Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write a program that takes one number from the OUSB Board DIP switches and a second number from the command line arguments as input numbers
Write a program that takes one number from the OUSB Board DIP switches and a second number from the command line arguments as input numbers to your program. The program will then perform a specific type of arithmetic operation, which will be determined by the value of the second command line argument (if it is provided). The resulting answer will then be displayed to the console screen in signed decimal representation. Additionally your program will be required to also represent the absolute value of the result as an 8-bit unsigned integer on the OUSB IO Board LEDs. Therefore if the arithmetic operation on the two input numbers results in a decimal result, the signed decimal value shall be displayed on the console screen and the absolute 8-bit integer representation (ie. truncated version) will be represented on the OUSB board LEDs. You will need to account for the limitations in the hardware and appropriately write the correct value to the OUSB board. The type of arithmetic operation that will be performed on the two input numbers will be determined by the second command line argument (if provided), where if the second argument is: 'a' an addition is to be performed, 's' a subtraction is to be performed, 'm' a multiplication is to be performed, 'd' a division is to be performed, and 'p' an exponential operation is to be performed. If only one argument is provided, then the two input numbers should added together. The functionality of this lab is relatively simple: + - / * ^ The emphasis in this lab is to achieve the basic FUNCTIONALITY REQUIREMENTS, however your program will also need to consider range checking, as well as basic, intermediate and advanced error level detection and handling to achieve full marks. You should aim for functionality first, and if time permits you should then attempt the error checking and implementing the basic, then intermediate and then the advanced error handling in priority order. ___________________________________________________________________________________________ 1. All outputs are a number or single character error indicator followed by a linefeed ( endl or ). 2. DO NOT use 'cin' or gets() type functions. Do NOT ask for user input. All input MUST be specified on the command line separated by blank spaces (i.e. use the argv and argc input parameters). 3. All input and output is case sensitive. 4. When your code exits the 'main()' function using the 'return' command, you MUST use zero as the return value. This requirement is for exiting the 'main()' function ONLY. 5. Do NOT put any functions after the 'main()' function - if you write your own functions, add them BEFORE the 'main()' function. This is a requirement of the Autotester and failure to do so will result in the Autotester not compiling your code correctly and loss of marks, even though the code will compile during the lab test session. 6.You MUST run this file as part of a Project - No other cpp files should be added to your solution. The OUSB.exe file will be provide as part of the project. 7. The OUSB Board switch state is always used as the first numerical argument. 8. The user-defined functions to read and write to the OUSB board are provided before the 'main()' function. Do not change the location of these functions (ReadPinC() an WritePortB()) as all user defined functions must be placed before the main() function in this test. 9. If an error (as per the definition in this specification) occurs then the value on the OUSB LEDs must not to be updated. 10. All numerical values read from / written to the OUSB Board are considered to be unsigned and are between 0 and 255 (inclusive). 11. All binary numbers within this document have the prefix 0b. This notation is not C++ compliant, however is present to avoid confusion between decimal, hexadecimal and binary number formats with in the description and specification provided in this document. For example number 10 in decimal could be written as 0xA in hexadecimal and 0b1010 in binary. The could equally be written with leading zeroes such as: 0x0A or 0b00001010 ___________________________________________________________________________________________ ___ FUNCTIONAL REQUIREMENTS: F1. When no commnd line arguments display name F2. For the situation where ONE command line argument is passed to your program: F2.1 Your program must perform an addition operation, taking the first number from the DIP switch state on the OUSB board and the command line argument as the second operand for the arithmetic calculation. The signed result should be displayed to