Question
Write a program that, given an integer, sums all the numbers from 1 through that integer (both inclusive). Do not include in your sum any
Write a program that, given an integer, sums all the numbers from 1 through that integer (both inclusive). Do not include in your sum any of the intermediate numbers (1 and n inclusive) that are divisible by 5 or 7.
Input:
Your program should read lines from standard input. Each line contains a positive integer.
Output:
For each line of input, print to standard output the sum of the numbers from 1 through n, disregarding those divisible by 5 and 7. Print out each result on a new line.
The code is then
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.nio.charset.StandardCharsets;
public class Main { /** * Iterate through each line of input. */ public static void main(String[] args) throws IOException { InputStreamReader reader = new InputStreamReader(System.in, StandardCharsets.UTF_8); BufferedReader in = new BufferedReader(reader); String line; while ((line = in.readLine()) != null) { System.out.println(line); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
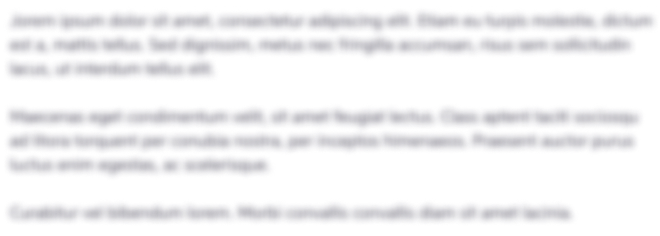
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started