Question
Write a Python function that determines whether a given list of integers represents a valid UPC(also known as barcode). Use a loop to apply the
Write a Python function that determines whether a given list of integers represents a valid UPC(also known as barcode). Use a loop to apply the algorithm described here. UPC codes can be different lengths, so dont assume itll always be exactly 12 digits.
For UPC numbers, the validation algorithm proceeds on the number from right to left:
-
Digits in even positions, including zero: no change
-
Digits in odd positions: *3
-
Sum the results
If its a valid UPC number, this result is a multiple of 10.
An example of a UPC number is is 0 7 3 8 5 4 0 0 8 0 8 9.
We apply the algorithm (the number above is written from left to right, but the algorithm goes right to left, so we say 9 is at position 0, 8 is at position 1, etc.):
9+38+0+38+0+30+4+35+8+33+7+30
= 9 + 24 + 24 + 4 + 15 + 8 + 9 + 7
= 100
And were done! Weve verified that this, in fact, a valid UPC number.
**Special case: If the input is less than 2 digits long, or if all the digits have value 0, the code is not valid.**
Your function must meet the following specifications:
-
Name: is_valid_upc
-
Input: List of integers, a possible UPC number
-
Returns: Boolean, indicating whether the given input is valid or not
********must pass the following tests below**********
def test_valid_upc(): ''' Function test_valid_upc Input: Nothing Returns: int, number of tests that failed. Does: Invokes the is_valid_upc function on several different inputs, expecting the function to return True. Counts the number of times it returns False instead, chalking them up as fails. ''' num_failed = 0 # Test 1: 12-digit UPC, starts with 0 test_input = [0, 7, 3, 8, 5, 4, 0 ,0, 8, 0, 8, 9] print('Testing', test_input, 'is valid...', end = '') if is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 2: 12-digit UPC, starts/ends with non-zero test_input = [7, 1, 8, 1, 0, 3, 1, 6, 7, 9, 5, 6] print('Testing', test_input, 'is valid...', end = '') if is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 3: 13-digit UPC, starts/ends with non-zero test_input = [9, 7, 8, 1, 4, 9, 1, 9, 3, 9, 3, 6, 9] print('Testing', test_input, 'is valid...', end = '') if is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 4: 13-digit UPC, ends with zero test_input = [9, 7, 8, 1, 4, 9, 4, 9, 6, 9, 6, 6, 0] print('Testing', test_input, 'is valid...', end = '') if is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 5: 2-digit UPC, still valid test_input = [7, 9] print('Testing', test_input, 'is valid...', end = '') if is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 6: 5-digit UPC, still valid test_input = [0, 7, 9, 0, 0] print('Testing', test_input, 'is valid...', end = '') if is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 return num_failed def test_invalid_upc(): ''' Function test_invalid_upc Input: Nothing Returns: int, number of tests that failed. Does: Invokes the is_valid_upc function on several different inputs, expecting the function to return False. Counts the number of times it returns True instead, chalking them up as fails. ''' num_failed = 0 # Test 1: 12-digit UPC, mistyped a 6 as 5 from a valid one test_input= [7, 1, 8, 1, 0, 3, 1, 6, 7, 9, 5, 5] print('Testing', test_input, 'is not valid...', end = '') if not is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 2: 12-digit UPC, transposed two numbers from a valid one test_input = [0, 7, 3, 8, 4, 5, 0, 0, 8, 0, 8, 9] print('Testing', test_input, 'is not valid...', end = '') if not is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 3: 13-digit UPC, transposed two numbers from a valid one test_input = [9, 8, 7, 1, 4, 9, 1, 9, 3, 9, 3, 6, 9] print('Testing', test_input, 'is not valid...', end = '') if not is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 4: 2 digits, but invalid test_input = [9, 7] print('Testing', test_input, 'is not valid...', end = '') if not is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 5: 1-digit UPC, invalid by default test_input = [1] print('Testing', test_input, 'is not valid...', end = '') if not is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 6: 0-digit UPC, invalid by default test_input = [] print('Testing', test_input, 'is not valid...', end = '') if not is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 # Test 7: 12-digit UPC, but all zeroes, so invalid test_input = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0] print('Testing', test_input, 'is not valid...', end = '') if not is_valid_upc(test_input): print('SUCCESS! ') else: print('FAIL :( ') num_failed += 1 return num_failed def main(): print('Testing VALID upc numbers. ') num_fails = test_valid_upc() if num_fails == 0: print('All valid tests passed! ') else: print('Sorry, something failed. Go back and fix pls. ')
Step by Step Solution
There are 3 Steps involved in it
Step: 1
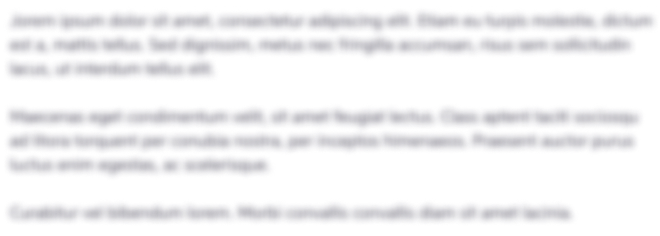
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started