Question
Write c++ files for the following programs: trax.h,trax.cpp, gladiator.cpp, gladiator.h, murmillo.h, murmillo.cpp, retiarius.h, retiarius.cpp, dimachaerus.cpp, dimachaerus.h UMLS are as follows: 3.1.1 Gladiator Parent Class gladiator
Write c++ files for the following programs:
trax.h,trax.cpp, gladiator.cpp, gladiator.h, murmillo.h, murmillo.cpp,
retiarius.h, retiarius.cpp, dimachaerus.cpp, dimachaerus.h
UMLS are as follows:
3.1.1 Gladiator Parent Class
gladiator
-HP:int
-AS:double
-DS:double
-appeal:int
-damage:int
-seedR:int
---------------------------
+gladiator()
+setRandomSeed():void
+getSeedR() const:int
+setSeedR(s:int):void
+getHP() const:int
+getAS() const:double
+getDS() const:double
+getAppeal() const:int
+getDamage() const:int
+setHP(s:int):void
+setAS(s:double):void
+setDS(s:double):void
+setAppeal(s:int):void
+setDamage(s:int):void
+attack(glad: gladiator &):int
+~gladiator()
+taunt()=0:string
+print()=0:void
+defenceBolster():void
+specialAction():void
The variables are as follows:
HP: This is the Gladiator's health. A gladiator is able to ght with health less
than 0, but doing so decreases their eectiveness dramatically. This reects the
more sportive nature of the arena where the ghts are done for entertainment and
casualties are by and large, very rare statistical events.
AS: This is their Attack Skill, and represents the probability of an attack performed
by them, succeeding. It will range from 0 to 1.
DS: This is their Defence Skill, and represents the probability of an attack performed
to them, failing in part to their own natural defence and armour. It represents their
chance of surviving an enemy attack unscathed. It will range from 0 to 1.
appeal: This represents their charisma and crowd appeal. The higher this number,
the greater the bonus applied to renown scored when a gladiator competes.
damage: This is the physical damage that a gladiator will cause with their weapons.
Higher values represent stronger or more potent weapons and can quickly reduce
opponents to 0 health.
The functions are as follows:
The functions are as follows:
gladiator: This is the default destructor for the class.
setRandomSeed: This uses the seedR variable to seed the random number generator
for the gladiator class.
taunt: This is a pure virtual function in this class. It will represent a unique and
specic taunt that every gladiator class has and can do when they ght. See the
specic classes for more details.
attack: It will be used specically to conduct a single attack from a gladiator to
another. The exact functioning of this follows the given process:
1. Generate a random number between 0 and 1. If this number is less than the
attacking Gladiator's AS, the attack is successful and generates 10 Renown
points. If it misses it will instead be -10 Renown points.
2. Generate another random number between 0 and 1. If this number is less than
or equal to the DS of the attacked gladiator, their armour and skill saves them
and the attack is nullied. If not the attack will cause them damage.
3. Reduce the attacked gladiator's HP value by the damage value of the attacking
gladiator. If this reduces a gladiator to 0 or fewer HP, it adds an additional 30
Renown points.
4. Return the number of Renown points generated plus the appeal of that gladi-
ator as the total Renown for that attack.
This is a process that follows a certain structure. A gladiator will not need to test
their DS if an attack does not hit them for example. If the attack misses, it misses
and nothing further will happen. If the attack lands, then it should be necessary to
check if the attack will bypass defences.
print: This is a pure virtual function used to print out the gladiator's statistics.
defenceBolster: This represents a defensive bolster. A gladiator will use this to
increase their stats and recover during the ght and depending on the type, dierent
eects will result.
specialAction: This represents a special action that tries to bolster the gladiator's
popularity with the crowd.
getter and setter: Each of the variables of the class has a getter and a setter assigned
to it that simply returns or overwrites the variable's value.
3.1.2 Trax
trax
-sprints:int
---------------------------
+getSprints():int
+setSprints(s:int):void
+trax()
+~trax()
+taunt():string
+defenceBolster():void
+specialAction():void
+print():void
The variables specic to the Trax are:
sprints: This is a special stat used to represent the mobility of the Trax Gladiator.
They will use their sprints as part of their special action but they only have a nite
number of them available in any given ght.
The methods of the class are:
getter and setter: A specic getter and setter for the sprints variable.
trax(): This is the destructor for the class. It will print "Trax removed" without
the quotation marks and with a new line at the end.
trax: This constructor has a default prole to use when creating a Trax gladiator.
The statistics that are the default values for the class are below:
1. HP: 16
2. AS: 0.65
3. DS: 0.55
4. appeal: 5
5. damage: 1
6. sprints: 5
7. seedR: 100
print: This method will print out the statistics of the gladiator in a row by row
fashion. For example:
HP: 16
AS: 0.65
DS: 0.55
Appeal: 1
Damage: 1
Sprints: 5
SeedR: 100
taunt: This method will return a unique message. The message is "THRACE
REMEMBERS!", without quotations.
defenceBolster: This bolster will decrease the Trax's AS by 0.05 and increase his DS
by 0.15, representing him focusing more on his greater mobility to the detriment of
his short ranged weapon.
specialAction: When activated, this must check how many sprints the Trax has left.
