Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a program that reads a graph from the console and determines whether the graph is connected. The first line in the input contains a
Write a program that reads a graph from the console and determines whether the graph is connected. The
first line in the input contains a number that indicates the number of vertices n The vertices are labeled as
n Each subsequent line, with the format u v v describes edges
u vu v and so on Figure gives the examples of two input for their corresponding
graphs.
Your program should prompt the user to enter the input, create an instance g of Graph, invoke
gprintEdges to display all edges, and invokes dfs to obtain an instance tree of Tree.
If tree.getNumberOfVerticeFound is the same as the number of vertices in the graph, the
graph is connected.
When
reading a line of integers, read it as a string using getline and then split it and convert to int. You can
use the stringstream to split a string separated by whitespace characters
Enter the number of vertices:
The number of vertices is
Enter the edges in lines:
:
:
:
:
:
:
The graph is connected
Enter the number of vertices:
The number of vertices is
Enter the edges:
:
:
:
:
:
:
The graph is not connected
Search for "WRITE YOUR CODE" to complete this program
#ifndef GRAPHH
#define GRAPHH
#include
#include For implementing BFS
#include
#include For converting a number to a string
#include
#include
using namespace std;
#ifndef TREEH
#define TREEH
class Tree
public:
Construct an empty tree
Tree
Construct a tree with root, parent, and searchOrder
Treeint root, const vector& parent,
const vector& searchOrders
thisroot root;
thisparent parent;
thissearchOrders searchOrders;
Return the root of the tree
int getRoot const
return root;
Return the parent of vertex v
int getParentint v const
return parentv;
Return search order
vector getSearchOrders const
return searchOrders;
Return number of vertices found
int getNumberOfVerticesFound const
return searchOrders.size;
Return the path of vertices from v to the root in a vector
vector getPathint v const
vector path;
do
path.pushbackv;
v parentv;
while v ;
return path;
Print the whole tree
void printTree const
cout "Root is: root endl;
cout "Edges: ;
for unsigned i ; i searchOrders.size; i
if parentsearchOrdersi
Display an edge
cout parentsearchOrdersi
searchOrdersi;
cout endl;
private:
int root; The root of the tree
vector parent; Store the parent of each vertex
vector searchOrders; Store the search order
;
#endif
#ifndef EDGEH
#define EDGEH
class Edge
public:
int u;
int v;
Edgeint u int v
thisu u;
thisv v;
;
#endif
template
class Graph
public:
Construct an empty graph
Graph;
Construct a graph from vertices in a vector and
edges in D array
Graphconst vector& vertices, const int edges int numberOfEdges;
Construct a graph with vertices n and
edges in D array
Graphint numberOfVertices, const int edges int numberOfEdges;
Construct a graph from vertices and edges objects
Graphconst vector& vertices, const vector& edges;
Construct a graph with vertices n and
edges in a vector
Graphint numberOfVertices, const vector& edges;
Return the number of vertices in the graph
int getSize const;
Return the degree for a specified vertex
int getDegreeint v const;
Return the vertex for the specified index
V getVertexint index const;
Return the index for the specified vertex
int getIndexV v const;
Return the vertices in the graph
vector getVertices const;
Return the neighbors of vertex v
vector getNeighborsint v const;
Print the edges
void printEdges const;
Print the adjacency matrix
void printAdjacencyMatrix const;
Clear the graph
void clear;
Adds a vertex to the graph
virtual bool addVertexconst V& v;
Adds an edge
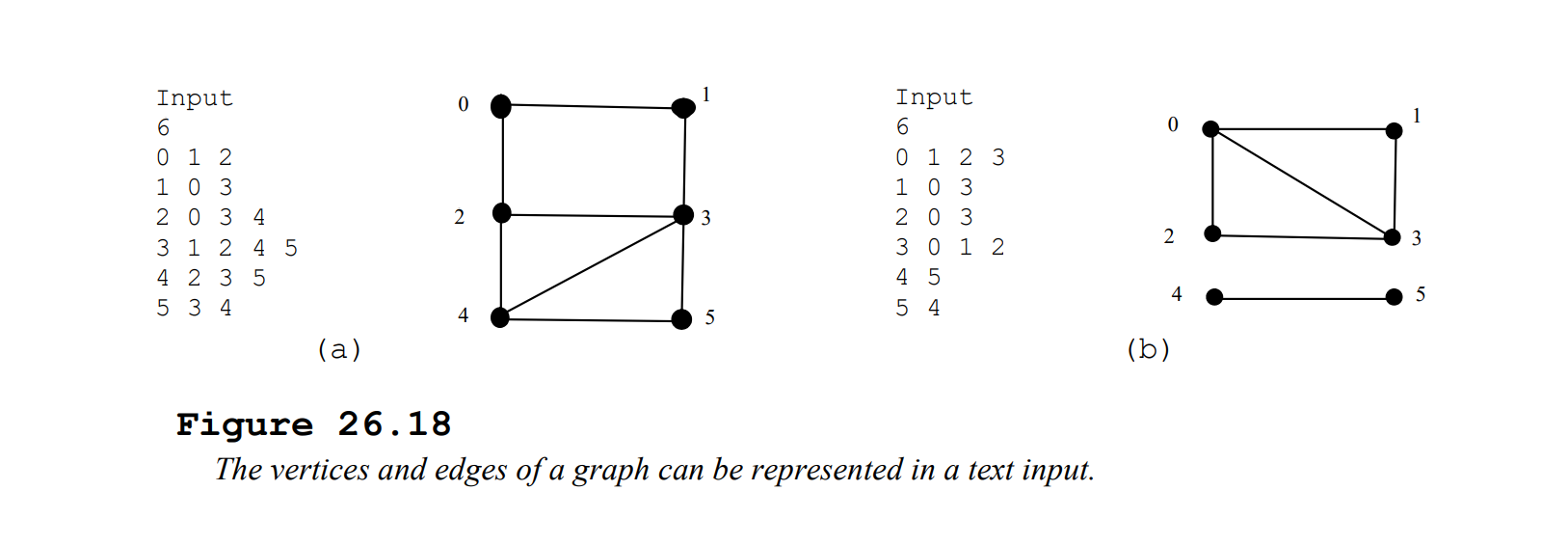
Step by Step Solution
There are 3 Steps involved in it
Step: 1
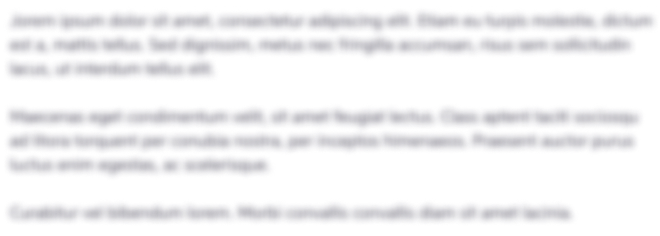
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started