Question
Write a program that plays rock-paper-scissor game. The program randomly generates a number 0, 1, or 2 representing scissor, rock, and paper. The program prompts
Write a program that plays rock-paper-scissor game. The program randomly generates a number 0, 1, or 2 representing scissor, rock, and paper. The program prompts the user to enter a number 0,1, or 2 and displays a message indicating whether the user or the computer wins, loses, or draws. Here is my code for this program.
However, I need to add a loop to allow the user to play until either the Human or the Computer has won 3 times in a row. How would I go about doing this? Using NetBeans 8.1 JAVA.
package problem; import java.util.*; public class problem_1 { public static void main(String[] args) { Scanner input = new Scanner(System.in); // Create scanner object String result = "", symbolA = "", symbolB = ""; System.out.print("Scissor (0), rock (1), paper (2): "); // Prompt user to enter value int choice = input.nextInt(); // Assign users value to integer choice int pick = (int)(Math.random()*3); // Generate a random number, either 0,1, or 2 if(choice==pick) result = "It is a draw"; // If the values equal each other, tell the user it is a draw else { if(((choice==0)&&(pick==2))||((choice==1)&&(pick==0))||((choice==2)&&(pick==1))) result = "You won"; // Tell the user if he won against the computer else result = "You lose"; // Tell the user if he lost against the computer } if (choice==0) symbolA = "scissor"; if (pick==0) symbolB = "scissor"; if (choice==1) symbolA = "rock"; if (pick==1) symbolB = "rock"; if (choice==2) symbolA = "paper"; if (pick==2) symbolB = "paper"; // Assign rock, paper, and scissor to the values generated System.out.println("The computer is " + symbolB + ". You are " + symbolA + ". " + result); //Display the output } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
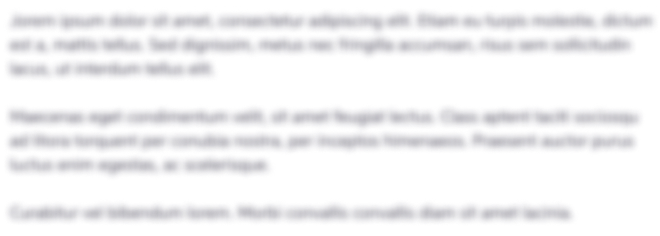
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started