the console with a new line character and the equivalent unsigned 8-bit absolute integer represenation to the OUSB LEDs. F3. For the situation where TWO command line arguments are passed to your program: F3.1 If the second argument is 'a', your program must perform an addition operation, taking the first number from the DIP switch state on the OUSB board and the command line argument as the second operand. The signed result should be displayed to the console with a new line character and the equivalent unsigned 8-bit absolute integer represenation to the OUSB LEDs. F3.2 If the second argument is 's', your program must perform a subtraction operation, taking the first number from the DIP switch state on the OUSB board and the command line argument as the second operand. The signed result should be displayed to the console with a new line character and the equivalent unsigned 8-bit absolute integer represenation to the OUSB LEDs. F3.3 If the second argument is 'm', your program must perform a multiplication operation, taking the first number from the DIP switch state on the OUSB board and the command line argument as the second operand. The signed result should be displayed to the console with a new line character and the equivalent unsigned 8-bit absolute integer represenation to the OUSB LEDs. F3.4 If the third argument is 'd', your program must perform a division operation, taking numerator from the DIP switch state on the OUSB board and the command line argument as the denominator. The signed result should be displayed to the console with a new line character and the equivalent unsigned 8-bit absolute integer represenation to the OUSB LEDs. F3.5 If the third argument is 'p', your program must perform an exponential operation, taking the base operator from the DIP switch state on the OUSB board and the command line argument as the exponent. The signed result should be displayed to the console with a new line character and the equivalent unsigned 8-bit absolute integer represenation to the OUSB LEDs. Hint: Consider using the pow() function, which has the definition: double pow(double base, double exponent); Example 1: lab2_1234567.exe -5 d Given an OUSB Switch value of 35 (0b00100011): which should return 35 / -5 = -7, i.e. the last (and only) line on the console will be: -7 The absolute value of -7, which is 7 should be written to the OUSB LEDs (0b00000111). Example 2: lab2_1234567.exe -10 d Given an OUSB Switch value of 41 (0b00101001): which should return 41 / -10 = -4.1, i.e. the last (and only) line on the console will be: -4.1 The absolute value of -4.1, which is 4 should be written to the OUSB LEDs (0b00000100). Example 3: lab2_1234567.exe 2 p Given an OUSB Switch value of 10 (0b00001010): which should return 10 ^ 2 = 100, i.e. the last (and only) line on the console will be: 100 The value of 100 should be also written to the OUSB LEDs (0b01100100). F4. For situation where more than TWO command line arguments are passed to your program, your program must display the character 'P' to the console with a new line character. F5. For specifications F1 to F4 inclusive: F5.1 Program must return 0 under all situations at exit. F5.2 Program must be able to handle both integer and float arguments from the command line arguments. F5.3 Program must write only the equivalent unsigned 8-bit absolute integer represenation of the result produced by the arithmetic operation to the OUSB LEDs. F5.4 If the result to be written to the OUSB Board LEDs is above 255 (0xFF), the value 255 (or 0xFF) should be written to the board instead. Hint: Consider using a (int) casting operation to truncating the value after the decimal point. Example 4: lab2_1234567.exe 2 s Given an OUSB switch value of 10 (0b00001010): which should return 10 - 2 = 8, i.e. the last (and only) line on the console will be: 8 The value of 8 should be also written to the OUSB LEDs (0b00001000). Example 5: lab2_1234567.exe 10 Given an OUSB switch value of 2 (0b00000010): which should return 2 + 10 = 12, i.e. the last (and only) line on the console will be: 12 The value of 12 should be also written to the OUSB LEDs (0b00001100). Example 6: lab2_1234567.exe 4 a Given an OUSB Switch value of 10 (0b00001010): which should return 10 + 4 = 14, i.e. the last (and only) line on the console will be: 14 The value of 14 should be also written to the OUSB LEDs (0b00001110). Example 7: lab2_1234567.exe 93 a Given an OUSB Switch value of 230 (0b11100110): which should return 230 + 93 = 323, i.e. the last (and only) line on the console will be: 323 As the result is greater than 255, the value of 255 should be written to the OUSB LEDs (0b11111111). ___________________________________________________________________________________________ ___ ERROR