Question
Write a test case (testcase.py) for this Python Hangman game. The following python files provided are hangman.py and word.py. Textfile with the set of random
Write a test case (testcase.py) for this Python Hangman game. The following python files provided are hangman.py and word.py. Textfile with the set of random words is also provided and is under the name hangman_words.txt.
hangman.py
from string import ascii_lowercase from randomWord import random_word
def user_num_attempts(): """Asks user input for how many incorrect attempts for guessing the word before they either win or lose the game.""" while True: num_attempts = input('Select the number of guesses to play with. Pick a number between 10 to 20.') try: num_attempts = int(num_attempts) if 10 <= num_attempts <= 20: return num_attempts else: print('{0} is not between the numbers 10 and 20'.format(num_attempts)) except ValueError: print('{0} is not an integer between the numbers 10 and 20'.format(num_attempts))
def user_word_length(): """Asks user input for the length of word they want for the game.""" while True: word_length = input( 'Select a number to choose the length of word you want for the game. Pick a number between 4 to 16. ') try: word_length = int(word_length) if 4 <= word_length <= 16: return word_length else: print('{0} is not between the numbers 4 and 16'. format(word_length)) except ValueError: print('{0} is not an integer between the numbers 4 and 16'.format(word_length))
def user_word(word, index): """Displays the word for user's game play.""" if len(word) != len(index): raise ValueError('Length of word does not match length of index.') word_display = ''.join([letter if index[i] else '*' for i, letter in enumerate(word)]) return word_display.strip()
def user_next_letter(letters): """Asks user input for their next letter guess.""" if len(letters) == 0: raise ValueError('There are no more remaining letters.') while True: next_letter = input('Your next letter is: ').lower() if len(next_letter) != 1: print('Select a single a letter.') elif next_letter not in ascii_lowercase: print('Letter input must be lowercase only.') elif next_letter not in letters: print('This letter has already been guessed.') else: letters.remove(next_letter) return next_letter
def user_play_hangman_game(): """User plays a game of hangman.""" """If user wins at the end of the game, dataset will be given out and prompt user if they want to play again.""" """If user loses at the end of the game, prompts user if they want to play again."""
print('A game of Hangman is starting') print('') num_of_attempts_remaining = user_num_attempts() word_length = user_word_length()
print('Selecting a word for the game of Hangman... ') word = random_word(word_length) print()
index = [letter not in ascii_lowercase for letter in word] letter = set(ascii_lowercase) incorrect_letters = [] word_solve = False
while num_of_attempts_remaining > 0 and not word_solve: print('Word: {0}'.format(user_word(word, index))) print('Attempts Remaining: {0}'.format(num_of_attempts_remaining)) print('Previous Letter: {0}'.format(' '.join(incorrect_letters)))
nextLetter = user_next_letter(letter)
if nextLetter in word: print('{0} is correct and is in the word'.format(nextLetter)) for i in range(len(word)): if word[i] == nextLetter: index[i] = True
else: print('{0} is incorrect and is not in the word'.format(nextLetter)) num_of_attempts_remaining -= 1 incorrect_letters.append(nextLetter)
if False not in index: word_solve = True print()
print('The Hangman word is {0}'.format(word))
if word_solve: print('Congratulations. You have won the game and may now have access to the datasets.') else: print('Try again to win the game of Hangman.')
play_again = input('Would you like to play another round of Hangman? Please select y') return play_again.lower() == 'y'
if __name__ == '__main__': while user_play_hangman_game(): print()
word.py
import random
list_of_random_words = 'hangman_words.txt' def random_word(word_length): """User gets random word generated for their game of Hangman.""" user_word = 0 current_word = None with open(list_of_random_words, 'r') as file: for game_word in file: if '(' in game_word or ')' in game_word: continue game_word = game_word.strip().lower() if len(game_word) < word_length: continue user_word += 1 if random.randint(1, user_word) == 1: current_word = game_word return current_word
hangman_words.txt
comaetho
untremulent
consignment
termer
karroo
misdeem
intracellular
ewe
formulator
postmammillary
unrotating
drabble
gyroscope
daddy
predeceive
tittivated
sandalwood
rathely
nonslanderous
begrimed
appellee
relievable
hypotrachelium
junkie
brendel
awaiter
bookstack
embussed
scheel
lemminkinen
mismanaging
webworm
rascal
neglecter
nervelessness
burnham
subcontrary
reexamined
undergentleman
visising
nondeleterious
mudcapped
journalizer
unfulsome
blockhouse
mesophyll
talaria
unarrestable
hippogryph
iglu
Step by Step Solution
There are 3 Steps involved in it
Step: 1
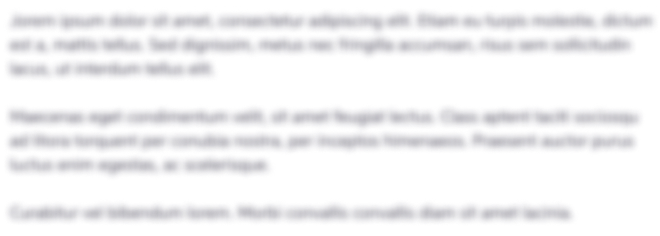
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started