Question
Write a program that prompts the user to enter two numbers. The numbers will always be >= 0 and
Write a program that prompts the user to enter two numbers. The numbers will always be >= 0 and <= 999. You can assume that the user will always supply you with an integer within this range and do not need to "test" this number to ensure that it falls within the range.
Next, you will be performing a series of computations that will require you to isolate specific digits in each number based on their place value. You will also be computing a "super number" which is the result of a series of additional calculations using these values. The sample output below shows how this program will work (user input is highlighted in yellow, though you do not need to replicate the yellow highlights in your program):
Enter a 3 digit number between 000 and 999: 7 Enter a 3 digit number between 000 and 999: 908 Digits in the 1's places: 7 and 8 Digits in the 10's places: 0 and 0 Digits in the 100's places: 0 and 9 Graphical representation of your numbers Hundreds Tens Ones 7777777 999999999 88888888 Computing Your Super Number! Step #1: Add Each Place Value - Hundreds: 0 + 9 = 9 - Tens: 0 + 0 = 0 - Ones: 7 + 8 = 15 Step #2: Combine New Values - 9 + 0 + 15 = 9015 Step #3: Compute The Sum of All Digits in First Number - 0 + 0 + 7 = 7 Step #4: Compute The Sum of All Digits in Second Number - 9 + 0 + 8 = 17 Step #5: Combine The Numbers In This Order -- Step 3 + Step 2 + Step 4 - 7901517
Your program should begin by asking the user for two numbers. The program should then isolate the values that exist at each place value (One's place, Ten's place and Hundred's place) and print them out in the format provided.
The program should then move on and generate a graphical chart showing the numbers in each place value in such a way that they are "repeated" the correct number of times. For example, the number 921 would be printed "999999999 22 1" (9 nine's, 2 two's and 1 one). Ensure that your program formats the values such that they line up nicely into three columns.
Next, you will compute the user's "super number" using the following algorithm:
Step 1: Compute the sum of each place value (i.e. hundreds1 + hundreds2, tens1 + tens2, ones1 + ones2)
Step 2: Combine these values together (i.e. hundreds_sum + tens_sum + ones_sum) - note that this step asks you to "combine" the numbers, not "add" them together. Hint: concatenation may be helpful here!
Step 3: Compute the sum of all digits in the first number
Step 4: Compute the sum of all digits in the second number
Step 5: Combine the results into a final value (Step3 combined with Step 2 combined with Step 4, in that order) - note that this is not a sum, but a combination of the numbers in a particular order.
Hint: Think about how you isolate the "ones" digit - is there a math operation that you can use to extract this information? Huge hint: what happens when you divide the number by 10? Does that give you any usable information? How about the remainder of this division operation? Once you isolate the "ones" digit you then need to move on to the "tens" digit. One way to do this would be to "throw away" the ones digit so you can focus your attention on the "tens" digit. Is there a way you can do this using a math operation?
You may not use any advanced techniques that we have not covered to solve this problem (i.e. if you've read ahead and you have learned about string or list indexing / slicing you may not use these to solve this problem). You may, however, need to use the str and int functions to convert between integers and strings. In short, any of the following techniques are allowed:
Math operations (+, -, *, /, //, %)
The print function
The format function
The str, int or float functions
Your program should be formatted exactly as the one above.
Here are some additional examples of the program:
Enter a 3 digit number between 000 and 999: 123 Enter a 3 digit number between 000 and 999: 456 Digits in the 1's places: 3 and 6 Digits in the 10's places: 2 and 5 Digits in the 100's places: 1 and 4 Graphical representation of your numbers Hundreds Tens Ones 1 22 333 4444 55555 666666 Computing Your Super Number! Step #1: Add Each Place Value - Hundreds: 1 + 4 = 5 - Tens: 2 + 5 = 7 - Ones: 3 + 6 = 9 Step #2: Combine New Values - 5 + 7 + 9 = 579 Step #3: Compute The Sum of All Digits in First Number - 1 + 2 + 3 = 6 Step #4: Compute The Sum of All Digits in Second Number - 4 + 5 + 6 = 15 Step #5: Combine The Numbers In This Order -- Step 3 + Step 2 + Step 4 - 657915
Enter a 3 digit number between 000 and 999: 999 Enter a 3 digit number between 000 and 999: 0 Digits in the 1's places: 9 and 0 Digits in the 10's places: 9 and 0 Digits in the 100's places: 9 and 0 Graphical representation of your numbers Hundreds Tens Ones 999999999 999999999 999999999 Computing Your Super Number! Step #1: Add Each Place Value - Hundreds: 9 + 0 = 9 - Tens: 9 + 0 = 9 - Ones: 9 + 0 = 9 Step #2: Combine New Values - 9 + 9 + 9 = 999 Step #3: Compute The Sum of All Digits in First Number - 9 + 9 + 9 = 27 Step #4: Compute The Sum of All Digits in Second Number - 0 + 0 + 0 = 0 Step #5: Combine The Numbers In This Order -- Step 3 + Step 2 + Step 4 - 279990
Enter a 3 digit number between 000 and 999: 1 Enter a 3 digit number between 000 and 999: 23 Digits in the 1's places: 1 and 3 Digits in the 10's places: 0 and 2 Digits in the 100's places: 0 and 0 Graphical representation of your numbers Hundreds Tens Ones 1 22 333 Computing Your Super Number! Step #1: Add Each Place Value - Hundreds: 0 + 0 = 0 - Tens: 0 + 2 = 2 - Ones: 1 + 3 = 4 Step #2: Combine New Values - 0 + 2 + 4 = 024 Step #3: Compute The Sum of All Digits in First Number - 0 + 0 + 1 = 1 Step #4: Compute The Sum of All Digits in Second Number - 0 + 2 + 3 = 5 Step #5: Combine The Numbers In This Order -- Step 3 + Step 2 + Step 4 - 10245
Enter a 3 digit number between 000 and 999: 33 Enter a 3 digit number between 000 and 999: 983 Digits in the 1's places: 3 and 3 Digits in the 10's places: 3 and 8 Digits in the 100's places: 0 and 9 Graphical representation of your numbers Hundreds Tens Ones 333 333 999999999 88888888 333 Computing Your Super Number! Step #1: Add Each Place Value - Hundreds: 0 + 9 = 9 - Tens: 3 + 8 = 11 - Ones: 3 + 3 = 6 Step #2: Combine New Values - 9 + 11 + 6 = 9116 Step #3: Compute The Sum of All Digits in First Number - 0 + 3 + 3 = 6 Step #4: Compute The Sum of All Digits in Second Number - 9 + 8 + 3 = 20 Step #5: Combine The Numbers In This Order -- Step 3 + Step 2 + Step 4 - 6911620
Step by Step Solution
There are 3 Steps involved in it
Step: 1
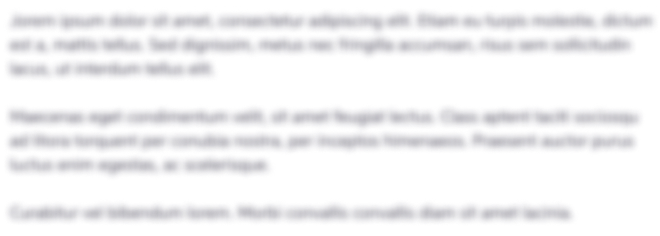
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started