Question
Write an XMLNode .cpp and .h that implements the methods below in c++. XMLNode takes in a file and parses it for the information it
Write an XMLNode .cpp and .h that implements the methods below in c++.
XMLNode takes in a file and parses it for the information it contains, look at parsetool for an example below.
The methods for parsing and write file must utilize recursion!!
Here are the files described above:
PARSETOOL **AN EXAMPLE OF WHAT THIS SHOULD DO BUT NOT RECURSIVE**
*****************************Parsetool.cpp*****************************************
#include "parsetool.h"
#include
#include
#include
#include
using namespace std;
/*********************************************************
* Input: 2 Strings - First is the string to split, second is the
delimiter
* Output: A vector of the split string components
* Purpose: splits a string into parts based on a delimiter
* ******************************************************/
vector split (string line, char delimiter) {
vector splitStrings;
int start = 0;
int end = line.find(delimiter);
//cout
while (end != string::npos ) {
string subs = line.substr(start, end-start);
//cout
start = end+1;
end = line.find(delimiter, start);
//cout
//cout
splitStrings.push_back(subs);
}
string subs = line.substr(start, line.length()-start);
//cout
splitStrings.push_back(subs);
return splitStrings;
}
/*************************************************
* Input: String -- single line from an XML file in the form
* contents
* Output: Node - contents of a node
* Purpose: Converts a single line into a node for processing
**************************************************/
Node parseline (string line) {
Node newNode;
// identifies the start of a node
int startOfTag = 1;
int endOfTag = line.find(">");
string tagString = line.substr(startOfTag, endOfTag - startOfTag);
//cout
//cout
// pulls the name and properties out of the node
vector properties;
properties = split(tagString, ' ');
for (auto val: properties) {
//cout
vector props = split(val, '=');
if (props.size() != 2) { // we know this is the name
of the node
cout
newNode.name = props[0];
}
else { // got an actual property
newNode.properties.insert(pair(props[0], props[1]));
}
}
// gets the content from the node
// the contents could be more nodes, so it is up to you to parse the
contents for more nodes (think, recursion!)
int startOfContent = endOfTag+1;
string endNode = "";
int endOfContent = line.rfind(endNode);
//cout
endl;
newNode.content = line.substr(startOfContent, endOfContent -
startOfContent);
return newNode;
}
**************************************************************************************
**********************************parsetool.h**************************************
#ifndef _PARSETOOLS_H_
#define _PARSETOOLS_H_
#include
#include
#include
using namespace std;
struct Node {
string name; // name of the node
map properties; // any properties found in the node
start tag
string content; // if the node has content between the start and end
tag, put it here
vector children; // if the node has children, create new
Nodes and store their pointers here
};
Node parseline (string);
vector split (string, char);
#endif
****************************************************************************
**********************testparsetool.cpp**********************************
#include
#include "parsetool.h"
#include
#include
#include
using namespace std;
int main () {
ifstream test;
test.open("test.xml");
while (!test.eof()) {
string line;
getline(test, line);
if (line.length() == 0) { // skip empty liness
continue;
}
Node myNode = parseline(line); // turns a line into a
node
// print out the Node info
cout
cout
for(const auto& kv : myNode.properties) {
cout
}
cout
cout
}
test.close();
return 0;
}
***********************************************************************
*********************test.xml****************************************
This is a page
This is the child
This is the child
name=other>another child
I am a
grandchild
*********************************************************************************
HERE IS THE DRIVER WE ARE GIVEN AND SOME THE TEST FILES
**************************XMLDriver.cpp***************************************
#include #include "XMLNode.h" using namespace std; int main () { XMLNode test1; XMLNode test2("test2.xml"); test1.ParseFile("test1.xml"); test1.WriteFile("test3.xml"); XMLNode test3("test3.xml"); //test1.PrettyPrintDocument(); //test2.PrettyPrintDocument(); //test3.PrettyPrintDocument(); return 0; }
***************************************************************************
***************************test1.xml**************************************
This is the childanother child
***********************************************************************************
*************************test2.xml**************************************
I am a grandchild