HANDLING: The following text lists errors you must detect and a priority of testing. Note: order of testing is important as each test is slight more difficult than the previous test. E1. Error precedence: The order of errors can theoretically be check in any order, however if multiple errors occur during a program execution event, your program should only display one error code followed by a newline character and then exit (with a return 0). The displayed error codes to the console window should occur in this order: 'P' - Incorrect number of input command line arguments 'X' - Invalid numerical command line argument 'R' - command line argument out of range 'V' - Invalid operation selection 'M' - Division by zero 'Y' - MURPHY'S LAW (undefined error) Therefore if an invalid numerical command line argument and an invalid operation argument are passed to the program, the first error code should be displayed to the console, which in this case would be 'X'. Displaying 'V' or 'Y' would be result in a loss of marks. E2. Valid operator input: If the second command line input argument is not a valid operation selection, the output shall be 'V'. Valid operators are ONLY (case sensitive): a - addition s - subtraction m - multiplication d - division p - exponentiation i.e. to the power of: 2 ^ 3 = 8 (base exponent p) E3. Basic invalid number detection: Valid numbers are all numbers that the "average Engineering graduate" in Australia would consider valid. Therefore if the first command line argument is not a valid number, the output shall be 'X'. For example: 013 is valid +3 is valid -45 is valid .3 is valid ABC123 is not valid 1.3.4 is not valid 123abc is not valid E4. OUSB IO Board communication error: If the pipe code communicating to the OUSB.exe file reports and issue with communication to the OUSB IO Board, the output shall be 'Y'. This type of error can be forced by disconnecting the USB cable between the PC and the OUSB IO Board. Note: The OUSB.exe will give a message "Fatal Exception" if the board is not connected or is disconnected during normal operation. This message should NOT be returned by your code. The only output your code should give is the single character, 'Y' which is the same as the return character as the MURPHY'S LAW TEST below. E5. Intermediate invalid number detection: If the first command line argument is not a valid number, the output shall be 'X'. Using comma punctuated numbers and scientific formatted numbers are considered valid. For example: 92.000 is valid 3,000 is valid - NB: atof() will read this as '3' not as 3000 +1.23e+2 is valid 2E2 is valid 1,000.9 is valid e-1 is not valid .e3 is not valid E6. Advanced invalid number detection: If the first command line argument is not a valid integer the output shall be 'X'. +212+21-2 is not valid - NB: mathematical operation on a group of numbers, not ONE number 5/2 is not valid - NB: mathematical operation on a number of numbers, not ONE number HINT: consider the function atof(), which has the definition: double atof (const char* str); Checking the user input for multiple operators (i.e. + or -) is quite a difficult task. One method may involve writing a 'for' loop which steps through the input argv[] counting the number of operators. This process could also be used to count for decimal points and the like. The multiple operator check should be considered an advanced task and developed once the rest of the code is operational. E7. Input number range checking: E7.1 If the value of the first command line argument is greater than 65536 or less than -65535 the output shall be 'R'. Zero is a valid input. E7.2 All 8-bits of the OUSB Board DIP switches are valid. Therefore 0 to 255 will be valid inputs. E8. Division by zero should produce output 'M'. The LEDs on the OUSB Board should not be updated. E9. ANYTHING ELSE THAT CAN GO WRONG (MURPHY'S LAW TEST): If there are any other kinds of errors not covered here, the output shall be 'Y'. As an example, the type of error that could be considered here would be: - no OUSB Board connection, - disconnection during operation - HW dead. - anything else Murphy can dream up. - everything that has not been covered elsewhere is Murphy's domain. Rhetorical question: What for example are the error codes that the Power function returns ? If this happens then the output shall be 'Y'. E10. Errors that will not be considered (and hence no score awarded). 1,00.0 will not be tested (comma placement) 1.4E0.2 will not be tested (multiple decimal points). ___________________________________________________________________________________________
Step by Step Solution
There are 3 Steps involved in it
Step: 1
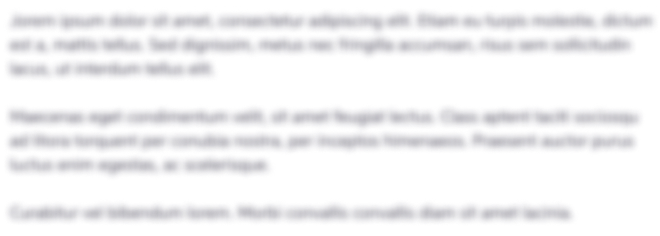
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started