Question
Write a program that asks for the customers last and current meter reading in Kilowatt Hours (KwHs). Determine the amount of usage for the month
Write a program that asks for the customers last and current meter reading in Kilowatt Hours (KwHs). Determine the amount of usage for the month and calculate a sub total (before tax) and a total amount due (tax included) using the following constraints.
This is my 3rd time submitting this to cheggs. Can someone help me with this please. Thank you in advance for any assistance you can give me. I'm not doing well grasping this java stuff.
Constraints
Rate A: For 500 KwHs or less = $0.0809 / KwH
Rate B: For 501 to 900 KwHs = $0.091 / KwH
Rate C: For greater than 900 KwHs = $0.109 / KwH Utilities Tax is 3.46% regardless of usage. Requirements
Use a method to determine the usage
Use a second method to determine the tax
Use a third method to determine the subtotal
Format all output as follows:
o Usage to 1 decimal place, KhWs
o Rate to 4 decimal places, monetary
o Subtotal to 2 decimal places, monetary
o Taxes to 2 decimal places, monetary
o Total to 2 decimal places, monetary
Implement a loop to return and enter new values (run the program again) if the user wishes to
Hints
The type of loop can be of your choosing
Make sure you use Java coding conventions
Expected Output
Below is a sample run with three iterations. User input in BOLD
Welcome to the City Power Bill Calculator! Please enter your previous meter reading: 750
Please enter your current meter reading: 1250
Your usage was: 500.0 KwHs
Your rate was: $0.0809/KwH
Your subtotal is: $40.45
Taxes are: $1.40
Your total bill this month is: $41.85
Would you like to calculate a new usage? (Y for Yes, N to exit): y
Please enter your previous meter reading: 750
Please enter your current meter reading: 1350.63
Your usage was: 600.6 KwHs
Your rate was: $0.0910/KwH
Your subtotal is: $54.66
Taxes are: $1.89
Your total bill this month is: $56.55
Would you like to calculate a new usage? (Y for Yes, N to exit): y
Please enter your previous meter reading: 750.39
Please enter your current meter reading: 1655.37
Your usage was: 905.0 KwHs
Your rate was: $0.1090/KwH
Your subtotal is: $98.64 Taxes are: $3.41
Your total bill this month is: $102.06
Would you like to calculate a new usage? (Y for Yes, N to exit): n
Thank you for using this program. Goodbye!
Below the code in BOLD is my code.
package venkanna;
import java.util.Scanner;
public class CurrentReading
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
char ch;
do
{
double subtotal=0;
System.out.println("Welcome to The City Power Bill Calculator");
System.out.println("Please Enter Your Previous meter Reading:");
int prev=sc.nextInt();
System.out.println("Please Enter Your Current meter Reading:");
int current=sc.nextInt();
double reading=current-prev;
System.out.println("Your Usage was:"+reading+" KwHs");
if(reading<=500) { System.out.println("Your Rate was: $0.0809/Kwh");
subtotal=reading*0.0809;
}
else if(reading>500&&reading<=900)
{
System.out.println("Your Rate was: $0.091/Kwh");
subtotal=reading*0.091;
}
else if(reading>900)
{
System.out.println("Your Rate was:$0.109 / KwH:");
subtotal=reading*0.109;
}
System.out.println("Your Sub-Total was:$"+subtotal);
double tax=(subtotal*3.46)/100;
double total_bill=subtotal+tax;
System.out.println("Taxes are $"+tax);
System.out.println("Your total bill this month is:$"+total_bill);
System.out.println("Would you like to calculate new Usage(y for yes N for No)");
ch = sc.next().trim().charAt(0);
}
while(ch=='Y'||ch=='y');
sc.close();
}
}
Below are the comments from my instructor
There are some issues with this code: Place this line: System.out.println("Welcome to The City Power Bill Calculator"); outside the do loop. No need to see that with each iteration. Rates and tax are set amounts. They should be final variables and passed by reference. The biggest issue I see is that you did not use methods at all. So, I am attaching some skeleton code (s-code) for you. This code has some code but mostly commenting with instructions on how to build this program.
Below is a Skeleton code my instructor would like me to work in. Can anyone one help me with this?
package week6_Skeleton; /**
* @Course: SDEV 250 ~ Java Programming I
* @Author Name: * @Assignment Name:
* @Date: Oct 17, 2016 * @Description:
*/ //
Imports import java.util.Scanner;
//Begin Class Week6_Skeleton
public class Week6_Skeleton {
//Begin Main Method
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
//Variable for do loop response
String ans = "";
/**
* Other variables would be best defined here. Rates and the tax are set
* values so they should be final. Placing those at the top would allow
* easy changes if rates and taxes were to ever change.
*/
System.out.println("Welcome to the City Power Bill calculator! ");
//Begin loop
do{
System.out.print("Please enter your previous meter reading: ");
double previousReading = sc.nextDouble();
System.out.print("Please enter your current meter reading: ");
double currentReading = sc.nextDouble();
/**
* Assign a variable to the method call and send the two variables
* that were just input from the user.
*/
double usage = MyUsage(previousReading, currentReading); //See method MyUsage below
/**
* Now that we have the variable usage defined with the method
* call and the variables have been sent to the method, the variable
* usage will now be set equal to the result returned from the method.
* next line of code should be moved to the output statements below.
* This was just to show you how the method produces the correct
* result.
*/
/**
* Assign the rates defined in variables above with a series of if &
* else/if statements.
*/
/**
* Now that you have usage and the rate assigned, call a method
* SubTot to determine subtotal Assign a variable to the method call
* similar to the way usage was above. Send usage & rate to the
* method
*/
/**
* Next, call a method to determine tax based on SubTot. Assign a
* variable to the method call similar to the way usage was above.
* Send subTot & the variable that you will define up-top for the tax
* rate to the method
*/
/**
* Now that you have the subtotal and tax amount, you can use those
* to determine final total. This can be one line of code and does
* not have to be a method call.
*/
/**
* Now you can do the outputs to output all of the results "Your usage was:" "Your rate was:" "Your subtotal is:" "Taxes are:"
* "Your total bill this month is:" The first one is below.
*/
System.out.printf("The usage was: %.2f ", usage);
//Other required outputs here
//Exit the program?
System.out.print("Would you like to run the program again? (Y for Yes, N for No): ");
ans = sc.next();
//End loop
}
while (ans.equalsIgnoreCase("y"));
//Ignores case (upper or lower)
System.out.println("Thank you for using the program Goodbye.");
}
//End Main Method
/**Below is a proper method header above the method. To have NetBeans do this
* for you, write the method. Then, place your cursor above the p in private
* and hit enter. The compiler will add most of the header for you. Then you
* can edit the header to look like the one below.
*/
/**
* Method MyUsage: Determine the power usage.
*
* @param prev
* @param curr
* @return customer usage
*/
private static double MyUsage(double prev, double curr) {
return curr - prev; //SMH Return the difference.
}
}//End Class Week6_Skeleto
Step by Step Solution
There are 3 Steps involved in it
Step: 1
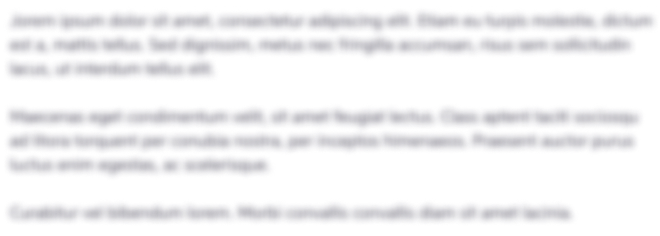
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started