Question
Write a Python program to assign passengers seats in an airplane. Assume a small airplane with seat numbering as follows. Row 1 A B C
Write a Python program to assign passengers seats in an airplane. Assume a small airplane with seat numbering as follows.
Row | |||||
1 | A | B | C | D | |
2 | A | B | C | D | |
3 | A | B | C | D | |
4 | A | B | C | D | |
5 | A | B | C | D | |
6 | A | B | C | D | |
7 | A | B | C | D |
The program should display the seat pattern, with an X making the seats already assigned. For example, after seats 1A, 2B and 4C are taken the display should look like this:
Row | |||||
1 | X | B | C | D | |
2 | A | X | C | D | |
3 | A | B | C | D | |
4 | A | B | X | D | |
5 | A | B | C | D | |
6 | A | B | C | D | |
7 | A | B | C | D |
The program should display the seats available, followed by a message to select the Aisle/Seat, and mark the seat with an X until all seats are filled or the user ends the program. If a seat is already assigned, tell the user.
- You must use a function to display the seating map
- You must use functions that allows the user to enter the row and seat number
- You must use a function that asks the user in they want to continue or stop. The function should only accept an uppercase or lowercase y or n.
---
Use the following snippets pre-done:
def display_seat_map(aA, aB, aC, aD):
for r in range (1, 8):
print(" ", r, " ", aA[r]," ", aB[r], " ", aC[r], " ", aD[r])
Use this code for the seat map
aisleA = ["","A","A","A","A","A","A","A"]
aisleB = ["","B","B","B","B","B","B","B"]
aisleC = ["","C","C","C","C","C","C","C"]
aisleD = ["","D","D","D","D","D","D","D"]
display_seat_map(aisleA, aisleB, aisleC, aisleD)
Notice that whenever you want to display the seat map you have to send it the 4 lists (aisleA, aisleB, aisleC and aisleD.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
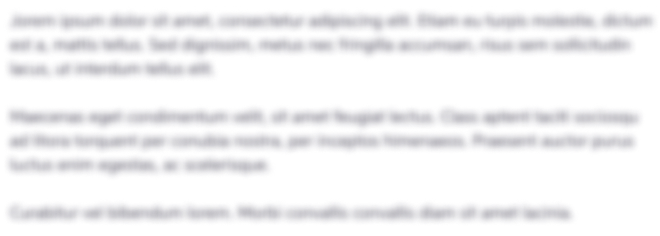
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started