Question
WRITE CODE IN C PLEASE ------------------------------------------ floatStuff.c ------------------------------------------ #include #include #include #include #define NORM 0 #define DNORM 1 #define SPEC 2 #define BIAS 127 /*
WRITE CODE IN C PLEASE
------------------------------------------
floatStuff.c
------------------------------------------
#include
#define NORM 0 #define DNORM 1 #define SPEC 2 #define BIAS 127
/* Declare a "typedef struct {} flt;" to contain data for a float The data should include: An integer for the sign (1 for positive, -1 for negative) An integer for the exponent value (should be bit value to integer minus BIAS or -126 for denormalized) A float to contain the value of the mantissa (Actual float value extracted from the binary value) An integer to contain the mode using the defines above (NORM, DNORM and SPEC) Example after processing: -15.375 = 1 10000010 11101100000000000000000 sign = -1 exp = 3 man = 0.9218750000 mode = NORM */
/* Write a function get_flt_bits_int to return an integer with the bits copied from a float. Example: for f = -15.375, the bits of int n = 11000001011101100000000000000000 Look at the slides and code from the float lectures and use the indirection trick. This can easily be done in one line of code. The function should accept a float and return an int. */
/* Write a function that returns the sign of a float as a char. You should call get_flt_bits_int to get the bits in an int and return '1' if the sign is negative else return '0'. The function should accept a float and return a string. */
/* Write a function that returns the sign of a float as an integer. You should call get_flt_bits_int to get the bits in an int and return -1 if the sign is negative else return 1. The function should accept a float and return an int. */
/* Write a function to return a string containing the actual binary value of the exponent of a float in a char array. You should call get_flt_bits_int to get the bits in an int and return the string. Example: for f = -15.375 n = 11000001011101100000000000000000 the exponent bits are "10000010" The function should accept a float and return a string. */
/* Write a function to return an integer containing the actual integer value of the exponent of a float. You should call get_flt_bits_int to get the bits in an int and return the int with the exponent value. Example: for f = -15.375 n = 11000001011101100000000000000000 the exponent bits are 10000010 the actual value of the exponent is 3 The function should accept a float and return an int. */
/* Write a function to return an integer containing the mode of the exponent of a float. You should call get_flt_exp_val to get the bits in an int and return the int with the mode value. Example: for f = -15.375 n = 11000001011101100000000000000000 the exponent bits are 10000010 the mode is NORM The function should accept a float and return an int. */
/* Write a function to return a string containing the actual binary value of the mantissa of a float in a char array. You should call get_flt_bits_int to get the bits in an int and return the string. Example: for f = -15.375 n = 11000001011101100000000000000000 the mantissa bits are "11101100000000000000000" The function should accept a float and return a string. */
/* Write a function to return a float containing the actual float value of the mantissa of a float. You should call get_flt_bits_int to get the bits in an int and return the int with the mantissa value. Example: for f = -15.375 n = 11000001011101100000000000000000 the mantissa bits are 11101100000000000000000 the actual value of the mantissa is 0.9218750000 The function should accept a float and return an int. */
/* Write a function to return a string containing the actual binary value of a float in a char array. You should call get_flt_sign_char, get_flt_exp_str and get_flt_man_str to get the bits in an char and two strings and return the concatenated string. Example: for f = -15.375 n = 11000001011101100000000000000000 The sign is '1' the exponent is "10000010" and the mantissa bits are "11101100000000000000000" The string should be formatted as: "1 10000010 11101100000000000000000" to clearly separate the 3 parts. The function should accept a float and return a string. */
/* Write a function to separate the parts of a float into a flt struct as described above. You should call get_flt_sign_val, get_flt_exp_mode, get_flt_exp_val and get_flt_man_val. Hint: make sure to set exponent to -126 for DNORM mode. */
/* Write a function to print a flt struct to screen. It should accept a flt struct and return nothing. Hint: Use if statement to print mode. */
