Question
Write a program that simulates an image viewer application. The program should allow typical viewer functionalities such as viewing an image, its previous image, its
Write a program that simulates an image viewer application. The program should allow typical viewer functionalities such as viewing an image, its previous image, its next image, slideshow, compress image, delete image... To do that, the program creates a doubly linked list of Images sorted by their names (ascending order of image names).
Each Image has two pointers to an Image, previous and next, and other private attributes as summarized below:
string name; // includes path
double size; // in Kbytes
string format; // jpg, bmp, png
string resolution; // 1024x1024, 640x480, 3376x6000
Image * previous; // pointer to previous Image node
Image * next; // pointer to next Image node
The class ImageViewer has the following private attributes:
Image * head; // pointer to list head
Image * current; // pointer to current node being viewed. It is initialized to NULL // and is updated as new images are viewed/deleted
ImageViewer has at least one constructor, one destructor, and the following member functions:
? void addImage(string iName, double iSize, string iFormat, string iResolution)
This function creates a new Image and initializes its data to iName, iSize, iFormat, and iResolution. The function then inserts the new Image into the list while maintaining the list order.
? void viewImage(string iName)
This function displays the details of the image whose name matches iName. The function should update the current pointer to point to this Image. If no such Image exists, an error message must be displayed.
? void viewNextImage()
This function updates the current pointer to point to the next image in the list, if there is any, and then displays its details. If the current image is the last element in the list, the current pointer should not be updated and a message should be displayed that this is the last image.
? void viewPreviousImage()
This function updates the current pointer to point to the previous image in the list, if there is any, and then displays its details. If the current image is the first element in the list, the current pointer should not be updated and a message should be displayed that no previous image exists.
? void deleteCurrentImage()
This function deletes the current image. It also updates the current pointer to point to either its previous image, next image if there is no previous image, or NULL if the list becomes empty.
? void compressCurrentImage (double ratio)
This function compresses the current image. Its new size becomes the product of its old size and the compression ratio.
? void slideshow()
This function traverses the list and prints the details of all images in order.
? void reverseSlideshow()
This function traverses the list and prints the details of all images in reverse order (backwards).
? void processTransactionFile()
This function reads a set of commands from the transaction file and executes the corresponding member functions.
After designing your Image and ImageViewer classes, you need to write the following in your main program:
int main()
{
ImageViewer viewer;
viewer.processTransactionFile( );
return 0;
}
A sample transaction file is shown below. You may use it to test your code: addImage C:/Users/Mayssaa/CS211/HW4/roadtrip 3840 jpg 3376x6000 addImage C:/Users/Mayssaa/CS211/HW4/graduation 4370 jpg 60003376 addImage C:/Users/Mayssaa/CS211/HW4/family 3330 jpg 24004240 addImage C:/Users/Mayssaa/CS211/HW4/party 373 jpg 9601280 addImage C:/Users/Mayssaa/CS211/HW4/car 1770 jpg 24483264 addImage C:/Users/Mayssaa/CS211/HW4/beach 302 jpg 20481365 addImage C:/Users/Mayssaa/CS211/HW4/mountains 243 png 1280798 addImage C:/Users/Mayssaa/CS211/HW4/baby 960 png 1024746 addImage C:/Users/Mayssaa/CS211/HW4/selfie 257 bmp 512512 addImage C:/Users/Mayssaa/CS211/HW4/faces 80.7 jpeg 717558 addImage C:/Users/Mayssaa/CS211/HW4/random 299 jpeg 537400 addImage C:/Users/Mayssaa/CS211/HW4/summer 257 bmp 512512 slideshow viewImage car viewNextImage viewPreviousImage viewPreviousImage compressCurrentImage 0.8 viewPreviousImage deleteCurrentImage viewPreviousImage viewNextImage viewNextImage viewNextImage viewNextImage |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
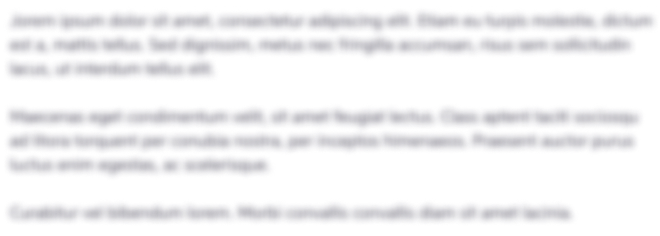
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started