Question
Write junit test cases that EXECUTES AND TEST EVERY LINE of the code provided below: package edu.buffalo.cse116; /** * Calculate the resistance of a circuit.
Write junit test cases that EXECUTES AND TEST EVERY LINE of the code provided below:
package edu.buffalo.cse116;
/** * Calculate the resistance of a circuit. This is important for computer engineers and professors needing good code * which makes students write lots of test cases. * * @author Matthew Hertz */ public class CircuitResistance { /** * Calculate the resistance of a circuit which includes three parallel resistors. According to Wikipedia, circuit * resistance when the resistors are parallel is calculated as R^-1 = R1^-1 + R2^-1 + R3^-1. * * @see Wikipedia article inspiring this * problem * @param r1 Resistance provided by the first parallel component of the circuit. * @param r2 Resistance provided by the second parallel component of the circuit. * @param r3 Resistance provided by the third parallel component of the circuit. * @return The overall resistance of the circuit. {@link Double#NaN} is returned when an impossible resistance is * supplied. Despite having worked for a company which developed computers, the author's understanding of what * this means is shaky. */ public static double resistorsInParallel(double r1, double r2, double r3) { if (r1 == 0) { return Double.NaN; } if (r2 == 0) { return Double.NaN; } if (r3 == 0) { return Double.NaN; } double inverseResist = (1 / r1) + (1 / r2) + (1 / r3); return 1 / inverseResist; }
/** * Calculate the resistance of a circuit which includes three resistors one after the other. According to Wikipedia, * circuit resistance when the resistors are serial is calculated as R^ = R1 + R2 + R3. * * @see Wikipedia article inspiring this * problem * @param r1 Resistance provided by the first parallel component of the circuit. * @param r2 Resistance provided by the second parallel component of the circuit. * @param r3 Resistance provided by the third parallel component of the circuit. * @return The overall resistance of the circuit. {@link Double#NaN} is returned when an impossible resistance is * supplied. The author has a better understanding how this equation works. */ public static double resistorsInSerial(double r1, double r2, double r3) { // Because Java uses "short-circuited" boolean operators, it executes the single if statement below as if it were // written like the 3 if statements above. if ((r1 == 0) || (r2 == 0) || (r3 == 0)) { return Double.NaN; } double resist = r1 + r2 + r3; return resist; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
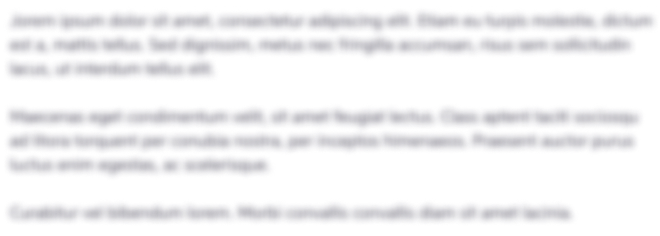
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started