Question
Write a program that outputs the nodes of a graph in a breadth-first traversal. c++ This is what I have so far but I am
Write a program that outputs the nodes of a graph in a breadth-first traversal. c++
This is what I have so far but I am having issues
#include "stdafx.h"
#include
#include
using namespace std;
class graphType {
public:
bool isEmpty() const;
void createGraph();
void clearGraph();
void printGraph() const;
//void depthFirstTraversal();
//Function to perform the depth first traversal of
//the entire graph.
//Postcondition: The vertices of the graph are printed
// using the depth first traversal algorithm.
//void dftAtVertex(int vertex);
//Function to perform the depth first traversal of
//the graph at a node specified by the parameter vertex.
//Postcondition: Starting at vertex, the vertices are
// printed using the depth first traversal algorithm.
void breadthFirstTraversal();
graphType(int size = 0);
~graphType();
protected:
int maxSize; //maximum number of vertices
int gSize; //current number of vertices
unorderedLinkedList
//adjacency lists
private:
void dft(int v, bool visited[]);
//Function to perform the depth first traversal of
//the graph at a node specified by the parameter vertex.
//This function is used by the public member functions
//depthFirstTraversal and dftAtVertex.
//Postcondition: Starting at vertex, the vertices are
// printed using the depth first traversal algorithm.
};
template
{
public:
bool search(const Type& searchItem) const;
void insertFirst(const Type& newItem);
void insertLast(const Type& newItem);
void deleteNode(const Type& deleteItem);
};
bool graphType::isEmpty() const
{
return (gSize == 0);
}
void graphType::createGraph()
{
ifstream infile;
char fileName[50];
int vertex;
int adjacentVertex;
if (gSize != 0) //if the graph is not empty, make it empty
clearGraph(); cout << "Enter input file name: ";
cin >> fileName; cout << endl;
infile.open(fileName);
if (!infile)
{
cout << "Cannot open input file." << endl;
return;
}
infile >> gSize; //get the number of vertices
for (int index = 0; index < gSize; index++)
{
infile >> vertex; infile >> adjacentVertex;
while (adjacentVertex != -999)
{
graph[vertex].insertLast(adjacentVertex);
infile >> adjacentVertex;
} //end while
} // end for
infile.close();
}
void graphType::clearGraph()
{
for (int index = 0; index < gSize; index++)
graph[index].destroyList();
gSize = 0;
}
void graphType::printGraph() const
{
for (int index = 0; index < gSize; index++)
{
cout << index << " ";
graph[index].print();
cout << endl;
}
cout << endl;
}
void graphType::breadthFirstTraversal()
{
linkedQueueType
bool *visited; visited = new bool[gSize];
for (int ind = 0; ind < gSize; ind++)
visited[ind] = false; //initialize the array
//visited to false
linkedListIterator
for (int index = 0; index < gSize; index++)
if (!visited[index])
{
queue.addQueue(index);
visited[index] = true;
cout << " " << index << " ";
while (!queue.isEmptyQueue())
{
int u = queue.front();
queue.deleteQueue();
for (graphIt = graph[u].begin();
graphIt != graph[u].end(); ++graphIt)
{
int w = *graphIt;
if (!visited[w])
{
queue.addQueue(w);
visited[w] = true;
cout << " " << w << " ";
}
}
} //end while
} delete [] visited;
} //end breadthFirstTraversal
graphType::graphType(int size)
{
maxSize = size;
gSize = 0;
graph = new unorderedLinkedList
}
graphType::~graphType()
{
clearGraph();
}
int main()
{
return 0;
}
template
bool unorderedLinkedList
{
bool found = false;
nodeType
//pointer to traverse the list
current = first;
//start the search at the first node
while (current != NULL && !found)
if (current->info >= searchItem)
found = true;
else
current = current->link;
if (found)
found = (current->info == searchItem);
//test for equality
return found;
}
template
void unorderedLinkedList
{
insert(newItem);
}
template
void unorderedLinkedList
{
insert(newItem);
}
template
void unorderedLinkedList
{
nodeType
//pointer to traverse the list
nodeType
//pointer just before current bool found;
if (first == NULL) //Case 1
cout << "Cannot delete from an empty list." << endl;
else
{
current = first;
found = false;
while (current != NULL && !found) //search the list
if (current->info >= deleteItem)
found = true;
else
{
trailCurrent = current;
current = current->link;
}
if (current == NULL) //Case 4
cout << "The item to be deleted is not in the list." << endl;
else if (current->info == deleteItem) //the item to be
//deleted is in the list
{
if (first == current) //Case 2
{ first = first->link;
if (first == NULL)
last = NULL;
delete current;
}
else //Case 3
{
trailCurrent->link = current->link;
if (current == last)
last = trailCurrent;
delete current;
}
count--;
}
else //Case 4
cout << "The item to be deleted is not in the "
<< "list." << endl;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
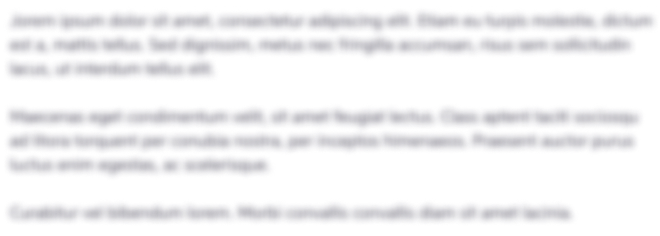
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started