Question
Write a short C/C++ program that reads a 64-bit machine instruction as a hex integer and extracts the values for its components from certain bits,
Write a short C/C++ program that reads a 64-bit machine instruction as a hex integer and extracts the values for its components from certain bits, specified left-to-right, as follows:
Bits 0-4 code (the instruction code) Bits 5-8 ladrm (left address mode) Bits 9-12 radrm (right address mode) Bits 13-19 si (short immediate) Bits 20-25 lreg (left register) Bits 26-31 rreg (right register) Bits 32-63 li (long immediate)
You will read 16 hexadecimal digits, such as 08800080000004D2, which represent the numeric value of the 64 bits to be analyzed. The output for this input string should be:
Instruction: 08800080000004D2
rreg = 0 lreg = 2 si = 0 radrm = 0 ladrm = 1 code = 1 li = 1234
Note that the output numbers are base 10, not hexadecimal. To get full credit for this program, youll need to use a union with a nested bit-field structure in C/C++(use no bitwise operators). If youre using an Intel machine, remember that it is a little-endian machine, which means youll have to reverse the order of the bit-field declarations to extract the correct values. Use the following input strings to test your program:
08800080000004D2 E05000000000008E 1140408300000000 5008000300000000 E800000000000020
Here is a main program to use:
int main( ) { unsigned long long x; while (cin >> hex >> x) { cout << "Instruction: " << hex << x << endl; cout << dec; // Convert back to decimal readStuff(x); cout << endl; } }
For all programming problems, be sure to submit your source code and a copy/screen shot of the execution output. No executables or project files, please.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
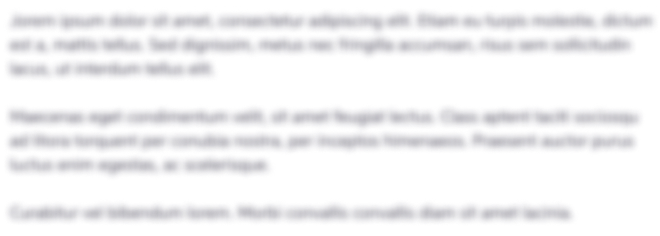
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started