**********************************************************************************
Many documents are structured such as HTML and XML (including RDF, OWL, etc.) These documents tend to consist of a head node or document node which has multiple properties and child nades. Each child nade can also have multiple properties and children. Besides the WWW, other systems such as knowledge management and semantic web systems use these formats to represent knowledge and make it easy for algorithms to parse and utilize the data. In this assignment, you will create an XMLNode Class that contains a Map of properties and a Vector of Children. Within the node class you will also include a method that uses a recursive algorithm to read in a structured file and a method to write out the data in the class as a structured file. I have provided you with some code to help you get started. You can convert this code into your class or write everything from scratch. The included code demonstrates how you can read in an XML file and parse it for properties and content. My code is not recursive though, so if a line of the XML file has children, they are just treated as content. It is your job to convert the struct and helper functions into a class that recursively reads and writes XML files parsetoolh test.xml Note: My code uses C++ constructs that require you to compile with at least C++11 or higher. Note: I have provided a very limited XML format. Yours does not need to he able to parse any arbitrary XML fle. You can assume the following things about the format: Properties are a single word. They don't contain any special characters. An entire tree of nodes is contained on a single line. . If a node has a chid, it does not have any other content Methods you must implement Public Constructor (ilename) ParseFileenae) Must use recursion WriteFile (file) Must use recursion PrettyPrintDocument 0 Must use recursion hint: This will use a lot of the same functionality as WriteFile, so share as much code as possible between the two with generic functions. PrettyPrintDocument should print the Node structure using tabbed spacing similar to what I have done in the testparsetool.cpp (but it should support multiple levels of children). As discussed in class this is output to the screen. If you output this to a file already instead, then that will be fine too. Protected/Private You will be expected to create helper functions as needed for internal use by your class Deliverables . XMLNode.h Class Signature XMLDriver.cpp - Test program (provided by me) test1.xml and test2.xml Test input (provided by mel test.sh--testing script (provided by me) Many documents are structured such as HTML and XML (including RDF, OWL, etc.) These documents tend to consist of a head node or document node which has multiple properties and child nades. Each child nade can also have multiple properties and children. Besides the WWW, other systems such as knowledge management and semantic web systems use these formats to represent knowledge and make it easy for algorithms to parse and utilize the data. In this assignment, you will create an XMLNode Class that contains a Map of properties and a Vector of Children. Within the node class you will also include a method that uses a recursive algorithm to read in a structured file and a method to write out the data in the class as a structured file. I have provided you with some code to help you get started. You can convert this code into your class or write everything from scratch. The included code demonstrates how you can read in an XML file and parse it for properties and content. My code is not recursive though, so if a line of the XML file has children, they are just treated as content. It is your job to convert the struct and helper functions into a class that recursively reads and writes XML files parsetoolh test.xml Note: My code uses C++ constructs that require you to compile with at least C++11 or higher. Note: I have provided a very limited XML format. Yours does not need to he able to parse any arbitrary XML fle. You can assume the following things about the format: Properties are a single word. They don't contain any special characters. An entire tree of nodes is contained on a single line. . If a node has a chid, it does not have any other content Methods you must implement Public Constructor (ilename) ParseFileenae) Must use recursion WriteFile (file) Must use recursion PrettyPrintDocument 0 Must use recursion hint: This will use a lot of the same functionality as WriteFile, so share as much code as possible between the two with generic functions. PrettyPrintDocument should print the Node structure using tabbed spacing similar to what I have done in the testparsetool.cpp (but it should support multiple levels of children). As discussed in class this is output to the screen. If you output this to a file already instead, then that will be fine too. Protected/Private You will be expected to create helper functions as needed for internal use by your class Deliverables . XMLNode.h Class Signature XMLDriver.cpp - Test program (provided by me) test1.xml and test2.xml Test input (provided by mel test.sh--testing script (provided by me)Step by Step Solution
There are 3 Steps involved in it
Step: 1
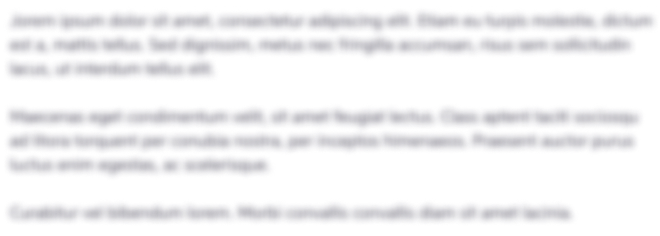
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started