Question
Write a program to test various operations of the class doublyLinkedList. Just need the main.cpp coded please do not add more to the tabs/classes c++
Write a program to test various operations of the class doublyLinkedList.
Just need the main.cpp coded
please do not add more to the tabs/classes
c++
output must match results on the bottom right.
is already written and will be after the picture.
doublyLinkedList.h
#ifndef H_doublyLinkedList
#define H_doublyLinkedList
#include
#include
using namespace std;
//Definition of the node
template
struct nodeType
{
Type info;
nodeType
nodeType
};
template
class doublyLinkedList
{
public:
const doublyLinkedList
(const doublyLinkedList
//Overload the assignment operator.
void initializeList();
//Function to initialize the list to an empty state.
//Postcondition: first = nullptr; last = nullptr; count = 0;
bool isEmptyList() const;
//Function to determine whether the list is empty.
//Postcondition: Returns true if the list is empty,
// otherwise returns false.
void destroy();
//Function to delete all the nodes from the list.
//Postcondition: first = nullptr; last = nullptr; count = 0;
void print() const;
//Function to output the info contained in each node.
void reversePrint() const;
//Function to output the info contained in each node
//in reverse order.
int length() const;
//Function to return the number of nodes in the list.
//Postcondition: The value of count is returned.
Type front() const;
//Function to return the first element of the list.
//Precondition: The list must exist and must not be empty.
//Postcondition: If the list is empty, the program
// terminates; otherwise, the first
// element of the list is returned.
Type back() const;
//Function to return the last element of the list.
//Precondition: The list must exist and must not be empty.
//Postcondition: If the list is empty, the program
// terminates; otherwise, the last
// element of the list is returned.
bool search(const Type& searchItem) const;
//Function to determine whether searchItem is in the list.
//Postcondition: Returns true if searchItem is found in
// the list, otherwise returns false.
void insert(const Type& insertItem);
//Function to insert insertItem in the list.
//Precondition: If the list is nonempty, it must be in
// order.
//Postcondition: insertItem is inserted at the proper place
// in the list, first points to the first
// node, last points to the last node of the
// new list, and count is incremented by 1.
void deleteNode(const Type& deleteItem);
//Function to delete deleteItem from the list.
//Postcondition: If found, the node containing deleteItem
// is deleted from the list; first points
// to the first node of the new list, last
// points to the last node of the new list,
// and count is decremented by 1; otherwise,
// an appropriate message is printed.
doublyLinkedList();
//default constructor
//Initializes the list to an empty state.
//Postcondition: first = nullptr; last = nullptr; count = 0;
doublyLinkedList(const doublyLinkedList
//copy constructor
~doublyLinkedList();
//destructor
//Postcondition: The list object is destroyed.
protected:
int count;
nodeType
nodeType
private:
void copyList(const doublyLinkedList
//Function to make a copy of otherList.
//Postcondition: A copy of otherList is created and
// assigned to this list.
};
template
doublyLinkedList
{
first= nullptr;
last = nullptr;
count = 0;
}
template
bool doublyLinkedList
{
return (first == nullptr);
}
template
void doublyLinkedList
{
nodeType
while (first != nullptr)
{
temp = first;
first = first->next;
delete temp;
}
last = nullptr;
count = 0;
}
template
void doublyLinkedList
{
destroy();
}
template
int doublyLinkedList
{
return count;
}
template
void doublyLinkedList
{
nodeType
current = first; //set current to point to the first node
while (current != nullptr)
{
cout info
current = current->next;
}//end while
}//end print
template
void doublyLinkedList
{
nodeType
//the list
current = last; //set current to point to the
//last node
while (current != nullptr)
{
cout info
current = current->back;
}//end while
}//end reversePrint
template
bool doublyLinkedList
search(const Type& searchItem) const
{
bool found = false;
nodeType
current = first;
while (current != nullptr && !found)
if (current->info >= searchItem)
found = true;
else
current = current->next;
if (found)
