Question
Write C# program to evaluate odd/even values passing through processes in ring topology ? Random number generator code is given below. Concurrent two leader election
Write C# program to evaluate odd/even values passing through processes in ring topology ? Random number generator code is given below.
Concurrent two leader election algorithm:
Process 0 sends its computer value to the left neighbor (clockwise). That value can be even or odd. If the value is even, then process 0 adds a second value of 1; if the value is odd, then process 0 adds a second value of 0. The two values are sent to process 1.
On receiving the value, process 1 compares:
-
its value with the odd one if it has an odd value or
-
its value with the even one, if it has an even value.
The largest value and the other unchanged value are sent further to process 2. Process 2 does the same check. The two values received at process 0 will be the largest even and odd generated values in the ring and will be declared by process 0 as the two leaders.
Election of the even leader:
If process 0 has an even computer value, it sends to process 1; if process 1 has an odd computed value then it sends the value of 0 to process 1. On receiving the value, if process 1 has:
- also an even value, it compares the received value with its own and the largest among the two is sent further to process 2
- an odd value, then the value is sent further to process 2.
Process 2 does the same comparison and sends the result to process 3, etc. The value received at process 0 is declared as the even leader.
Election of the odd leader:
If process 0 has an odd computer value, it sends to process 1; if process 1 has an even computed value then it sends the value of 1 to process 1. On receiving the value, if process 1 has:
- also an odd value, it compares the received value with its own and the largest among the two is sent further to process 2
- an even value, then the value is sent further to process 2.
Process 2 does the same comparison and sends the result to process 3, etc. The value received at process 0 is declared as the odd leader.
What to do
1. Write clear pseudocode for each algorithm, describe what parameters are needed to execute the program and submit it as a PDF report.
2. Implement your algorithm in C/C++ using MPI primitives
3. Compile and execute the program.
4. Submit the source code
#include
#include
#include
#include
#include
int main(int argc, char *argv[]) {
int rank, size;
MPI_Init( &argc, &argv );
MPI_Comm_rank( MPI_COMM_WORLD, &rank );
MPI_Comm_size( MPI_COMM_WORLD, &size );
srand(time(NULL) * rank);
int rand_num;
rand_num = (rand() % 100) + 1;
printf("Process %d generated number: %d ",rank, rand_num);
int local_sum = 0; #pragma omp parallel for schedule(static, 1)
for (int a = 0; a < rand_num; a++) {
local_sum += a;
}
printf("Process %d generated local sum: %d ", rank, local_sum);
MPI_Finalize();
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
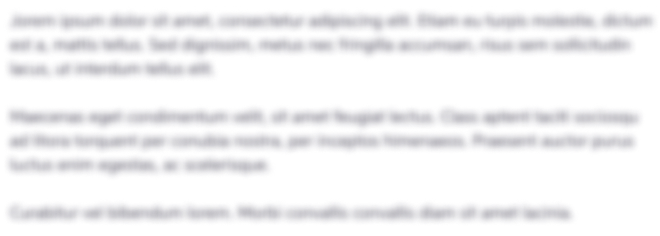
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started