's title * @param author the book's author * @param price the book's price * @param isbn the book's 7-digit isbn * @param numCopies the current num copies */ public Book(String title, String author, double price, String isbn, int numCopies) { } /** * Returns the first and last names * of the author * @return the author */ public String getAuthor() {
return "";
} /** * Returns the 7 digit ISBN number * @return the ISBN number */ public String getISBN() {
return "";
} /** * Returns the total number of books * @return the value of numBooks */ public static int getNumBooks() {
return 0;
} /** * Increases the price of the book by * $0.25 each time a copy is sold */ @Override public void updatePrice() { } /** * Updates numBooks variable by * a specified amount * @param n the number of books to add */ public static void updateNumBooks(int n) { } /** * Overrides equals for Object using the * formula given in class. For the purposes * of this assignment, we will consider * two books to be equal on the basis of * title and author only * @return whether two books have the same * title and author */ @Override public boolean equals(Object o) {
return false;
} /** * Creates a book String in the following format * Title: * Author: * Price: $ * ISBN #: * Copies: * Note that there are no <> around the values * The <> are standard in programming * to indicate fill in here */ @Override public String toString() { DecimalFormat df = new DecimalFormat("#.##"); return " Price: $" + df.format(getPrice()); }
/** * Compares two Books. * Returns 0 if the two Books are equal * If the two books have the same title * returns compareTo of the authors * Otherwise, returns compareTo of the titles */ public int compareTo(Book b) {
return -1;
}
}
Catalogue.java Interface
- Add a new interface to your project.
- TwiceSoldTales.java (below) will implement the Catalogue interface
/*
* Catalogue.java
* @author * CIS 36B, Lab 7 */ import java.io.IOException; import java.util.Scanner; public interface Catalogue { /** * Reads from a file and populates the catalogue * @param input the Scanner used to read in the data */ void populateCatalogue(Scanner input) throws IOException; /** * Searches for m in the catalogue * @param m the Media to locate * @return the location of m in * the catalogue */ int binarySearch(Media m); /** * Sorts the catalogue into * ascending order */ void bubbleSort(); /** * Prints a menu of options to interact * with the catalogue */ void printMenu(); /** * Prints out the current catalogue */ void printStock(); }
TwiceSoldTales.java:
- Please add any additional methods to this class that you find necessary, in addition to implementing those that have been provided.
/** * TwiceSoldTales.java * Note: the name of an awesome * used bookstore in Seattle, WA! * @author
* @author
* CIS 36B, Lab 7 */ import java.util.ArrayList; import java.util.Scanner; import java.io.File; import java.io.IOException; public final class TwiceSoldTales { //update the signature to inherit from Catalogue private ArrayList books = new ArrayList(); private static final String filename = "books.txt"; public static void main(String[] args) throws IOException { TwiceSoldTales t = new TwiceSoldTales(); String title, author, isbn; double price;
int numCopies;
File file = new File(filename); Scanner input = new Scanner(file);
input.close();
} }
- Update TwiceSoldTales.java to implement the Catalogue interface
- This class should then offer a menu of options to the user
- Option A: Search for a book
- This menu option should take in a title and author, and call the two argument constructor of the Book class
- It should then pass this new Book object into binarySearch to locate the book in the Catalogue.
- If the book is not in the Catalogue, it should print a message that the book is not in stock
- If the book is in stock, it should ask the user if they would like to purchase the book.
- When a book is purchased, the number of copies should decrease (call updateNumCopies)
- Please see below example output for more information
- Option B: Sell a Book
- It should also prompt the user for the price they paid to buy the book originally.
- See this section highlighted below.
- Note that the price offered to the user should be one quarter (25%) the amount the user entered.
- This menu option should should prompt the user for a title, author, isbn and price.
- If the book is not one that is currently in the catalogue, then the store should offer to purchase the used book. In this case:
- If the book is in the catalogue, it should offer to purchase the book for one quarter (25%) of the book's listed price.
- In both cases, your program should search for the book using binarySearch. If the book is not in the catalogue, it should be added into the ArrayList. If it already exists in the Catalogue, the number of copies should be increased.
- Option C: Print Catalogue
- This menu option should print out the entire ArrayList by calling the printStock method
Option X: Exit
- This option should end the program with the message "Please come again!"
