Question
Write c++ program of Wordle Reload by creating a wordleword text file. For this program you can choose either to play a three letter or
Write c++ program of Wordle Reload by creating a wordleword text file.
For this program you can choose either to play a three letter or five letter Wordle board game. The Wordle text file consist of sorted three and five letter words.
Indicators will be given if characters of the user-entered word are reflected in the guessed word. Example 3-letter word: fan
If the character is in the correct position, the character will display as an uppercase value.
Example:
Random word: fan
Guessed word: sad
Output: [ * ] [ A ] [ * ]
If the character is within the random word, the character will display as a lowercase value.
Example:
Random word: fan
Guessed word: net
Output: [ n ] [ * ] [ * ]
If you enter a character that is not in the word, an asterisk '*' will display.
Example:
Random word: fan
Guessed word: tic
Output: [ * ] [ * ] [ * ]
Starter Code, main.cpp
#include
#include
#include
#include
#include
#include
// Display name and program information
void displayIdentifyingInformation() {
cout << " \n"
<< "Program 3: Wordle Reload \n"
<< "CS 141, Spring 2022, UIC \n"
<< endl;
cout << "The objective of this game is to guess the randomly selected word within a given number of attempts. You can select either a three or five word board. \n"
<< "At the conclusion of the game, stats will be displayed. \n"
<< "Indicators will be given if characters of the user entered word are reflected in the guessed word. \n"
<< "- If the character is in the correct position, the character will display as an uppercase value. \n"
<< "- If the character is within the random word, the character will display as a lowercase value. \n"
<< "- If you enter a character that is not in the word, an asterisk '*' will display. \n"
<< endl;
}//end displayIdentifyingInformation()
void readWordsIntoWordlewords(
vector
char fileName[]) {
ifstream inStream;
inStream.open( fileName);
assert( inStream.fail() == false );
wordlewords.clear(); // Clear vector in case it already had some words in it
// Keep repeating while input from the file yields a word
string newWord; // Store a single input word
while( inStream >> newWord) { // While there is another word to be read
// Add this new word to the end of the vector, growing it in the process
wordlewords.push_back( newWord);
}
inStream.close(); // Close the wordlewords file
} //end readWordsIntoWordlewords()
int main() {
displayIdentifyingInformation;
int menuChoice;
vector
// Read in words from the wordlewords words files into vectors.
readWordsIntoWordlewords( wordlewords, "wordlewords.txt");
// Keep looping until user selects the option to exit
while( true) {
cout << endl
<< "Select a menu option:" << endl
<< " 1. To play Wordle Reload 3 letter play" << endl
<< " 2. To play Wordle Reload 5 letter play" << endl
<< " 3. Exit the program" << endl
<< "Your choice --> ";
cin >> menuChoice;
cout << endl;
// Dictionary will store commonly misspelled words
if( menuChoice == 1) {
cout << "To get started, enter your first 3 letter word." << endl;
cout << "You have 4 attempts to guess the random word." << endl;
cout << "The timer will start after your first word entry." << endl;
cout << "Try to guess the word within 20 seconds." << endl;
cout << endl;
cout << "Please enter word --> " << endl;
}
else if( menuChoice == 2) {
cout << "To get started, enter your first 5 letter word." << endl;
cout << "You have 6 attempts to guess the random word." << endl;
cout << "The timer will start after your first word entry." << endl;
cout << "Try to guess the word within 40 seconds." << endl;
cout << endl;
cout << "Please enter word --> " << endl;
} else if( menuChoice == 3) { // Exit if option 3 to exit was chosen
cout << "Overall Stats:" << endl;
cout << "- You guessed: " << endl;
cout << "- Your longest streak is: " << endl;
cout << "- Average word completion time: " << endl;
cout << "Exiting program" << endl;
break; // Break out of enclosing infinite game-play loop
}
} //end while( true)
return 0;
}//end main()
Output
Successful 3 letter word completion and exit Program 3: Wordle Reload CS 141, Spring 2022, UIC The objective of this game is to guess the randomly selected word within a given number of attempts. You can select either a three- or five-word board. At the conclusion of the game, stats will be displayed. Indicators will be given if characters of the user entered word are reflected in the guessed word. -If the character is in the correct position, the character will display as an uppercase value. -If the character is within the random word, the character will display as a lowercase value. - If you enter a character that is not in the word, an asterisk will display. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice -->1 To get started, enter your first 3 letter word. You have 4 attempts to guess the random word. The timer will start after your first word entry. Try to guess the word within 20 seconds. Please enter word--> are [A] [*][*] Please enter word --> ant [A] [*] [*] [A] [N] [*] Please enter word --> and [A] [*] [*] [A] [N] [*] [A] [N] [D] Nice Work! You guessed the correct word - You completed the board in: 5 seconds. - It took you 3/4 attempts. