Question
Write a program that consists of multiple source files to parse the header information from a ppm image. Some ppm images and some starter code
Write a program that consists of multiple source files to parse the header information from a ppm image. Some ppm images and some starter code will be provided. You will need to write the function to parse the header, as well as a makefile, and a header file. The files from this lab will be starter files for the third programming assignment. Background Information For this lab, you will write a program that parses the header of a ppm image and prints out the dimensions of the image to the user. This will be used as starter code for your parsing function for PA3 in lecture. - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - ppm Images As you have learned in lecture, ppm images are a type of image format. It is easy to write and analyze programs to process this type of image. There are two parts to ppm images: the header and the pixel data. The header consists of a special tag indicating the type of image we will be using images with the P6 tag. The dimensions follow that, with columns stated first then rows; and then the largest value for any pixel, which is usually 255. If you open an image file using an editor like vim, you can see the header information at the top of the file. The image pixel data immediately follows the newline character after the 255, and is binary data, so it is not anything that will make any sense when viewing the file with an editor like vim. - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - Command-Line Arguments You also saw in lecture that when programs that use command-line arguments, the main() function signature changes from int main(void) { to int main(int argc, char *argv[]) { where argc holds the number of items entered at the command-line, and argv is the array that holds each item (or actually, a pointer to each item) that was entered at the command-line. For example, with the reverse_echo program from the previous lab, you saw that to run that program, you would type something like the following: ./reverse_echo testing 1 2 3 In that case, the value of argc would be 5, and the argv array would look like the following: argv[0] ------- argv[1] ------- argv[2] ------- argv[3] ------- argv[4] ------- argv[5] ------- * * * * * * ./reverse_echo testing 1 2 3 \0 2 For this lab, and for the third assignment, your program will use command-line arguments. To run the program, you will type the executable followed by the image file name, such as: ./a.out tiger.ppm - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - Pointers In yesterdays lecture, you were introduced to pointers. You will use pointers in this lab for the parameters of the parseHeader() function that you will be writing. As you know, functions can only return one value, but we need to get two values from the header information of the image file: the number of columns and the number of rows. You also know by now that variables that are passed by value to functions are copied into the function parameters. When the function ends, those local copies go away and any changes that were made to those local copies in the function are not reflected back in the arguments that were sent in to that function. So to be able to get more than one value changed or assigned or modified from a function, addresses to the variables are sent in to the function. This is called pass by reference. An example of a function using pass by reference can be found in the swapPBR.c source file found on Canvas in the module for Chapter 10, Pointers). When the function assigns the values to those address locations sent in to the function parameters, the variables sent in will contain those values too because those function parameters point to those very same memory locations. - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - File Pointers Also covered in lecture earlier were file pointers. The general form for declaring a file pointer is the following: FILE *
Step by Step Solution
There are 3 Steps involved in it
Step: 1
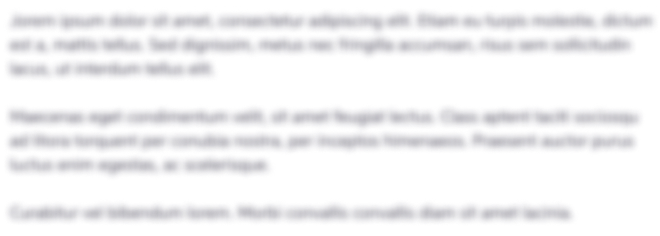
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started