- Any other options entered should provide a message "Invalid Option"
Sample Output - Your Output Must Work Identically to What is Shown Below
Welcome to Twice Sold Tales! We currently have 80 books in stock! Please select from one of the options: A. Search for a book to purchase B. Sell a book C. Print catalogue X. Exit Enter your choice: A Enter the book information below: Title: Flight Behavior Author: Barbara Kingsolver We have Flight Behavior in stock! Title: Flight Behavior Author: Barbara Kingsolver Price: $9.98 ISBN #: 56-382-34 Copies: 4 Would you like to purchase it (y/n): y Thank you for your purchase! Please select from one of the options: A. Search for a book to purchase B. Sell a book C. Print catalogue X. Exit Enter your choice: z Invalid option! Please select from one of the options: A. Search for a book to purchase B. Sell a book C. Print catalogue X. Exit Enter your choice: z Invalid option! Please select from one of the options: A. Search for a book to purchase B. Sell a book C. Print catalogue X. Exit Enter your choice: A Enter the book information below: Title: A Man Called Ove Author: Fredrik Backman Sorry! We don't carry that title right now. Please select from one of the options: A. Search for a book to purchase B. Sell a book C. Print catalogue X. Exit Enter your choice: A Enter the book information below: Title: A Is for Alibi Author: Sue Grafton We have A Is for Alibi in stock! Title: A Is for Alibi Author: Sue Grafton Price: $4.55 ISBN #: 34-323-21 Copies: 3 Would you like to purchase it (y/n): n Please select from one of the options: A. Search for a book to purchase B. Sell a book C. Print catalogue X. Exit Enter your choice: B Enter the book information below: Title: A Is for Alibi Author: Sue Grafton Thank you! We will purchase the book for $1.14 Please select from one of the options: A. Search for a book to purchase B. Sell a book C. Print catalogue X. Exit Enter your choice: B Enter the book information below: Title: B Is for Burgler Author: Sue Grafton
ISBN #: 34-323-45
Please enter the price you paid: $10.95
Thank you! We will purchase the book for $2.74
Please select from one of the options: A. Search for a book to purchase B. Sell a book C. Print catalogue X. Exit Enter your choice: C Current Selection of Books: Title: A Face like Glass Author: Frances Hardinge Price: $15.95 ISBN #: 44-554-43 Copies: 1 Title: A Is for Alibi Author: Sue Grafton Price: $4.55 ISBN #: 34-323-21 Copies: 4 (Note this value got updated from 3 to 4 when a new copy was purchased) Title: A Room with a View Author: E.M. Forster Price: $7.50 ISBN #: 11-778-89 Copies: 3
Title: B Is for Burgler Author: Sue Grafton
Price: $10.95
ISBN #: 34-323-45
Copies: 1
Title: Bleak House Author: Charles Dickens Price: $8.99 ISBN #: 35-678-97 Copies: 4 Title: Fangirl Author: Rainbow Rowell Price: $10.79 ISBN #: 24-137-25 Copies: 6 Title: Flight Behavior Author: Barbara Kingsolver Price: $10.23 ISBN #: 56-382-34 Copies: 3 Title: Jane Eyre Author: Charlotte Bronte Price: $7.90 ISBN #: 23-456-74 Copies: 4 Title: Lady Audley's Secret Author: Mary Elizabeth Braddon Price: $5.50 ISBN #: 12-121-34 Copies: 1 Title: Middlemarch Author: George Elliot Price: $12.50 ISBN #: 12-567-43 Copies: 4 Title: Murder on the Orient Express Author: Agatha Christie Price: $2.99 ISBN #: 98-375-83 Copies: 8 Title: Our Souls at Night Author: Kent Haruf Price: $11.99 ISBN #: 78-474-89 Copies: 2 Title: Outlander Author: Diana Galbadon Price: $19.95 ISBN #: 54-665-65 Copies: 7 Title: Ramona Blue Author: Julie Murphy Price: $9.99 ISBN #: 93-283-11 Copies: 4 Title: Rebecca Author: Daphne Dumaurier Price: $5.50 ISBN #: 32-423-82 Copies: 5 Title: Still Life Author: Louise Penny Price: $18.99 ISBN #: 33-443-22 Copies: 5 Title: The Hunger Games Author: Suzanne Collins Price: $6.90 ISBN #: 42-323-22 Copies: 10 Title: The Rosie Project Author: Graeme Simsion Price: $14.99 ISBN #: 82-389-31 Copies: 5 Title: The Time in Between Author: Maria Duenas Price: $9.86 ISBN #: 43-453-44 Copies: 2 Title: The Woman in White Author: Wilkie Collins Price: $10.75 ISBN #: 32-567-89 Copies: 2 Please select from one of the options: A. Search for a book to purchase B. Sell a book C. Print catalogue X. Exit Enter your choice: X Please come again!
Once your program is working identically to what is shown, upload Book.java, Media.java and TwiceToldTales.java to Canvas
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Heres the complete implementation for the bookstore database program Mediajava Java Mediajava author CIS 36B Lab 7 public abstract class Media private ...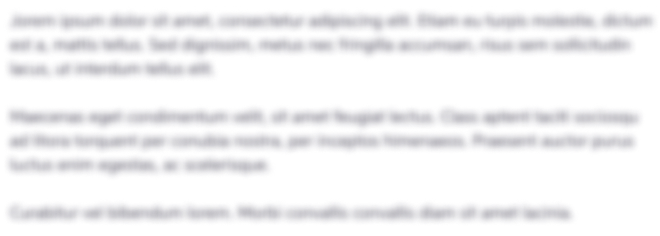
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started