Question
Write a Python class, WolfGoatCabbage , that describes the Wolf, goat and cabbage problem (same problem from HW #2) and can then be used to
Write a Python class, WolfGoatCabbage, that describes the Wolf, goat and cabbage problem (same problem from HW #2) and can then be used to solve it by calling a search algorithm.
The class must extend class Problem in the search.py code.
Represent the state by a set of characters, representing the objects on the left bank. Use the characters: F, G, W, C. Note that it is sufficient to represent the objects on one bank since the remaining will be on the other bank. E.g., {F, G} represents Farmer and Goat on the left bank and Wolf and Cabbage on the right.
An action in this puzzle is 1-2 objects crossing in the boat. Represent an action as a set of characters representing the objects crossing. E.g., {F, G} represents the farmer and goat crossing. Note that it is not necessary to represent the direction of the boat as this will be clear from the state (e.g., if the farmer is on the left, then the boat will have to cross to the right).
The class should be usable in the main function below to print the sequence of actions to reach the goal state.
from search import *
# YOUR CODE GOES HERE
if __name__ == '__main__':
wgc = WolfGoatCabbage()
solution = depth_first_graph_search(wgc).solution()
print(solution)
solution = breadth_first_graph_search(wgc).solution()
print(solution)
Your code should print something like:
[{'G', 'F'}, {'F'}, {'C', 'F'}, {'G', 'F'}, {'W', 'F'}, {'F'}, {'G', 'F'}]
[{'G', 'F'}, {'F'}, {'W', 'F'}, {'G', 'F'}, {'C', 'F'}, {'F'}, {'G', 'F'}]
Hints:
The class will be similar to class EightPuzzle in search.py. However, this is a different type of puzzle, so the code logic will be very different, just the structure remains the same. Specifically, your class should have a
a constructor that sets the initial and goal states
a method goal_test(state) that returns True if the given state is a goal state
a method result(state, action) that returns the new state reached from the given state and the given action. Assume that the action is valid.
a method actions(state) that returns a list of valid actions in the given state
It is recommended to implement the methods in the order above and test each method individually
Do not change anything in search.py
search.py --> https://github.com/aimacode/aima-python/blob/master/search.py#L426
wgc --> https://en.wikipedia.org/wiki/Wolf,_goat_and_cabbage_problem
also, please pass these unit tests
Step by Step Solution
There are 3 Steps involved in it
Step: 1
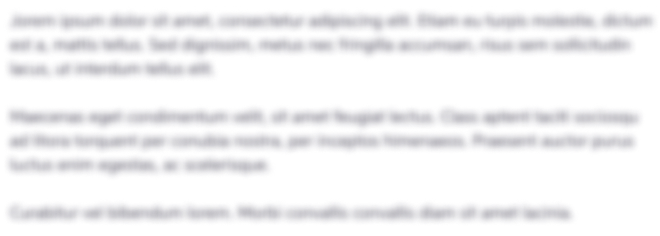
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started