Question
Write C++ code with comments to circulate a book to the employees in the system. The circulation starts on a given date. Further, the system
Write C++ code with comments to circulate a book to the employees in the system. The circulation starts on a given date. Further, the system makes a queue of employees that should receive the book. The queue should be prioritized based on two factors: (1) the total waiting time for the employee: How many days the employee waited to get a book since the beginning of the circulation. (2) The total retaining time: How many days the employee retained books.
I already have a library class with employees and books. I also have a priority queue already.
#pragma once
#ifndef LIBRARY_H
#define LIBRARY_H
#include
#include
#include
#include
#include
#include "Date.h"
namespace BD {
class Library
{
public:
//Data Containers for Employees and Books
struct Employee {
std::string name;
int waiting_time = 0;
int retaining_time = 0;
vector
};
struct Book {
std::string title;
Date start_circ;
Date end_circ;
queue
};
Library();
void Add_Book(const std::string &book_title);
void Add_Employee(const std::string &emp_name);
void Assign_Books();
void Test_Output();
private:
list
list
vector
vector
int total_books;
//Validates input for Adding Employee and Book
bool Validate_Str(const std::string &in_str);
};
}
#endif
#include "Library.h"
using namespace BD;
BD::Library::Library(){}
void BD::Library::Add_Book(const std::string &book_title)
{ //Need to add Error Handling for , empty str, and other whitespace chars ####<=======NOTE
if(Validate_Str(book_title)){
Book new_book;
new_book.title = book_title;
book_list.push_back(new_book);
archieved_list.push_back(new_book); //Create, and add new_book as archieved.
total_books += 1; //Increment total books
}
else
std::cout << "ERROR: Cannot Add Book without an Alphabetic Character as the first character." << endl;
//#####May need an assign Employee function
}
void BD::Library::Add_Employee(const std::string &emp_name) {
if (Validate_Str(emp_name)) { //Error Handle Employee Name
for (std::vector
if (emp_iter->name == emp_name) { //Check for possible duplication
std::cout << "ERROR: There is already a Library Member with the name: " << emp_iter->name << endl
<< "Please try again, or add a distinguishing character." << endl;
return;
}
Employee new_emp; //Create and add new employee
new_emp.name = emp_name;
employee_list.push_back(new_emp);
}
else
std::cout << "ERROR: Employee's Name must start with Alphabetical Character." << endl;
//#####May need an assign books function.
}
bool BD::Library::Validate_Str(const std::string &in_str) {
if (!in_str.empty()) {
std::string::const_iterator str_iter = in_str.begin();
if ((int(*str_iter) >= 65 && int(*str_iter) <= 122) && (int(*str_iter) <= 90 || int(*str_iter) >= 97))
return true;
}
return false;
}
void BD::Library::Test_Output() {
for (std::vector
std::cout << test_it->title << endl;
if (Validate_Str(test_it->title))
std::cout << "TRUE!!!" << endl << endl;
else
std::cout << "FALSE" << endl << endl;
for (std::vector
std::cout << emp_iter->name << endl;
}
}
BD::Library::Assign_Books() {
//Assigns Books to new employee, adds employee's name to active circulation list.
return;
}
This is the priority queue im working on
#include
#include
#include
#include
using namespace std;
// Node declaration and properties
struct node{
int priority;
int data;
struct node *link;
};
// Class implementing the Priority Queue
class PQ{
private:
node *front;
public:
PQ(){
front = NULL;
}
// Function to push an element into the Priority Queue
void push(int number, int priority){
node *demo, *q;
demo = new node;
demo->data = number;
demo->priority = priority;
if (front == NULL || priority < front->priority){
demo->link = front;
front = demo;
}
else{
q = front;
while (q->link != NULL && q->link->priority <= priority)
q = q->link;
demo->link = q->link;
q->link = demo;
}
}
// Method to pop the highest priority element in the queue
void pop(){
node *temp;
if (front == NULL)
cout << "There is no item in the queue." << endl;
else{
temp = front;
cout << "Deleted item is: " << temp->data << endl;
front = front->link;
free(temp);
}
}
// Displaying the elements of Priority Queue
void display(){
node *ptr;
ptr = front;
if (front == NULL)
cout << "Priority Queue is empty ";
else{
cout << "Priority Queue is : ";
cout << "Priority Item ";
while (ptr != NULL)
{
cout << ptr->priority << " " << ptr->data << endl;
ptr = ptr->link;
}
}
}
};
// Main function that tests the priority queue
int main(){
int choice, number, priority;
PQ pq;
do
{
cout << "1.Push an element into the priority queue ";
cout << "2.Pop an element from the priority queue ";
cout << "3.Display the priority queue ";
cout << "4.Quit ";
cout << "Enter your choice : ";
cin >> choice;
switch (choice)
{
case 1:
cout << "Enter the value to be added in the queue : ";
cin >> number;
cout << "Enter its priority : ";
cin >> priority;
pq.push(number, priority);
break;
case 2:
pq.pop();
break;
case 3:
pq.display();
break;
case 4:
break;
default:
cout << "Wrong choice ";
}
}
while (choice != 4);
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
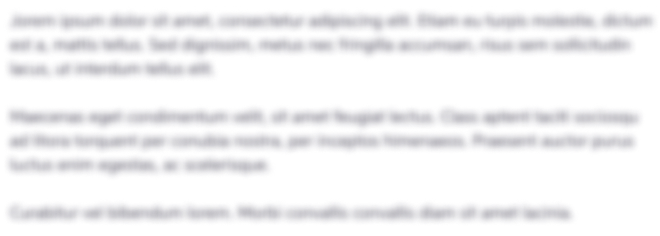
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started