Question
Write a program that generates an array of N positive integers randomly. Allow the user to enter any integer bigger than 0. Then sort them
Write a program that generates an array of N positive integers randomly. Allow the user to enter any integer bigger than 0. Then sort them using Insertion Sort. The algorithm is as follows (this is pseudo-code so it isn't real Java code): for i 1 to length(Array) - 1 j i while j > 0 and Array[j-1] > Array[j] swap Array[j] and Array[j-1] j j - 1 end while end for
Requirements: You need the following methods. Call these from main: int[] createRandomArray(int n): Create an array of int of n size. Don't worry about duplicates. Then use a for loop to create n random numbers to store in the array. The numbers should be chosen in a range from 1 to 2 x n. void sort(int array[]): Pass in random array and sort the numbers in ascending order. You don't need to pass back the array as Insertion sort works on the array. Swaping elements in the array can be done in a separate method but this is optional. void printArray(int array[]): Print out entire array.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
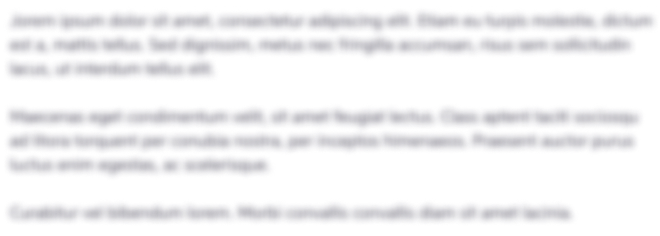
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started