Question
Write C++ Code sample code (3 in total) 1---ECArrayofStrings.c #include #include ECArrayofStrings.h using namespace std; ECArrayofStrings :: ECArrayofStrings() { } ECArrayofStrings :: ~ECArrayofStrings() { //
Write C++ Code
sample code (3 in total)
1---ECArrayofStrings.c
#include
#include "ECArrayofStrings.h"
using namespace std;
ECArrayofStrings :: ECArrayofStrings()
{
}
ECArrayofStrings :: ~ECArrayofStrings()
{
// your code goes here
}
void ECArrayofStrings :: AddString( const string &strToAdd )
{
// your code goes here
}
int ECArrayofStrings :: GetNumofStrings() const
{
// your code goes here
}
int ECArrayofStrings :: GetMaxLen() const
{
// your code goes here
}
string ECArrayofStrings :: GetLongestCommonPrefix() const
{
// your code goes here
}
string ECArrayofStrings :: Concatnate() const
{
// your code goes here
}
void ECArrayofStrings:: Dump() const
{
// your code goes here
}
2 --- ECArrayofStrings.h
#ifndef EC_ARRAY_OF_STRINGS #define EC_ARRAY_OF_STRINGS
using namespace std; #include
#include
// define a class for an array of strings // use vector
private: // your code };
#endif
3--- ECArrayofStringsMain.c
#include
#include"ECArrayofStrings.h"
int main(void)
{
ECArrayofStrings as;
as.AddString(std::string("hello1"));
as.AddString(std::string("hello2"));
as.AddString(std::string("hello3"));
as.AddString(std::string("hello4"));
as.AddString(std::string("hello"));
std::cout
as.Dump();
std::cout
std::cout
std::cout
return 0;
}
3 Array of strings In this assignment, you will implement a class called ECArrayofStrings. This class stores multiple strings and support multiple operations related to the strings. The header file of ECArrayofStrings is provided in the starter code. Here are some explanations. 1. We use STL vector to implement an array of strings. 2. Constructor doesn't take any input. 3. AddString: add a new string into the array of strings. 4. GetNumofStrings: return the total number of strings in the array. For example, suppose you use AddString to add two strings "abc" and "defg". Then GetNumofStrings should return "2". 5. GetMaxLen: return the maximum length of the (longest) string in the array. In the above example, this should return " 4 ". 6. GetLongestCommonPrefix: return the longest common prefix of all the strings. This is exactly the same as programming assignment 1 is supposed to do. You just need to turn this into a member function. 7. Concatnate: return the concatenated string. In the above example, return "abcdefg". Note: you need to follow the order of adding of strings: the earlier added strings should be dealt with earlier; the same for all the member functions in the class. 8. Dump: output to the standard output all the strings in the array each in new line (again, in the order of their addition). 9. GetLongestCommonPrefixofTwo: this is a private function (meaning that it is meant to be implementation only that is to be used in this class). You must use this in the GetLongestCommonPrefix implementation
Step by Step Solution
There are 3 Steps involved in it
Step: 1
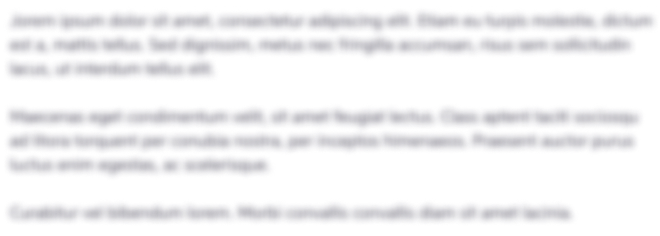
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started