/* Write a function to get the actual float value back out of a flt struct. Hints: The float value produced will depend on the mode. To set a float to infinity use the math library constant INFINITY To set a float to not-a-number use the math library constant NAN Check the slides and text for conditions for NORN, DNORM and SPEC You need to return (sign) * M * 2^e */
/* Write a main function that calls an prints results for each function when completed. get_flt_sign_char get_flt_sign_val
get_flt_exp_str get_flt_exp_val
get_flt_man_str get_flt_man_val
get_flt_bits_str
get_flt_val_flt print_flt
get_flt_bits_val */ int main(){
return 0; }
CIS 2107 Lab 6 -Float Stuff The purpose of this lab is to practice manipulating 32-bit floating point numbers. You will write code to extract both the bit value and, numeric values of a float. The code required is 1. A typedef struct flt for the sign, exponent, mantissa and mode of a float. 2. A main function to test all your functions after completion. 3. A function get flt bits int to get the bits of a float as an int for bitwise manipulation. 4. A function get flt sign_char to get the char value of a float 5. A function get flt sign_val to get the numeric sign of a float. 6. A function get flt exp str to return a string of the bits in the exponent of a float. 7. A function get flt exp val to return the integer value of the exponent in a float with respect to the bias. 8. A function get flt exp_mode to get the mode of a float from the exponent. 9. A function get fit_man_str to get a string containing the bit value of the mantissa in a float. 10. A function get fit man_val to get the float value of the mantissa in a float. 11. A function get flt bits str to return a string with the sign, exponent and mantissa of a float with each part separated by a space 12. A function get flt val fit that converts a float to a flt struct. 13. A function that prints a flt structs data to screen. 14. A function get flt bits val that converts a flt struct back to a float. Download the floatStuff.c file from Canvas. Read the comments about the content of the code in each function. There are lots of hints to help you complete this project. I strongly suggest that you write the functions in order as they appear above because subsequent functions depend on previous functions. Write a function and text it before moving to the next. I included the preprocessor statements to help Note that the #defines can all be interpreted as integer values in code. The main is at the bottom to avoid typing prototypes after the preprocessor statements. Good luck! CIS 2107 Lab 6 -Float Stuff The purpose of this lab is to practice manipulating 32-bit floating point numbers. You will write code to extract both the bit value and, numeric values of a float. The code required is 1. A typedef struct flt for the sign, exponent, mantissa and mode of a float. 2. A main function to test all your functions after completion. 3. A function get flt bits int to get the bits of a float as an int for bitwise manipulation. 4. A function get flt sign_char to get the char value of a float 5. A function get flt sign_val to get the numeric sign of a float. 6. A function get flt exp str to return a string of the bits in the exponent of a float. 7. A function get flt exp val to return the integer value of the exponent in a float with respect to the bias. 8. A function get flt exp_mode to get the mode of a float from the exponent. 9. A function get fit_man_str to get a string containing the bit value of the mantissa in a float. 10. A function get fit man_val to get the float value of the mantissa in a float. 11. A function get flt bits str to return a string with the sign, exponent and mantissa of a float with each part separated by a space 12. A function get flt val fit that converts a float to a flt struct. 13. A function that prints a flt structs data to screen. 14. A function get flt bits val that converts a flt struct back to a float. Download the floatStuff.c file from Canvas. Read the comments about the content of the code in each function. There are lots of hints to help you complete this project. I strongly suggest that you write the functions in order as they appear above because subsequent functions depend on previous functions. Write a function and text it before moving to the next. I included the preprocessor statements to help Note that the #defines can all be interpreted as integer values in code. The main is at the bottom to avoid typing prototypes after the preprocessor statements. Good luckStep by Step Solution
There are 3 Steps involved in it
Step: 1
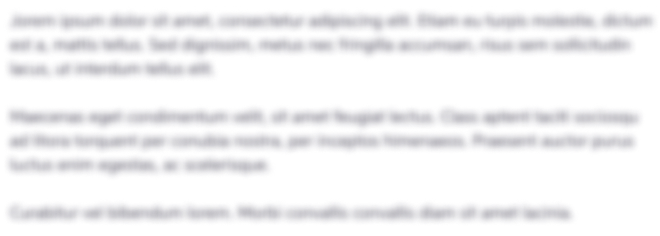
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started