Question
Write a Python script that implements the following functions **********************test.py******************* lst = [7, 2, 12, 9, 15, 4, 11, 5] print('The sum of the elemenst,
Write a Python script that implements the following functions
**********************test.py*******************
lst = [7, 2, 12, 9, 15, 4, 11, 5] print('The sum of the elemenst, left to right, without exceeding the') print('threshold 40 is ', sum_threshold(40, *lst)) print('The same but with a threshold of 70: ', sum_threshold(70, *lst)) print('With an empty list of numbers: ', sum_threshold(50))
print('my_slice(lst): ', my_slice(lst)) print('my_slice(lst, start=2, step=1): ', my_slice(lst, start=2, step=1)) print('my_slice(lst, start=2, length=5): ', my_slice(lst, start=2, length=5)) print('my_slice(lst, length=5): ', my_slice(lst, length=5))
print('with_factors(lst, [2, 5]): ', with_factors(lst, [2, 5]))
print('pairs_factors(lst): ', pairs_factors(lst))
Write a Python script that implements the following functions. Do not have any
functions input or output values. All values used by a function should be passed to it as arguments.
The value computed by a function should be returned, not output by it. Use the test code shown
below (and provided on Blackboard), which satisfies these constraints and outputs the results of
all function applications so we can test your implementations. The test code assigns to lst a list
of integers that is used as the running list for testing the functions. When we say below that a
function is passed the running list of integers, it could actually be passed any list of integers; it just
turns out that the running list is what is passed in our test code.
A function sum_threshold() that is passed as its first argument an integer, call it , to be
used as a threshold and thereafter any number of other integer arguments. It returns the sum
of the arguments after , calculated from left to right until adding the next would exceed ;
if the sum of all these arguments is less than or equal to , then this sum is returned. If only
the argument is provided, return 0. Use the arbitrary argument lists technique., with *.
(To use the list lst set at the beginning, you have to unpack it (use a *) when you provide it
as an argument. Note that, if you do this, youll be unpacking the list (with *) when you pass
it in and packing the arguments back up (using again a *) in the argument list in the function
definition.) The easy way to implement this involves a beak.
A function my_slice() that, by default, returns a slice consisting of every second element
of the list passed to it. There is a parameter start with default 0 that determines the first
index of the slice returned. Parameter step, with default 2, determines the spacing between
the elements in the input list that are included in the returned list. (E.g., with step=2, every
other element is included; with step = 3, every third element is included.) And parameter
length determines the length of the input list (starting at start) that is considered in
producing the returned slice. It is not the length of the returned slice. For example, if the
input list is lst =[0, 1, 2, 3, 4, 5, 6], then
>>> my_slice(lst, length=6, step=1)
[0, 1, 2, 3, 4, 5]
>>> my_slice(lst, length=6, step=2)
[0, 2, 4]
In both cases, the list from index 0 to index 5 is considered since in both cases length=6.
In the first case, every element in this part of the list is included in the returned slice whereas,
in the second case, only every other element in this part of the list is included.
A function with_factors() that is passed the running list of integers (at least in our test
code) and a second list of integers and returns a list containing the elements in the running
list that have at least one element in the second list as a factor. For example, if the running
list is [2, 3, 4, 5, 6, 7, 8, 9] and the list of factors in [2, 3], then the returned
list should be [2, 3, 4, 6, 8, 9]. Within the definition of this function, define another
function, has_factor(), which is passed two arguments: an integer, x, and the list fcts
of factors passed to the top-level function. Then has_factor(x, fcts) returns True if
one of the integers in fcts is a factor of x (and otherwise returns False). You can then use
filter to apply a lambda expression that uses has_factor() to the running list (that was passed in to the function). You will have to convert the filter expression to a list to force it to evaluate.
A function pairs_factors() that is passed the running list of integers, lst, and returns
a list of pairs (2-element tuples), one pair for each element of lst. In a given pair, the first
element of a pair is the element of lst itself, call it x, and the second element is the list of
those elements of lst that are factors of x. Note that neither 1 nor x counts as factors of x.
Implement this using a nested list comprehension. Note that y is a factor of x if and only if
x % y == 0 (and y isnt in {x, 1}). When performing this mod operation, guard against
the case where y is 0, which amounts to division by 0.
The following is test code. It is available as test.py on the page where you got this assignment.
lst = [7, 2, 12, 9, 15, 4, 11, 5]
print('The sum of the elemenst, left to right, without exceeding the')
print('threshold 40 is ', sum_threshold(40, *lst))
print('The same but with a threshold of 70: ', sum_threshold(70, *lst))
print('With an empty list of numbers: ', sum_threshold(50))
print('my_slice(lst): ', my_slice(lst))
print('my_slice(lst, start=2, step=1): ', my_slice(lst, start=2, step=1))
print('my_slice(lst, start=2, length=5): ', my_slice(lst, start=2, length=5))
print('my_slice(lst, length=5): ', my_slice(lst, length=5))
print('with_factors(lst, [2, 5]): ', with_factors(lst, [2, 5]))
print('pairs_factors(lst): ', pairs_factors(lst))
The following is the output from this test code.
