Question
Write a program that will search soccer players data to check whether a name supplied by the user is on that list. For doing the
Write a program that will search soccer players data to check whether a
name supplied by the user is
on that list. For doing the search, the user may provide either the player
s full last name or one or
more starting letters of the last name. If a matching last name is
found, the program will display the
player
s full name and date of birth. Otherwise, it will display
Not found
.
Requirements specification:
At the start, the program will ask the user to enter information about 10 socc
er players constituting
soccer player data. Each soccer player data will consist of the following
fields:
Last name
First name
Birth month
Birth day
Birth year
The user will be asked to enter each soccer player data on a separate lin
e with the field values
separated by a space.
Once the data is entered, the program will display a menu of choices as
below:
Chose an option:
(1
input data, 2
display original data, 3
sort data , 4
display sorted data 5
search by last
name 6
exit the program )
If the user chooses option 1, the program will ask the user for a data and populat
e the array of
structures that will hold the data for each of the soccer players.
If the user chooses option 2, the program will display data in the order provided by the
user (original
data).
If use chooses option 3, the program will sort data by last name.
If user chooses number 4 the program will display the data sorted by last name.
If the user chooses option 5, the program will ask the user to enter one or more st
arting letters of the
soccer player
s last name. It will then search the soccer player data for the matchi
ng last name. If a
match is found, it will display the first player found with the matc
hing pattern. If a match is not
found, the program will display
Not found
. If the user enters two forward slashes (//) for the last
name, it will no longer search for the name. Instead it will displa
y the main option menu.
If the user chooses option 6, the program will display
Thank you for using this program
and will
end.
ANALYSIS
Input/Output:
(User input is in bold)
Enter data for 10 soccer players (you can use a txt file to input data if you wis
h )
(Separate the fields by spaces and enter each player data on a new line
)
Note the names of the list bellow are real soccer players but the birth
days are made up. You can
change this list as you wish.
Roberto Baggio 01 12 1992
David Beckham 05 12 1988
Pablo Aimar 05 13 1987
Michael Ballack 11 13 1999
Gabriel Batistuta 05 05 1979
Franz Beckenbauer 18 01 1976
Dennis Bergcamp 03 14 1989
Omar Bravo 03 03 1999
Jared Borgetti 09 23 1977
Fabio Cannavaro 02 25 1990
Chose an option:
(1
Input data, 2
display original data, 3
sort data by last name, 4
display sorted data 5
search
by last name 6
display goodbye message and exit the program )
1
Chose an option:
(1
Input data, 2
display original data, 3
sort data by last name, 4
display sorted data 5
search
by last name 6
display goodbye message and exit the program )
2
Unsorted data:
Roberto Baggio 01 12 1992
David Beckham 05 12 1988
Pablo Aimar 05 13 1987
Michael Ballack 11 13 1999
Gabriel Batistuta 05 05 1979
Franz Beckenbauer 18 01 1976
Dennis Bergcamp 03 14 1989
Omar Bravo 03 03 1999
Jared Borgetti 09 23 1977
Fabio Cannavaro 02 25 1990
Chose an option:
(1
Input data, 2
display original data, 3
sort data by last name, 4
display sorted data 5
search
by last name 6
display goodbye message and exit the program)
3
Chose an option:
(1
Input data, 2
display original data, 3
sort data by first name, 4
display sorted data 5
search by last name 6
display goodbye message and exit the program)
4
Sorted data:
David Beckham 05 12 1988
David Beckham 05 12 1988
Eric Cantona 03 14 1989
Fabio Cannavaro 02 25 1990
Franz Beckenbauer 18 01 1976
Gabriel Batistuta 05 05 1979
Jared Borgetti 09 23 1977
Michael Ballack 11 13 1999
Omar Bravo 03 3 1999
Pablo Aimar 05 13 1987
Roberto Baggio 01 12 1992
Chose an option:
(1
Input data, 2
display original data, 3
sort data by last name, 4
display sorted data 5
search
by last name 6
display goodbye message and exit the program)
5
// this will run in a loop
Enter one or more starting letters of the last name ( enter
//
to quit this option):
R
Roberto Baggio 01 12 1992
Enter one or more starting letters of the last name:
Mic
Michael Ballack 11 13 1999
Enter one or more starting letters of the last name:
Fabio
Fabio Cannavaro 02 25 1990
Enter one or more starting letters of the last name:
A
Not found.
Enter one or more starting letters of the last name:
Kerry
Not found.
Enter starting letter/letters from the last name:
//
Chose an option:
(1
Input data, 2
display original data, 3
sort data by last name, 4
display sorted data 5
search
by last name 6
display goodbye message and exit the program )
6
Thank you for using this program.
DESIGN of the main module ( main function )
read celebrity list
while (1)
begin
display option menu
switch (option)
begin
case 1: input data
break;
case 2:
display players list in the order entered
break
case 3:
sort by last name
break
case 4: display sorted array:
case 5: search by last name ( user will enter one or more letters fro
m the last name starting always
from the beginning of the last name)
read pattern
while (pattern !=
//
)
begin
search for the last name pattern in players list
if (found)
display players information
else
display
Not found
read pattern
end while
break
case 6:
display
Thank you for using this program
exit program
end switch
end while
IMPLEMENTATION
Bellow is the Socer player structure declaration:
struct splayer
{
char lname [20];
char fname [20];
int birthmonth;
int birthday;
int birthyear;
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
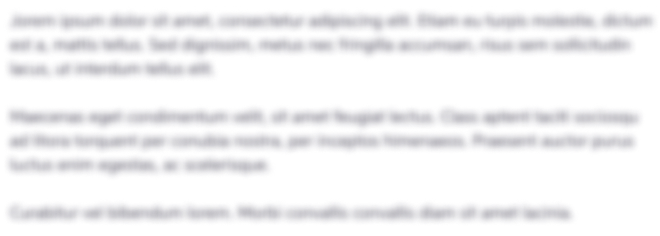
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started