Question
Write a public static void method drawMainDiagonal() to print a square picture with a main diagonal of 'X's as follows. (Side note: a diagonal of
Write a public static void method drawMainDiagonal() to print a square picture with a main diagonal of 'X's as follows.
(Side note: a diagonal of a matrix is made of the elements that go from top left to bottom right. The main diagonal starts at the first row & column position at top left. Super-diagonals lie above (or to the right) of the main diagonal. Sub-diagonals lie below or to the left.)
Your method takes a single integer parameter (n), which is the number of rows and columns of the square.
Your code will print the characters 'X' and '.' in each row as follows, putting an X on the main diagonal and padding with periods to fill out the square on both sides. Remember to println() at the end of each row.
For example, with n=5 your method would print:
X....
.X...
..X..
...X.
....X
The main ideas of this program are:
1. write a method. you will need to do this 100's of times in CS, so you need to be confident in doing it quickly.
2. use the input parameter from the method.
3. loop over the rows of the matrix, doing something
4. loop over the columns of a row, doing something
5. figure out what to do at each row & column.
public class Main { // TODO - write your method code here. // Do not modify this method. public static void main(String[] args) { drawMainDiagonal(5); drawMainDiagonal(7); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
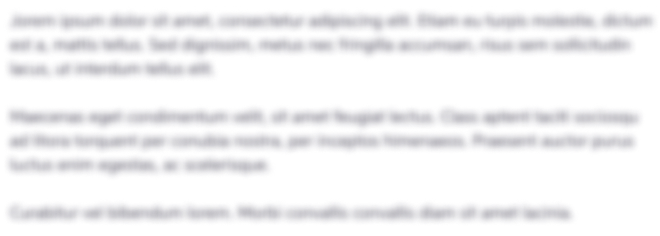
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started