Question
Write a program using a queue (array_queue.py below) class and stack (array_stack.py below) that contains a function isPalindrome(phrase) to validate whether an input phrase is
Write a program using a queue (array_queue.py below) class and stack (array_stack.py below) that contains a function isPalindrome(phrase) to validate whether an input phrase is a palindrome.
Array_queue.py
from exceptions import Empty
class ArrayQueue:
"""FIFO queue implementation using a Python list as underlying storage."""
DEFAULT_CAPACITY = 10 # moderate capacity for all new queues
def __init__(self):
"""Create an empty queue."""
self._data = [None] * ArrayQueue.DEFAULT_CAPACITY
self._size = 0
self._front = 0
def __len__(self):
"""Return the number of elements in the queue."""
return self._size
def is_empty(self):
"""Return True if the queue is empty."""
return self._size == 0
def first(self):
"""Return (but do not remove) the element at the front of the queue.
Raise Empty exception if the queue is empty.
"""
if self.is_empty():
raise Empty('Queue is empty')
return self._data[self._front]
def dequeue(self):
"""Remove and return the first element of the queue (i.e., FIFO).
Raise Empty exception if the queue is empty.
"""
if self.is_empty():
raise Empty('Queue is empty')
answer = self._data[self._front]
self._data[self._front] = None # help garbage collection
self._front = (self._front + 1) % len(self._data)
self._size -= 1
return answer
def enqueue(self, e):
"""Add an element to the back of queue."""
if self._size == len(self._data):
self._resize(2 * len(self.data)) # double the array size
avail = (self._front + self._size) % len(self._data)
self._data[avail] = e
self._size += 1
def _resize(self, cap): # we assume cap >= len(self)
"""Resize to a new list of capacity >= len(self)."""
old = self._data # keep track of existing list
self._data = [None] * cap # allocate list with new capacity
walk = self._front
for k in range(self._size): # only consider existing elements
self._data[k] = old[walk] # intentionally shift indices
walk = (1 + walk) % len(old) # use old size as modulus
self._front = 0 # front has been realigned
array_stack.py
class ArrayStack:
"""LIFO Stack implementation using a Python list as underlying storage."""
def __init__(self):
"""Create an empty stack."""
self._data = [] # nonpublic list instance
def __len__(self):
"""Return the number of elements in the stack."""
return len(self._data)
def is_empty(self):
"""Return True if the stack is empty."""
return len(self._data) == 0
def push(self, e):
"""Add element e to the top of the stack."""
self._data.append(e) # new item stored at end of list
def top(self):
"""Return (but do not remove) the element at the top of the stack.
Raise Empty exception if the stack is empty.
"""
if self.is_empty():
#raise Empty('Stack is empty')
print('Stack is empty')
return self._data[-1] # the last item in the list
def pop(self):
"""Remove and return the element from the top of the stack (i.e., LIFO).
Raise Empty exception if the stack is empty.
"""
if self.is_empty():
#raise Empty('Stack is empty')
print('Stack is empty')
return self._data.pop() # remove last item from list
def transfer(self,T):
while not self.is_empty():
temp = self.pop()
T.push(temp)
def main():
S = ArrayStack()
print(S.push(1))
print(S.top())
print(S.push(2))
print(S.top())
S.push(3)
S.push(4)
print(S.is_empty())
T = ArrayStack()
print(T.is_empty())
S.transfer(T)
print(T.is_empty())
print str(S)
main()
python
include docstring
comment code
typed please and show run screen
Step by Step Solution
There are 3 Steps involved in it
Step: 1
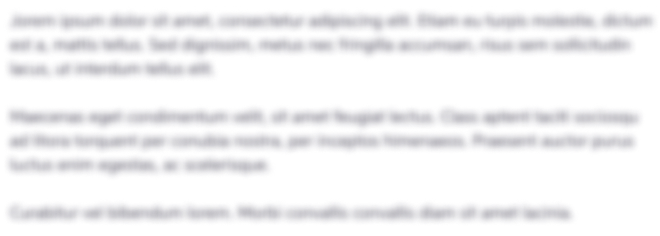
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started