The sum of the elemenst, left to right, without exceeding the
threshold 40 is 30
The same but with a threshold of 70: 65
With an empty list of numbers: 0
my_slice(lst): [7, 12, 15, 11]
my_slice(lst, start=2, step=1): [12, 9, 15, 4, 11, 5]
my_slice(lst, start=2, length=5): [12, 15, 11]
my_slice(lst, length=5): [7, 12, 15]
with_factors(lst, [2, 5]): [2, 12, 15, 4, 5]
pairs_factors(lst): [(7, []), (2, []), (12, [2, 4]), (9, []), (15, [5]),
(4, [2]), (11, []), (5, [])]
Include docstrings with all you function definitions.
Problem 1 (7 points) 1 Write a Python script that implements the following functions Don't have any functions input or output values All values used by a function should be passed to it as arguments The value computed by a function should be returned, not output 1 Use the test code shown below and provided on Blackboard o It satisfies these constraints and outputs the results of all function applications for testing your implementations . The test code assigns to lst a list of integers used as the running list tests Beside being passed the running list of integers, any function could be passed any list of integers 1 sum_threshold() is passed as its 1st argument an integer, call it 0, used as a threshold . This is followed by any number of other integer arguments It returns the sum of the arguments after 0, calculated from left to right until adding the next would exceed 0 . If the sum of all these arguments is so, then this sum is returned If only the o argument is provided, return 0 Use the arbitrary argument lists" technique, with * To use the list ist set at the beginning, unpack it (use a *) when you provide it as an argument . You'll be unpacking the list (with *) when you pass it in and packing the arguments back up (using again a *) in the argument list in the function definition The easy way to implement this involves a beak my_slice () by default returns a slice consisting of every 2nd element of the list passed to it Parameters: start (default O): the 1st index of the slice returned step (default 2): the spacing between the elements in the input list that are included in the slice returned length (default None, meaning to the end) the length of the input list (starting at start) considered in producing the returned slice It's not the length of the returned slice E.g., if the input list is 1st = [0, 1, 2, 3, 4, 5, 6], then >>> my_slice (1st, length=6, step=1) [0, 1, 2, 3, 4, 5] >>> my slice (1st, length=6, step=2) [0, 2, 4] In both cases, the list from index 0 to index 5 is considered: in both cases length=6 In the 1st case, every element in this part of the list is included the returned slice In the 2nd case, only every other element in this part of the list is included. 1 with_factors() is passed the running list of integers (at least in our test code) and a 2nd list of integers It returns a list containing the elements in the running list that have at least 1 element in the 2nd list as a factor 1 E.g., if the running list is [2, 3, 4, 5, 6, 7, 8, 9] and the list of factors in [2, 3], o then the returned list should be [2, 3, 4, 6, 8, 9] Within the definition of this function, define another function, has_factor () . It is passed 2 arguments: an integer, x, and the list fcts of factors passed to the top-level function. has_factor (x, fcts) returns True if one of the integers in fcts is a factor of x . Then use filter to apply a lambda expression that uses has_factor() to the running list (that was passed in to the function) Convert the filter expression to a list to force it to evaluate. 1 1 pairs_factors() is passed the running list of integers, 1st It returns a list of pairs (2-element tuples), one pair for each element of 1st. In a given pair, o the 1st element of a pair is the element of 1st itself, call it x, and o the 2nd element is the list of those elements of 1st that are factors of x Note: neither 1 nor x counts as factors of x Implement this using a nested list comprehension Note: y is a factor of x if and only if x % y == 0 (and y isn't in {x, 1}) . When performing this mod operation, guard against the case where y is 0, which amounts to division by 0 The following is test code available as test.py on the his assignment page lst = [7, 2, 12, 9, 15, 4, 11, 5] print('The sum of the elemenst, left to right, without exceeding the') print('threshold 40 is ', sum_threshold (40, *lst)) print('The same but with a threshold of 70: ', sum threshold (70, *lst)) print('With an empty list of numbers: sum threshold (50)) print('myslice (1st): , my_slice (lst)) print('my slice (1st, start=2, step=1): ', my_slice (1st, start=2, step=1)) print('my_slice (1st, start=2, length=5): ', my_slice (1st, start=2, length=5)) print('myslice (1st, length=5): ', my slice (1st, length=5)) print('with_factors (1st, [2, 5]): ', with_factors (1st, [2, 5])) print('pairs factors (1st): ', pairs factors (lst)) The following is the output from this test code. The sum of the elemenst, left to right, without exceeding the threshold 40 is 30 1st = [7, 2, 12, 9, 15, 4, 11, 5] The same but with a threshold of 70: 65 With an empty list of numbers: 0 my slice (lst): [7, 12, 15, 11] my_slice (1st, start=2, step=1): [12, 9, 15, 4, 11, 5] my slice (1st, start=2, length=5): [12, 15, 11] my_slice (1st, length=5): [7, 12, 15] with factors (1st, [2, 5]): [2, 12, 15, 4, 5] pairs factors (1st): [(7, []), (2, []), (12, [2, 4]), (9, []), (15, [5]), (4, [2]), (11, []), (5, [1] Include docstrings with all you function definitionsStep by Step Solution
There are 3 Steps involved in it
Step: 1
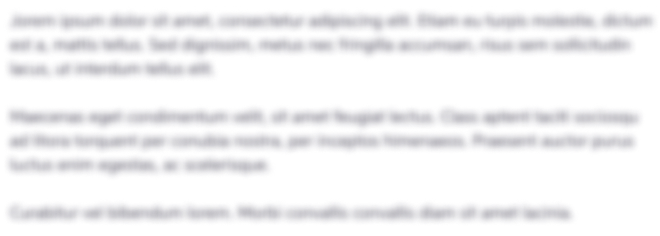
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started