Question
Write a program to solve for the number that meets the following rules. Rule 1: A number has five different digits, none of which is
Write a program to solve for the number that meets the following rules.
Rule 1: A number has five different digits, none of which is zero.
Rule 2: The first plus the second equal the third digit;
Rule 3: The third times two, plus the second, equals the fifth;
Rule 4: The second times two equals the first;
Rule 5: The first times four equals the fourth;
Rule 6: The fourth minus the second equals the fifth.
You must have a function for each rule. The function will receive the test number as input and will return a one if the rule is met, and a zero if it is not.
code so far:
#include
int rule5(int x) {//Rule 5: The first times four equals the fourth; };
int rule6(int x) {//Rule 6: The fourth minus the second equals the fifth; }; void trial() { int trialNumber=99; while (trialNumber != 0) { printf("Enter trial number, zero to stop: "); scanf_s("%d", &trialNumber); if (trialNumber != 0) { printf("Rule One: %d ", rule1(trialNumber)); printf("Rule Two: %d ", rule2(trialNumber)); printf("Rule Three: %d ", rule3(trialNumber)); printf("Rule Four: %d ", rule4(trialNumber)); printf("Rule Five: %d ", rule5(trialNumber)); printf("Rule Six: %d ", rule6(trialNumber)); } getchar(); } }; int main() { trial(); //Comment this out when done testing, and then un-comment out below. /*for (int i = 1; i < 100000; i++) { printf("%d %d%d%d%d%d%d ", i, rule1(i),rule2(i),rule3(i),rule4(i),rule5(i),rule6(i)); if (rule1(i)&&rule2(i)&&rule3(i)&&rule4(i)&&rule5(i)&&rule6(i)) getchar(); }*/ getchar(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
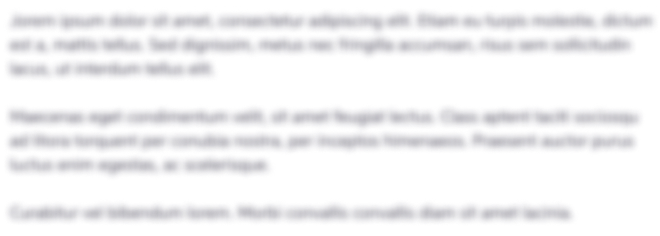
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started