Question
Write a program that prompts the user to enter a file name, then opens the file in binary mode and reads it. The input files
Write a program that prompts the user to enter a file name, then opens the file in binary mode and reads it. The input files are assumed to be a sequence of long integers. The program should read the entire file, sort the numbers and print the comma separated list of long integers on the console. For sorting, you're given a special Sort class file that can sort arrays or ArrayLists.
While entering the file name, the program should allow the user to type quit to exit the program. If the file with a given name does not exist, then display a message and allow the user to re-enter the file name. Each input file may contain any number of long integers. You can use any arrays or ArrayList objects in your program as you wish. All required input files and the Sort class are uploaded in advance and there is no need to upload them with your submission. You should only upload one file: FileSorting.java. You are able to download the input files and the Sort class file to be used in your eclipse project for testing.
Input validation:
a) If the file does not exist, then you should display a message "File somefile.dat is not found." and allow the user to re-enter the file name.
b) If the file is empty, then display a message "File somefile.dat is empty." and exit the program.
Hints:
a) Perform file name input validation immediately after the user entry and use a while loop.
b) You can use DataInputStream class for reading the input file in binary mode.
Grading guide:
1. Functionality: Does the program work and perform basic functionality as per requirements? (Need to be able to compile and run the program with some input and some output):
2. Correctness: Does the program properly sort numbers? Does the program read and display all required lines? -
3. Input Validation: Is the user input validated against requirements? -
4. Output Formatting: Is the output formatted according to the requirements:
5. Edge Cases: Does the program work without errors for ALL values of input (missing file, empty file, very large file, etc.) -
In java language.
here is reqired output
Please enter the file name or type QUIT to exit: File input0.dat is empty.
input1.dat | input0.dat input1.dat input10.dat input100.dat input2.dat input5.dat Sort.java |
Please enter the file name or type QUIT to exit: 1601643005562228485
Test Case 3
Standard Input | Files in the same directory |
---|---|
input3.datENTER input2.datENTER | input0.dat input1.dat input10.dat input100.dat input2.dat input5.dat Sort.java |
Please enter the file name or type QUIT to exit: File input3.dat is not found. Please re-enter the file name or type QUIT to exit: -9202349033351877375, -8462816956357834116
Test Case 4
Standard Input | Files in the same directory |
---|---|
input3.datENTER input4.datENTER input5.dat | input0.dat input1.dat input10.dat input100.dat input2.dat input5.dat Sort.java |
Please enter the file name or type QUIT to exit: File input3.dat is not found. Please re-enter the file name or type QUIT to exit: File input4.dat is not found. Please re-enter the file name or type QUIT to exit: -7565896176379649603, -6149589326294881399, -5651965763595747208, 514266395590023805, 6817002234570720838
Test Case 5
Standard Input | Files in the same directory |
---|---|
input3.datENTER QUIT | input0.dat input1.dat input10.dat input100.dat input2.dat input5.dat Sort.java |
Please enter the file name or type QUIT to exit: File input3.dat is not found. Please re-enter the file name or type QUIT to exit:
Test Case 6
Standard Input | Files in the same directory |
---|---|
input10.dat | input0.dat input1.dat input10.dat input100.dat input2.dat input5.dat Sort.java |
Please enter the file name or type QUIT to exit: -5767292692502522774, -4169140448305022054, -2943435244475216025, -2738128208131209360, 1202165787208679866, 2585265885761591908, 3422147174151445177, 4542974019794787627, 4935473097913448945, 5599150192793579671
Test Case 7
Standard Input | Files in the same directory |
---|---|
input100.dat | input0.dat input1.dat input10.dat input100.dat input2.dat input5.dat Sort.java |
Please enter the file name or type QUIT to exit: -9093892288597671005, -9041465125509896333, -8796509502074588152, -8729903085025641243, -8711345249569510977, -8543737869250479968, -8436732197396647866, -8299938783402889063, -8221573622585309850, -8212617737021167376, -7598541783780612791, -7597812177822245952, -7489795981624024828, -7452552786612835880, -7244645926331683023, -7062739647444280037, -7015725736819532778, -6959389503899408165, -6909675674385396997, -6709647628853939145, -6591233871059848319, -6521015277371523271, -6285614000247699013, -6044256651242237185, -5991519176114591979, -5673680526332951166, -5279171999309886498, -5272730074271413885, -5094932505672377285, -4767735853686971756, -4705680630242474655, -4487644718179080932, -4292987719785389290, -3581855543882657294, -2941778232835849144, -2820759146510706695, -1871949780683505070, -1761174345219882997, -1566421130403766415, -1415940539492099660, -1379780690201096804, -1266193936134150688, -1052899543038715921, -781407836382142280, -689716143654968776, -368168360075926897, -352755662753969408, -57147678790156786, 83557489988067884, 123692134202814875, 258759092784048934, 393316375691417845, 443805085884920184, 718729227331303621, 961437404049337603, 1061546702697866074, 1098563400841174830, 1103593660602145129, 1440821016901262349, 1703591866000406924, 1908033120171155924, 2195078529064499799, 2534413222502413749, 2569670551402808132, 2805801020236385676, 3166152702522760716, 3275209618810358163, 3508534202792913736, 3620135191278080958, 3677982223038316890, 4698763441022480522, 5011819719027860099, 5338023607514735912, 5465984465598519186, 5546630544108528859, 5792831162497161300, 5972895676049968972, 6132438922793014048, 6431907897810225811, 6465282569609276554, 6662910278286277660, 6724007707054033138, 6929975950441067225, 6953163279500090844, 7261856945730834721, 7285771983073060445, 7615435439097062191, 7812451230411144908, 8091059180703303765, 8199511620403064977, 8211957161738559527, 8255974619979120841, 8294618536962744593, 8315428680601813263, 8348558305770004080, 8352642467945766480, 8500988504293898086, 8573234912220269096, 8855050548459609610, 9016902179793090145
Step by Step Solution
There are 3 Steps involved in it
Step: 1
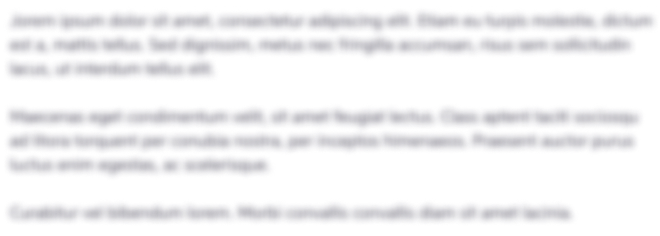
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started