Question
Write Java programs that use command-line input and call the following integer functions. All calculations for each function should be encapsulated within the function. Use
Write Java programs that use command-line input and call the following integer functions. All calculations for each function should be encapsulated within the function. Use a long datatype instead of int for all input and output variables. Do NOT use string variables in any of the algorithms.
1. SumOfDivisors: The input variable X is a positive integer. The function should return as Y the sum of the divisors of X. For example, if X is 10, then Y is 1+2+5+10=18. If X is 23, then Y is 24. Whenever Y = 2X, X is called a perfect number. Test your function with the following inputs:
X = 6 X = 277 X = 3443 X = 8128 X = 298760
2. ReverseDigits: The input variable X is a positive integer. The function should return as Y an integer containing the digits of X in reverse order. Leading zeros do not appear in X or Y. For example, if X is 2645, the Y is 5462. If X is 2400, then Y is 42. If Y = X, then X is called a palindrome (symmetric). Test your function with the following inputs:
X = 45600 X = 62826 X = 157910 X = 483047 X = 901108
3. GCD: The function has two input variables X1 and X2, which are positive integers. The function should return as Y the greatest common divisor (GCD) of X1 and X2, calculated using theEuclidean algorithm (non-recursive version). Test your function with the following inputs:
X1 = 136 X2 = 120
X1 = 512 X2 = 2217
X1 = 1595 X2 = 4255
X1 = 18684 X2 = 49878
X1 = 237732 X2 = 819918
4. BaseB: The function has two integer input values X and B, with variable X between 1 and 100,000 and base B between 2 and 10. The function should return as Y the value of X expressed as a base B number. Test your function with the following inputs:
X = 359 B = 2
X = 632 B = 3
X = 757 B = 5
X = 981 B = 8
X = 8046 B = 10
5.RecursiveSeq: The input variable X is an integer between 1 and 50. The function should return as Y the X-th term of a sequence defined recursively by:
f(1) = 1
f(2) = 3
f(X) = 2*f(X-1)2*f(X-2) for X = 3,4,5,...
Your function code should use recursion(not a loop). Test your function with the following inputs:
X = 2 X = 10 X = 24 X= 33 X = 40
For each of the above functions, state whether or not the function is one-to-one. Justify youranswers. If a function is not one-to-one, show two inputs that have the same output
This is the beginning of one:
// Program to calculate the sum of the divisors // of a positive integer. public class P1PartB1 { pulblic static void main (String args[]) { long X, Y; if(args.length >=1) { x = Long.parseLong(args[0]); if(X >=1){ System.out.println(" X = " + X + " Y = " + Y); } } return; } public static long SumOfDivisors(long x){ long y = 0; // ...put code here...
return y;
} } // end class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
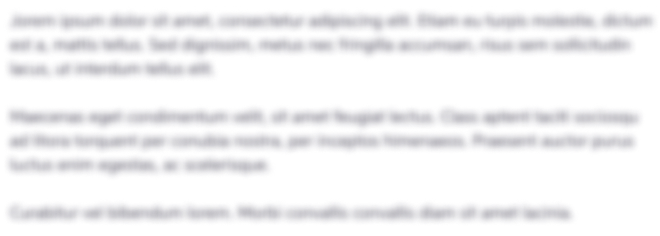
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started