Question
Write a program to solve the Tigger Problem Years of research have finally revealed the special mechanism of Tigger's bouncy step. You are to design
Write a program to solve the Tigger Problem
Years of research have finally revealed the special mechanism of Tigger's bouncy step. You are to design a Tigger class that implements this unique movement, which I will describe below.
A Tigger always starts in a random point (with coordinates x and y). When it decides to bounce a Tigger changes its x and y by the following rule(s):
x becomes the sum of the squares of its digits
y becomes the sum of the squares of its digits
Example:
if x is 37, then
one bounce turns x into 32 + 72 (= 58).
Both x and y change by this rule.
And the bounce goes on (as expected).
Here's a possible test of your Tigger class:
class One { public static void main(String[] args) { int a = (int)(Math.random() * 1000), b = (int)(Math.random() * 1000); Tigger u = new Tigger(a, b); for (int i = 0; i < 30; i++) { u.bounce(); System.out.println(u.report()); } } }
And here's the output that the previous program would produce:
Tigger just bounced to (162, 105) Tigger just bounced to ( 41, 26) Tigger just bounced to ( 17, 40) Tigger just bounced to ( 50, 16) Tigger just bounced to ( 25, 37) Tigger just bounced to ( 29, 58) Tigger just bounced to ( 85, 89) Tigger just bounced to ( 89, 145) Tigger just bounced to (145, 42) Tigger just bounced to ( 42, 20) Tigger just bounced to ( 20, 4) Tigger just bounced to ( 4, 16) Tigger just bounced to ( 16, 37) Tigger just bounced to ( 37, 58) Tigger just bounced to ( 58, 89) Tigger just bounced to ( 89, 145) Tigger just bounced to (145, 42) Tigger just bounced to ( 42, 20) Tigger just bounced to ( 20, 4) Tigger just bounced to ( 4, 16) Tigger just bounced to ( 16, 37) Tigger just bounced to ( 37, 58) Tigger just bounced to ( 58, 89) Tigger just bounced to ( 89, 145) Tigger just bounced to (145, 42) Tigger just bounced to ( 42, 20) Tigger just bounced to ( 20, 4) Tigger just bounced to ( 4, 16) Tigger just bounced to ( 16, 37) Tigger just bounced to ( 37, 58)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
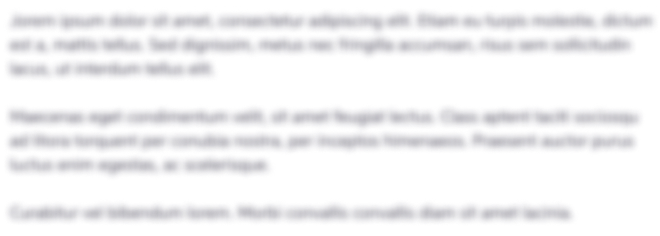
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started