Question
Write a program using references to create a doubly linked list, do not use ArrayLists, not an array, not anything but a LinkedList class that
Write a program using references to create a doubly linked list, do not use ArrayLists, not an array, not anything but a LinkedList class that has a reference to the first node in the list only. Not a circular linked list......just a double linked list.
You will only need one reference to the whole list, "first", In order to do this you will need to add new Nodes to the front of the list. So no reference to the last node, not allowed to solve the problem.
Fill the linked list with Nodes that will hold 10 names (just 10 random Strings, hardcode them in using an array to save time, then read them one by one out of the array and create a Node with the string. The array of strings should only be used as input, the array is just to save time so you don't have to type the name in every time you run it. Call a method called createList and set up the doubly linked list in there using the local array variable of names......don't leave that method until all ten names have been put into Nodes and the Nodes are added to the list.........the array of Strings (names) should be local, not an instance field.
After you have created the doubly linked list of 10 items. You should create a print method that will print the names in order.
Next your program will create a second list that is a copy of the first list but in reverse order. Create a method that takes the first list and creates a second list that is the has the exact same data, just in reverse order. Call the method and stay in the method until the complete copy has been created.
You will only have one reference to the beginning of the second linked list, call it listBFirst. No reference to the last Node in either list. Not circular lists.
You should be able to use the same print method you used above to now print out this list. You will just need to send in the reference to which list you want to print.
Node class will have three private instance variables (name, next, previous) NO OTHER INSTANCE FIELDS SHOULD BE USED
The LinkedList class will have two private instance variables (first and listBFirst) NO OTHER INSTANCE FIELDS SHOULD BE USED
The array of names can be a local variable in the createList method, not an instance field.
The public static main can be in the LinkedList class and just call the methods needed to create, print and copy and print, print again........but the public static main will not have access to the instance field first and listBFirst......do not make them static, but figure out a way to call one print method and be able to print which ever list you want to print.
I have successfully been able to create and print the list, but I do not know how to write the code to reverse it.
Here are my methods in my LinkedList class:
public void add(Node item) { if(first == null) { first = item; } else { item.next = first; first.previous = item; first = first.previous; } } public void createList() { String[] names = new String []{"bob", "jane", "pat", "gene", "alex", "chris", "kim", "hank", "joe", "greg"}; for(int i = 0; i < names.length; i++) { add(new Node(names[i])); } }
public void print() { Node iter = first; while(iter != null) { System.out.println(iter.getName()); iter.getName(); iter = iter.getNext(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
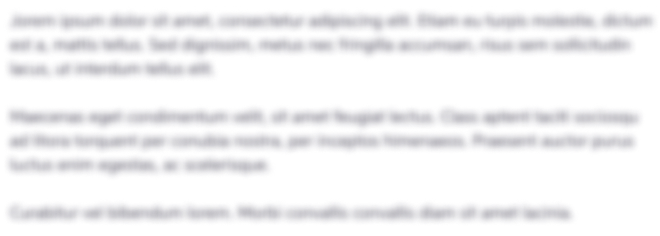
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started