Question
Write a program that prompts the user to enter a Social Security Number in the format DDD-DD-DDDD, where D is a digit. Your program should
Write a program that prompts the user to enter a Social Security Number in the format DDD-DD-DDDD, where D is a digit. Your program should check whether the input is valid.
HINT: Use chatAt() and isDigit() methods.
EX.
INPUT: 111-11-1111
OUTPUT: Valid SSN
---------------------------
INPUT: 11-11-1111
OUTPUT: Invalid SSN
******* I know that I can solve this problem by looping through the characters of the string in the SSN but I want to make the code I wrote beneath work. I am having a problem on my second to last if- statement. In that statement it says that all of my variables d1-d9 "cannot be resolved to variable." I initially thought that this might be a problem with scope of my variables but I dont belive it is. Why am I getting an error and what can I do to make this code work ???*********
import java.util.Scanner;
public class CheckSSN {
public static void main(String [] args) {
Scanner kbd_in = new Scanner(System.in);
System.out.print(" Enter your social security numer in the format DDD-DD-DDDD: ");
String social = kbd_in.nextLine().trim();
System.out.println(" You have entered: "+ social);
kbd_in.close();
//Test to see if length is correct
boolean properLength;
if (social.length() !=11) {
properLength = false;
}else {
properLength = true;
}
//Test to see if dashes are in the correct place
boolean properDash;
if((social.charAt(4)!='-')&&(social.charAt(7)!='-')) {
properDash = false;
}else {
properDash = true;
}
//Variables d1-d9 will hold a T/F depending on wether or not the value
//is an integer.
if(properLength == true) {
boolean d1 = Character.isDigit(social.charAt(social.length()-11));
boolean d2 = Character.isDigit(social.charAt(social.length()-10));
boolean d3 = Character.isDigit(social.charAt(social.length()-9));
boolean d4 = Character.isDigit(social.charAt(social.length()-7));
boolean d5 = Character.isDigit(social.charAt(social.length()-6));
boolean d6 = Character.isDigit(social.charAt(social.length()-4));
boolean d7 = Character.isDigit(social.charAt(social.length()-3));
boolean d8 = Character.isDigit(social.charAt(social.length()-2));
boolean d9 = Character.isDigit(social.charAt(social.length()-1));
}
// Testing if all of d1-d9 are actually digits
boolean properDigits;
if((d1 == true) && (d2 == true) && (d3 == true) && (d4 == true)
&& (d5 == true) && (d6 == true) && (d7 == true) && (d8==true)
&& (d9==true)) {
properDigits = true;
}else {
properDigits = false;
}
//If dashes are in proper place, we have the proper length, and d1-d9 are
//integers, then we have a valid input
if((properLength == true)&&(properDash==true)&&(properDigits==true)) {
System.out.println(" Valid Input ");
}else {
System.out.println(" Invalid Input ");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
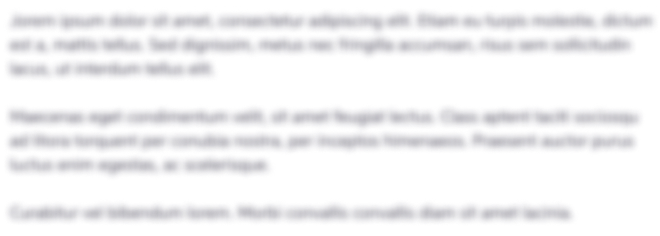
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started