Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a program that solves Sudoku puzzles. The input to Sudoku is a 9 x 9 board that is subdivided into 3 x 3 squares.
Write a program that solves Sudoku puzzles. The input to Sudoku is a x board that is subdivided
into x squares. Each cell is either blank or contains an integer from to
A solution to a puzzle is the same board with every blank cell filled in with a digit from to such
that every digit appears exactly once in every row, column, and square.
The input to the program is a text file containing a collection of Sudoku boards, with one board
per line. For example:
Z
For each board that is read, the output is a printout of the board correctly filled in
Part a
Some of the declarations and definitions for the board class are given to you. Optional
You can choose to write your own codes. Add functions to the class that:
initialize the board, and update conflicts,
print the board and the conflicts to the screen,
add a value to a cell, and update conflicts,
clear a cell, and update conflicts, and
check to see if the board has been solved return true or false, and print the result to
the screen
For each row i and digit j keep track of whether each digit j has been placed in row i Do
the same for each column and each square. We will use this information in part b of the
project to write the Sudoku solver.
The code you submit should read each Sudoku board from the file onebyone, print the
board and conflicts to the screen, and check to see if the board has been solved all boards
will not be solved at this point
board.cpp:
#include
#include
#include dmatrix.h
#include dexcept.h
#include
#include
using namespace std;
typedef int ValueType; The type of the value in a cell
const int Blank ; Indicates that a cell is blank
const int SquareSize ; The number of cells in a small square
usually The board has
SquareSize rows and SquareSize
columns.
const int BoardSize SquareSize SquareSize;
const int MinValue ;
const int MaxValue ;
int numSolutions ;
class board
Stores the entire Sudoku board
public:
boardint;
void clear;
void initializeifstream &fin;
void print;
bool isBlankint int;
ValueType getCellint int;
private:
The following matrices go from to BoardSize in each
dimension, ie they are each BoardSizeBoardSize
matrix value;
;
board::boardint sqSize
: valueBoardSizeBoardSize
Board constructor
clear;
void board::clear
Mark all possible values as legal for each board entry
for int i ; i BoardSize; i
for int j ; j BoardSize; j
valueij Blank;
void board::initializeifstream &fin
Read a Sudoku board from the input file.
char ch;
clear;
for int i ; i BoardSize; i
for int j ; j BoardSize; j
fin ch;
If the read char is not Blank
if ch
setCellijch; Convert char to int
int squareNumberint i int j
Return the square number of cell ij counting from left to right,
top to bottom. Note that i and j each go from to BoardSize
Note that int iSquareSize and int jSquareSize are the xy
coordinates of the square that ij is in
return SquareSize iSquareSizejSquareSize ;
ostream &operatorostream &ostr, vector &v
Overloaded output operator for vector class.
for int i ; i vsize; i
ostr vi;
cout endl;
ValueType board::getCellint i int j
Returns the value stored in a cell. Throws an exception
if bad values are passed.
if i && i BoardSize && j && j BoardSize
return valueij;
else
throw rangeErrorbad value in getCell";
bool board::isBlankint i int j
Returns true if cell ij is blank, and false otherwise.
if i i BoardSize j j BoardSize
throw rangeErrorbad value in setCell";
return getCellij Blank;
void board::print
Prints the current board.
for int i ; i BoardSize; i
if i SquareSize
cout ;
for int j ; j BoardSize; j
cout ;
cout ;
cout endl;
for int j ; j BoardSize; j
if j SquareSize
cout ;
if isBlankij
cout getCellij;
else
cout ;
cout ;
cout endl;
cout ;
for int j ; j BoardSize; j
cout ;
cout ;
cout endl;
int main
ifstream fin;
Read the sample grid from the file.
string fileName "sudoku.txt;
fin.openfileNamecstr;
if fin
cerr "Cannot open fileName endl;
exit;
try
board bSquareSize;
while fin && fin.peekZ
binitializefin;
bprint;
bprintConflicts;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
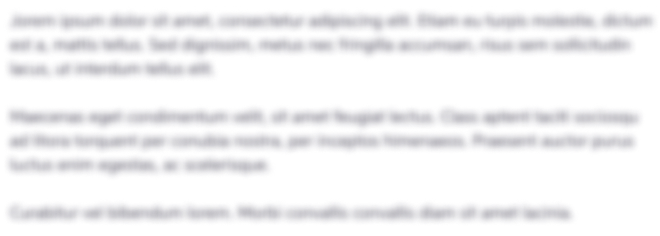
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started