Write a program that will compute and print the semester tuition bill for the Univeristy of Computers. The program will keep a running total for
Write a program that will compute and print the semester tuition bill for the Univeristy of Computers. The program will keep a running total for all students and print this as well as result for the University of Computers. Create University class that will have data and methods associated with the University. You MUST use the demo program that is given below. Please note: method called calculateData(); must call private mathods to do the calculations. An instance of the student is sent into the method collectDataForReport (person); (). The data of the student is private and you will need a getTuition() method to access it. A sample line of code in the University class would be : totalTuition = totalTuition + person.getTution();
You must use this demo
import java.util.Scanner;
public class UniversityDemo {
static Scanner scan = new Scanner(System.in);
public static void main(String[] args) {
University clerk = new University( );
Student student = new Student();// one student
int i;
System.out.println("Enter number of students:");
int numberOfStudents = scan.nextInt();
for (i = 1; i <= numberOfStudents; i++) {
System.out.println("Enter data for student number " + i);
student.readInput();
student.calculateData();
student.writeOutput( );
clerk.colectDataForUniversityReport(student);
}
clerk.printDataForSchoolReport();
}
}
/* the calculateData() method must have private methods. Examples: tuitionCalculation() lateCalculation (); healthCareCalculation(); incidentalCalculation (); readMealSelection(); computeFoodFee(); Getters and Setters for the private data is necessary. Eclipse will create them for you. Place your mouse where you would like them (Usually at the bottom of the class). Right click, choose Source, choose Generate Getters and Setters. Choose the private variables you want. (all of them). */
There are two static methods that can be used to print a string left or right justified in a space of characters.
public class OutPut { // ************************************************************************* //Blank fills and left justifies a string in a field of size characters // and returns string public static String makeStringLeft(int size, String formatted) { String answer = ""; int length = formatted.length(); answer = answer + formatted; while (size > length) { answer = answer + " "; size --; } // End while (size > length) return answer; } public static void printStringLeft(int size, String formatted) // Blank fills and left justifies a string in a field of size characters { int length = formatted.length(); System.out.print(formatted); while (size > length) { System.out.print(" "); size --; } // End while (size > length) } // End function printString // ************************************************************************* //Blank fills and right justifies a string in a field of size characters // and returns string public static String makeStringRight(int size, String formatted) // Blank fills and right justifies a string in a field of size characters { String answer = ""; int length = formatted.length(); while (size > length) { answer = answer + " "; size --; } // End while (size > length) answer = answer + formatted; return answer; } public static void printStringRight(int size, String formatted) // Blank fills and right justifies a string in a field of size characters { int length = formatted.length(); while (size > length) { System.out.print(" "); size --; } // End while (size > length) System.out.print(formatted); } // End function printString // *************************************************************************** }
ALSO
\\two ways to do monay formatting
import java.text.DecimalFormat;
import java. text.NumberFormat;
public class MoneyFormatDemo
{
public static void main (String[] args) {
NumberFormat moneyFormatter = NumberFormat,getCurrencyInstance();
System.out.println( MoneyFormatter.format (2003.4));
String money = moneyFormatter.format (20043.44);
System.out.println(money);
DecimalFormat dollarFormat = new DecimalFormat ("$#,###,###.00");
System.out.println (dollarFormat.format(34456.2));
money = dollarFormat.format(20043.44);
System.out.println(money);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
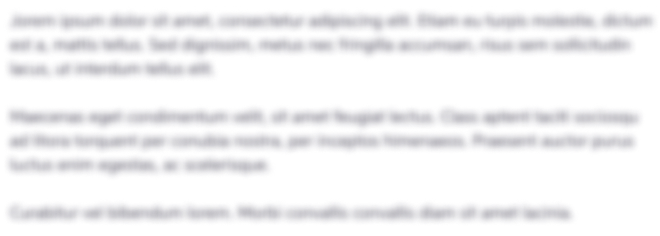
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started