found = (current->info == searchItem); //test for
//equality
return found;
}//end search
template
Type doublyLinkedList
{
assert(first != nullptr);
return first->info;
}
template
Type doublyLinkedList
{
assert(last != nullptr);
return last->info;
}
template
void doublyLinkedList
{
nodeType
nodeType
nodeType
bool found;
newNode = new nodeType
newNode->info = insertItem; //store the new item in the node
newNode->next = nullptr;
newNode->back = nullptr;
if(first == nullptr) //if the list is empty, newNode is
//the only node
{
first = newNode;
last = newNode;
count++;
}
else
{
found = false;
current = first;
while (current != nullptr && !found) //search the list
if (current->info >= insertItem)
found = true;
else
{
trailCurrent = current;
current = current->next;
}
if (current == first) //insert newNode before first
{
first->back = newNode;
newNode->next = first;
first = newNode;
count++;
}
else
{
//insert newNode between trailCurrent and current
if (current != nullptr)
{
trailCurrent->next = newNode;
newNode->back = trailCurrent;
newNode->next = current;
current->back = newNode;
}
else
{
trailCurrent->next = newNode;
newNode->back = trailCurrent;
last = newNode;
}
count++;
}//end else
}//end else
}//end insert
template
void doublyLinkedList
{
nodeType
nodeType
bool found;
if (first == nullptr)
cout
else if (first->info == deleteItem) /ode to be deleted is
//the first node
{
current = first;
first = first->next;
if (first != nullptr)
first->back = nullptr;
else
last = nullptr;
count--;
delete current;
}
else
{
found = false;
current = first;
while (current != nullptr && !found) //search the list
if (current->info >= deleteItem)
found = true;
else
current = current->next;
if (current == nullptr)
cout
else if (current->info == deleteItem) //check for
//equality
{
trailCurrent = current->back;
trailCurrent->next = current->next;
if (current->next != nullptr)
current->next->back = trailCurrent;
if (current == last)
last = trailCurrent;
count--;
delete current;
}
else
cout
}//end else
}//end deleteNode
template
void doublyLinkedList
{
nodeType
nodeType
if(first != nullptr) //if the list is nonempty, make it empty
destroy();
if(otherList.first == nullptr) //otherList is empty
{
first = nullptr;
last = nullptr;
count = 0;
}
else
{
current = otherList.first; //current points to the
//list to be copied.
count = otherList.count;
//copy the first node
first = new nodeType
first->info = current->info; //copy the info
first->next = nullptr;
first->back = nullptr;
last = first;
current = current->next;
//copy the remaining list
while (current != nullptr)
{
newNode = new nodeType
newNode->info = current->info;
newNode->next = nullptr;
newNode->back = last;
last->next = newNode;
last = newNode;
current = current->next;
}//end while
}//end else
}//end copyList
template
doublyLinkedList
{
first = nullptr;
copyList(otherList);
}
template
const doublyLinkedList
(const doublyLinkedList
{
if (this != &otherList) //avoid self-copy
{
copyList(otherList);
}//end else
return *this;
}
template
doublyLinkedList
{
destroy();
}
#endif
Due Today at 11:59 PM HST doublyLinkedList.hmain.cpp 0/2 passed Testing the doublyLinkedList.h 1 Winclude ostream 6 Information Build Failed 3 using nanespace std: Review Mode Project Help Download Share Instructions Some test are not run unless your program builds successfully Write a program to test various operations of the class doublyLinkedL1st S int matn) 6 IINrite your main here 7 return ? Build Output Theme Light nain.cpp :3 : 9: errar: #include expects "FILENAME" or -FILENAME nain.cpp: In Tunction int nain()*: natn.cpp:16:18: error: missing tenplate argunents before List Grading As you complete the steps above, you can use the Test button to check if the lab tests are passing. Once you are satisfied with the results, use the Grade button to save your score. Keymap Default doublylinkedl1st list ADanger Area nain.cpp:29:1: errar: ist was not declared in this scope Reset Project List.inser t (58); Test Case FAILED: Input / output test 1 INPUT 11 56 89 84 1 23 32 -999 23 32 OUTPUT RESULTS List in ascanding order: 1 11 23 32 56 84 89 List in descending order: 89 84 56 32 23 11 1 List after deleting 231 11 32 36 34 8s 2 found in the list Show Details
Step by Step Solution
There are 3 Steps involved in it
Step: 1
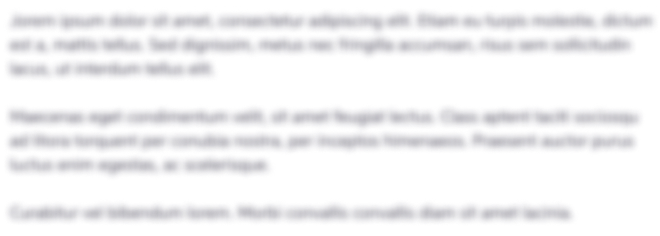
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started