Question
Write a program named Bank that maintains a database of bank accounts. Each account will be stored in a BankAccount object. The following example shows
Write a program named Bank that maintains a database of bank accounts. Each account will be stored in a BankAccount object. The following example shows what the user will see. O: Open account D: Deposit S: Select account C: Close account W: Withdraw L: List all accounts Q: Quit current account selected: NONE Enter command: O Enter new account number : 123-456 (Validate: No duplicates) Enter initial balance: 100:00 O: Open account D: Deposit S: Select account C: Close account W: Withdraw L: List all accounts Q: Quit current account selected: 123-456 Balance: $100.00 Enter command: The program repeatedly prompts the user to enter commands which it then executes until the user enters the command Q. Note the list of commands is redisplayed after each command has been executed, along with the current account and its balance. If there is no current account, the message None selected is displayed instead of the account number Here are descriptions of other commands: Open account: The user is prompted to enter a new account number and initial balance in to a new BankAccount object. BankAccount objects for all existing accounts must be stored in an array. The new account becomes the current account. (Validate: duplicate account numbers should not be allowed) Close account: If there is no current account the message Please select an account is displayed. Otherwise, the current account is removed from the array. There is no current account after this operation is completed Upon closing an account, the last account object will be moved to the position of the account that is being closed. For example if there are 5 accounts and the user closes the 3rd account in the array, then the 5th account will take the place of the 3rd account(You do not have to resize the array to make it smaller). By doing this you will not have any empty gaps in your array. You do not have to worry about this if after closing the account there is only one bank object left in the array or if you are closing the last account object in the array. Deposit. If there is no current account, the message Please select an account is displayed. Otherwise, the user is prompted to enter the amount of the deposit. Enter amount of deposit: 50.00 The amount entered by the user is added to the balance of the current account. Withdraw: If there is no current account, the message Please select an account is displayed. Otherwise the user is prompted to enter the amount of the withdrawal: Enter amount to withdraw: 50.00 The amount entered by the user is subtracted from the balance of the current account. (VALIDATION: Make sure that there is at least $1.00 in the account after withdrawal, otherwise display the message You are withdrawing too much: try again) Select account: The user is prompted to enter an account number Enter account number : 123-456 The array is then searched to see if any BankAccount object contains this number. If so, the object becomes the current account. If not, the following message is displayed: Account number was not found List all accounts: Displays the list of all the account numbers and each individual balance along with the total bank assets at the end. If there are no accounts display No accounts For example: 1) Acct #: 123-456 Bal: $200.00 2) Acct#: 111-222 Bal: $300.50 3) Acct#: 222-333 Bal: $450.55 Total Bank assets: $951.05 Here are a few things to keep in mind: The array used to store BankAccount objects must contain only one element initially. When the array becomes full, it must then be doubled in size. Duplicate account numbers should not be allowed Upon closing an account, the last account object will be moved to the position of the account that is being closed. For example if there are 5 accounts and the user closes the 3rd account, then the 5th account will take the place of the 3rd account(You do not have to resize the array to make it smaller). By doing this you will not have any empty gaps in your array. You do not have to worry about this if there is only one bank object in the array or if you are closing the last account object in the array. Note: Please keep it simple(so, I can understand). Language: In java programming languge : Java
Step by Step Solution
There are 3 Steps involved in it
Step: 1
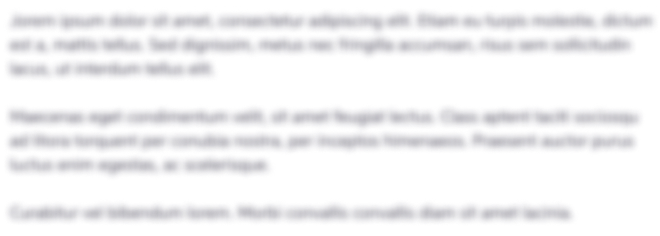
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started