Question
Write C++ code to implement a class Bubble. The implementation will include: a header file, an implementation file, an application file. Each line should have
Write C++ code to implement a class Bubble. The implementation will include: a header file, an implementation file, an application file. Each line should have an explanation.
The header file will be:
#ifndef _H_LENGTH_ #define _H_LENGTH_ #include
using namespace std;
class Bubble {
Private:
radius: real number
Public:
Default constructor: Set radius to 5.0
Constructor (with one parameter, double): Sets radius=
getRadius (no parameter): Returns (double) the value of the radius
setRadius (with one parameter, double): sets radius=
getVolume(): returns(double) the volume of the bubble( 4/3*Pi*r^3)
Add (another bubble object as a parameter):
Add the volume of the parameter bubble to the volume of self (ex. Radius will be changed accordingly)
Operator +:
Which allows two bubbles to be added to form a new bubble such that Volume(bNew)= Volume(b1) + volume(b2). Radius of the new bubble will be set accordingly ( Ex. Bubble b1, b2, bNew . bNew= b1+b2)
Operator -;
Which allows a bubble to separate form a given bubble by leaving a smaller remainder bubble. Such that, Volume(bRemainder)= volume(bOrigin) volume(bSeparated). The bubble being separated must be smaller than the original bubble, otherwise the result will be same as the original bubble. Radius of the new remainder buble will be set accordingly (ex. Bubble bOrig, bRem, bSep bRem = bOrig bSep)
};
#endif
An application file will be:
#include
int main()
{
Bubble b1, b2(7.0), b3;
cout << "B1's R:" << b1.getRadius() << endl;
cout << "B1's V:" << b1.getVolume() << endl;
cout << "B2's R:" << b2.getRadius() << endl;
cout << "B2's V:" << b2.getVolume() << endl;
b3.setRadius(6);
cout << "B3's R:" << b3.getRadius() << endl;
cout << "B3's V:" << b3.getVolume() << endl;
Bubble b4(3.4);
cout << "B4's R:" << b4.getRadius() << endl;
cout << "B4's V:" << b4.getVolume() << endl;
Bubble b5 = b3;
b5.add(b4);
cout << "B5's R:" << b5.getRadius() << endl;
cout << "B5's V:" << b5.getVolume() << endl;
Bubble b6 = b3 + b4;
cout << "B6's R:" << b6.getRadius() << endl;
cout << "B6's V:" << b6.getVolume() << endl;
Bubble b7 = b3 - b4;
cout << "B7's R:" << b7.getRadius() << endl;
cout << "B7's V:" << b7.getVolume() << endl;
return 0;
}
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
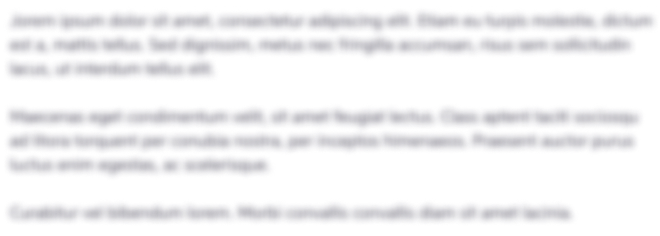
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started