Question
write and implement a C++ program to implement two search algorithms (linear search, binary search and print fuction) on randomly generated integers stored in vectors.
write and implement a C++ program to implement two search algorithms (linear search, binary search and print fuction) on randomly generated integers stored in vectors.
#include
const int DATA_RANGE = 100; const int DATA_SIZE = 100; const int DATA_SEED = 7; const int SEARCH_SEED = 9;
bool linear_search(const vector
{ }
bool binary_search(const vector
{ }
void print_vec( const vector
{ }
void average_comparisons(const vector
int random_number() { return rand()%DATA_RANGE+1; }
int main () {
// -------- create unique random numbers ------------------// vector
cout << "------ Data source: " << inputVec.size() << " unique random numbers ------" << endl; print_vec(inputVec); cout << endl;
// -------- create random numbers to be searched ---------// vector
cout << "------ " << searchVec.size() << " random numbers to be searched: ------" << endl; print_vec(searchVec); cout << endl;
cout << "Linear search: "; average_comparisons(inputVec, searchVec, linear_search); cout << "Binary search: "; average_comparisons(inputVec, searchVec, binary_search);
sort(inputVec.begin(), inputVec.end()); cout << "------- numbers in data source are now sorted ---------" << endl << endl; cout << "Linear search: "; average_comparisons(inputVec, searchVec, linear_search); cout << "Binary search: "; average_comparisons(inputVec, searchVec, binary_search);
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
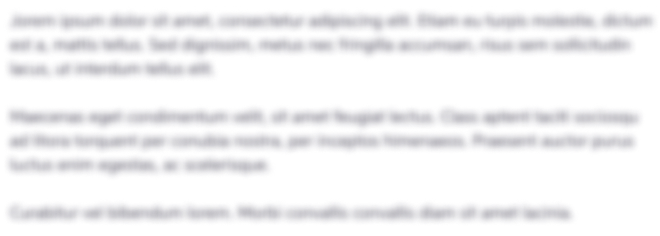
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started