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice -->3 Overall Stats: - You guessed: 1 - Your longest streak is: 1 - Average word completion time: 5 Exiting program 51 6 I Voice Sensitivity Editor Re Successful 5-letter word completion and exit Program 3: Wordle Reload CS 141, Spring 2022, UIC The objective of this game is to guess the randomly selected word within a given number of attempts. You can select either a three or five word board. At the conclusion of the game, stats will be displayed. Indicators will be given if characters of the user entered word are reflected in the guessed word. -If the character is in the correct position, the character will display as an uppercase value. -If the character is within the random word, the character will display as a lowercase value. - If you enter a character that is not in the word, an asterisk will display. 1 Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice -->2 To get started, enter your first 5 letter word. You have 6 attempts to guess the random word. The timer will start after your first word entry. Try to guess the word within 40 seconds. Please enter word--> birds [b] [] [] [d] [*] Please enter word --> erode [b][*] [*] [d] [*] [] [] [O] [d] [*] Please enter word--> adobo [b][*] [*] [d] [*] [] [] [O] [d] [*] [A] [D] [O] [B] [O] Nice Work! You guessed the correct word - You completed the board in: 17 seconds. - It took you 3/6 attempts. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice -->3 Overall Stats: - You guessed: 1 - Your longest streak is: 1 - Average word completion time: 17 Exiting program I Invalid word entry (Greater than or less than intended word length) and exit Program 3: Wordle Reload CS 141, Spring 2022, UIC The objective of this game is to guess the randomly selected word within a given number of attempts. You can select either a three or five word board. At the conclusion of the game, stats will be displayed. Indicators will be given if characters of the user entered word are reflected in the guessed word. - If the character is in the correct position, the character will display as an uppercase value. -If the character is within the random word, the character will display as a lowercase value. - If you enter a character that is not in the word, an asterisk * will display. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice --> 1 To get started, enter your first 3 letter word. You have 4 attempts to guess the random word. The timer will start after your first word entry. Try to guess the word within 20 seconds. Please enter word --> an Invalid word entry - please enter a word that is 3 characters long. Please enter word --> ants Invalid word entry - please enter a word that is 3 characters long. Please enter word --> and [A] [N] [D] Nice Work! You guessed the correct word. - You completed the board in: 0 seconds. - It took you 1/4 attempts. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play. 3. Exit the program Your choice --> 2 To get started, enter your first 5 letter word. You have 6 attempts to guess the random word. The timer will start after your first word entry. Try to guess the word within 40 seconds. Please enter word --> cold Invalid word entry - please enter a word that is 5 characters long. Please enter word --> working Invalid word entry - please enter a word that is 5 characters long. Please enter word --> crime 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice->1 To get started, enter your first 3 letter word. You have 4 attempts to guess the random word. The timer will start after your first word entry. Try to guess the word within 20 seconds. Please enter word --> an Invalid word entry - please enter a word that is 3 characters long. Please enter word --> ants Invalid word entry - please enter a word that is 3 characters long. Please enter word --> and [A] [N] [D] Nice Work! You guessed the correct word - You completed the board in: 0 seconds. - It took you 1/4 attempts. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice --> 2 To get started, enter your first 5 letter word. You have 6 attempts to guess the random word. The timer will start after your first word entry. Try to guess the word within 40 seconds. Please enter word --> cold Invalid word entry - please enter a word that is 5 characters long. Please enter word --> working Invalid word entry - please enter a word that is 5 characters long. Please enter word --> crime [C] [R] [I] [M] [E] Nice Work! You guessed the correct word - You completed the board in: 0 seconds. - It took you 1/6 attempts. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice -3 Overall Stats: - You guessed: 2 - Your longest streak is: 2 - Average word completion time: 0 Exiting program 1 Divlacn BO E E 3 and 5 letter word unsuccessfully guessed *Notice the completion time is N/A due to not guessing word correctly. Program 3: Wordle Reload CS 141, Spring 2022, UIC The objective of this game is to guess the randomly selected word within a given number of attempts. You can select either a three or five word board. At the conclusion of the game, stats will be displayed. Indicators will be given if characters of the user entered word are reflected in the guessed word. -If the character is in the correct position, the character will display as an uppercase value. -If the character is within the random word, the character will display as a lowercase value. - If you enter a character that is not in the word, an asterisk * will display. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice -->1 To get started, enter your first 3 letter word. You have 4 attempts to guess the random word. The timer will start after your first word entry. Try to guess the word within 20 seconds. Please enter word --> dan [d] [a] [n] Please enter word--> dap [d] [a] [n] [d] [a] [*] Please enter word ant [d] [a] [n] [d][a][*] [A] [N] [*] Please enter word-> any [d][a] [n] [d][a][*] [A] [N] [*] [A] [N] [*] Maximum amount of attempts have been reached. Try again. Select a menu option: 1. To play Wordle Reload 3 letter play: 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice --> 2 To get started, enter your first 5 letter word. You have 6 attempts to guess the random word. The timer will start after your first word entry. User entered word is not found in list of words (Binary Search to check list for word) Program 3: Wordle Reload CS 141, Spring 2022, UIC The objective of this game is to guess the randomly selected word within a given number of attempts. You can select either a three or five word board. At the conclusion of the game, stats will be displayed. Indicators will be given if characters of the user entered word are reflected in the guessed word. -If the character is in the correct position, the character will display as an uppercase value. -If the character is within the random word, the character will display as a lowercase value. - If you enter a character that is not in the word, an asterisk * will display. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice 1 To get started, enter your first 3 letter word. You have 4 attempts to guess the random word. The timer will start after your first word entry. Try to guess the word within 20 seconds. Please enter word--> a38 Not a playable word, please select another word. Please enter word--> tac Not a playable word, please select another word. Please enter word --> won [] [] [n] Please enter word --> and [*] [*] [n] [A] [N] [D] Nice Work! You guessed the correct word - You completed the board in: 6 seconds. -It took you 2/4 attempts. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice --> 3 Overall Stats: - You guessed: 1 - Your longest streak is: 1 - Average word completion time: 6 Exiting program 23 U ok n He DO q 3700 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice --> 2 To get started, enter your first 5 letter word. You have 6 attempts to guess the random word. The timer will start after your first word entry. Try to guess the word within 40 seconds. Please enter word --> erode [e] [R] [*] [*] [E] Please enter word --> prime [e] [R] [*] [*] [E] [] [R] [1] [M] [E] Please enter word --> found [e] [R] [*] [*] [E] [][R] [I] [M] [E] [*] [*] [*] [*] [*] Please enter word --> sound [e] [R] [*] [*] [E] [] [R] [1] [M] [E] [*] [*] [*] [*] [*] [*] [*] [*] [*] [*] Please enter word--> slope [e] [R] [*] [*] [E] [] [R] [1] [M] [E] [*] [*] [*] [*] [*] [*] [*] [*] [*] [*] [][*] [*] [*] [E] Please enter word --> chime [e] [R] [*] [*] [E] [] [R] [I] [M] [E] [*] [*] [*] [*] [*] [*] [*] [*] [*] [*] [*] [*] [*] [*] [E] [C] [*] [I] [M] [E] Maximum amount of attempts have been reached. Try again. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice --> 3 Overall Stats: - You guessed: 0. - Your longest streak is: 0 - Average word completion time: N/A Exiting program English Cataal Test Dredictions SA THE TA Simply Exiting the program Program 3: Wordle Reload CS 141, Spring 2022, UIC The objective of this game is to guess the randomly selected word within a given number of attempts. You can select either a three or five word board. At the conclusion of the game, stats will be displayed. Indicators will be given if characters of the user entered word are reflected in the guessed word. -If the character is in the correct position, the character will display as an uppercase value. -If the character is within the random word, the character will display as a lowercase value. - If you enter a character that is not in the word, an asterisk ** will display. Select a menu option: 1. To play Wordle Reload 3 letter play 2. To play Wordle Reload 5 letter play 3. Exit the program Your choice -3 Overall Stats: - You guessed: 0 Your longest streak is: 0 - Average word completion time: N/A Exiting program H
Step by Step Solution
3.19 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
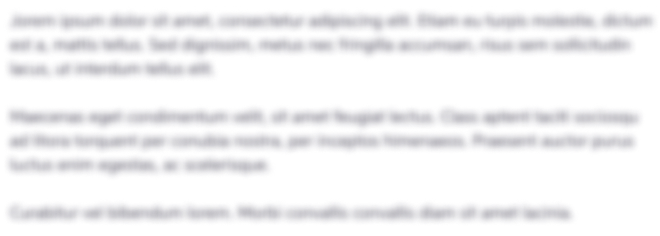
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started