Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Write C programs named matrix.h and matrix.c containing the headers and implementations of following functions. void magic_square(int *m, int n) generates an n by n
Write C programs named matrix.h and matrix.c containing the headers and implementations of following functions.
- void magic_square(int *m, int n) generates an n by n magic square and stores it in 2-D array passed by pinter m (only n=3 is required).
- void display_matrix(int *m, n) displays n by n matrix m in 2-D style.
- int is_magic_square(int *m, int n) checks if the given matrix m is a magic square, returns 1 if true otherwise 0.
- void transpose_matrix(int *m1, int *m2, int n) transposes the n by n matrix m1 and saves the resulted matrix in m2.
- void multiply_matrix(int *m1, int* m2, int *m3 int n) computes the matrix multiplication m1*m2 and saves the resulted matrix in m3.
matrix.h
#include
#include
void magic_square(int *m, int n);
int is_magic_square(int *m, int n);
void display_matrix(int *m, int n);
void transpose_matrix(int *m1, int *m2, int n);
void multiply_matrix(int *m1, int *m2, int *m3, int n);
matrix.c
//---------------------------------------------------------
void magic_square(int *m, int n) {
/* assign 3X3 matrix to following values
8 1 6
3 5 7
4 9 2
*/
int values[9] = { 8, 1, 6, 3, 5, 7, 4, 9, 2 };
int i, len = n*n, *p = values;
for (i = 0; i < len; i++) *m++ = *p++;
}
//---------------------------------------------------------
void display_matrix(int *m, int n) {
int *p = m, i, j;
for (i = 0; i < n; i++) {
printf(" ");
for (j = 0; j < n; j++) printf("%4d", *p++);
}
printf(" ");
}
//---------------------------------------------------------
int is_magic_square(int *m, int n) {
// your implementation
}
//---------------------------------------------------------
void transpose_matrix(int *m1, int *m2, int n) {
// your implementation
}
//---------------------------------------------------------
void multiply_matrix(int *m1, int *m2, int *m3, int n) {
// your implementation
}
Use the provided main program to test the above functions
#include "matrix.h"
int main() {
int n = 3;
int m1[n][n];
int m2[n][n];
int m3[n][n];
int *p1 = &m1[0][0];
magic_square(p1, n);
printf(" m1:");
display_matrix(p1, n);
printf("is_magic_square:%d ", is_magic_square(p1, n));
int *p2 = &m2[0][0];
printf(" m1':");
transpose_matrix(p1, p2, n);
display_matrix(p2, n);
printf("is_magic_square:%d ", is_magic_square(p2, n));
int *p3 = &m3[0][0];
multiply_matrix(p1, p2, p3, n);
printf(" m1*m1':");
display_matrix(p3, n);
printf("is_magic_square:%d ", is_magic_square(p3, n));
return 0;
}
the output is like the following.
m1:
8 1 6
3 5 7
4 9 2
is_magic_square:1
m1':
8 3 4
1 5 9
6 7 2
is_magic_square:1
m1*m1':
101 71 53
71 83 71
53 71 101
is_magic_square:0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
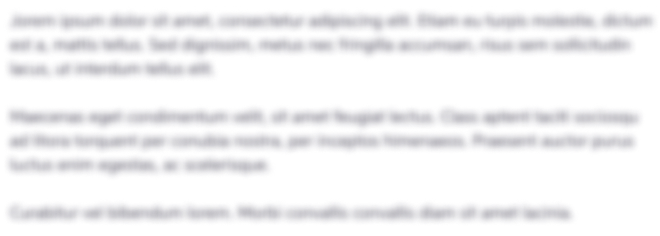
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started