If the Trax has sprints left, he will use one and increase his appeal by 2 and his AS
by 0.1.
3.1.3 Murmillo
murmillo
---------------------------
+murmillo()
+~murmillo()
+taunt():string
+defenceBolster():void
+specialAction():void
+print():void
The methods of the class are:
murmillo: This constructor has a default prole to use when creating a Murmillo
gladiator. The statistics that are the default values for the class are below:
1. HP: 30
2. AS: 0.45
3. DS: 0.75
4. appeal: 1
5. damage: 1
6. seedR: 100
murmillo: This is the destructor for the class. It will print "Murmillo removed"
without the quotation marks and with a new line at the end.
taunt: This method will return a unique message. The message is "I AM INVIN-
CIBLE!", without quotations
print: This method will print out the statistics of the gladiator in a row by row
fashion. For example:
HP: 16
AS: 0.65
DS: 0.55
Appeal: 1
Damage: 1
SeedR: 100
defenceBolster: This bolster will increase his DS by 0.01 and increase his damage
by 1, representing him focusing entirely on guarding any attacks coming from his
opponent and being more dangerous on a counter-attack.
specialAction: When activated, the Murmillo will change his ghting style depend-
ing on his current condition. Adjust his stats based on the following conditions:
1. If his health is less than or equal to 5, drop his DS to 0.5 and increase his AS
by 0.2. Additionally, increase his damage to 3.
2. If his health is greater than 5 but less than or equal to 10, drop his AS to 0.35
and increase his appeal by 2.
3. If his health is greater than 10, increase his appeal by 3.
3.1.4 Retiarius
retiarius
-control:int
---------------------------
+getControl():int
+setControl(s:int):void
+retiarius()
+~retiarius()
+taunt():string
+defenceBolster():void
+specialAction():void
+print():void
The variables specic to the Retiarius are:
control: This variable represents the capacity of the Retiarius to use his net to
control the enemy ghter. The longer a ght drags on, the more the Retiarius is
likely to critically expose an enemy to an attack. This variable has a getter and a
setter.
The methods of the class are:
retiarius: This is the destructor for the class. It will print "Retiarius removed"
without the quotation marks and with a new line at the end.
retiarius: This constructor has a default prole to use when creating a Retiarius
gladiator. The statistics that are the default values for the class are below:
1. HP: 17
2. AS: 0.70
3. DS: 0.25
4. appeal: 6
5. damage: 3
6. control: 0
7. seedR: 100
print: This method will print out the statistics of the gladiator in a row by row
fashion. For example:
HP: 16
AS: 0.65
DS: 0.55
Appeal: 1
Damage: 3
Control: 5
SeedR: 100
taunt: This method will return a unique message. The message is "NEPTUNE
TAKE YOU!", without quotations.
defenceBolster: This bolster will increase his DS by 0.1 and his AS by 0.01. However
it will decrease his appeal by 1.
specialAction: When activated, the Retiarius increases his control by 1. If the
amount of control he has is a multiple of 2, then he will again an additional 0.05
DS. If it is a multiple of 5, he will gain 2 appeal.
3.1.5 Dimachaerus
dimachaerus
---------------------------
+dimachaerus()
+~dimachaerus()
+taunt():string
+defenceBolster:void
+specialAction:void
+print():void
The methods of the class are:
dimachaerus: This constructor has a default prole to use when creating a Di-
machaerus gladiator. The statistics that are the default values for the class are
below:
1. HP: 16
2. AS: 0.75
3. DS: 0.15
4. appeal: 10
5. damage: 4
6. seedR: 100
print: This method will print out the statistics of the gladiator in a row by row
fashion. For example:
HP: 16
AS: 0.65
DS: 0.55
Appeal: 1
Damage: 4
SeedR: 100
dimachaerus: This is the destructor for the class. It will print "Dimachaerus
removed" without the quotation marks and with a new line at the end.
taunt: This method will return a unique message. The message is "MARS WILL
HAVE YOUR HEAD!", without quotations.
defenceBolster: This bolster will increase his DS by 0.01 and his AS by 0.01. How-
ever it will decrease his appeal by 1.
specialAction: When activated, the Dimachaerus will drop his appeal by 1 to gain
more 2 HP.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
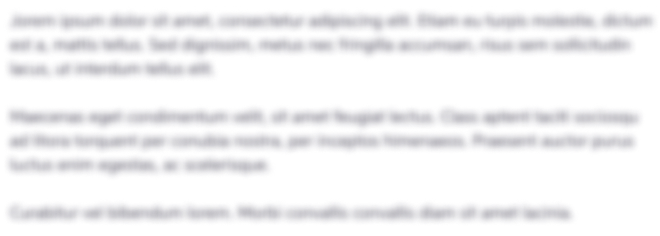
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started