Question
Write the class VendingMachine that will represent a typical vending machine (item number, price, stock). An instance of VendingMachine has access to five methods, newStock
Write the class VendingMachine that will represent a typical vending machine (item
number, price, stock). An instance of VendingMachine has access to five methods, newStock,
getStock, purchase, deposit and restock that will describe its interaction with the user returning
strings. This vending machine does not give you money back if you dont make a purchase. Tip:
use the string format method to construct the strings
Vending Machine notes:
When creating an instance of this class, the machine is empty, thus, calling getStock, purchase
and deposit simply return out of stock messages:
>>> x=VendingMachine()
>>> x.purchase(215)
'Machine out of stock'
>>> x.deposit(10)
'Sorry, out of stock. Take your $10 back'
>>> x.getStock()
'Machine out of stock'
>>> x.restock(215, 9)
'Invalid item'
newStock is used only when the registering a new item. If an item is out of stock or still has
stock, using newStock will return a message to the user. For already registered items, you use
the restock method. Restock returns an error when the item is not in the dictionary
>>> x.newStock(215,2.50,2)
'Current 215 stock: 2' >>> x.restock(215, 1)
'Current item stock: 3'
>>> x.restock(5, 1)
'Invalid item'
>>> x.getStock()
{215: [2.5, 3]}
# User deposits 7.5 and purchases 3 items of 215
>>> x.getStock()
{215: [2.5, 0]}
>>> x.newStock(215,2.50,2)
'Item already registered'
>>> x.newStock(156,3,3)
'Current 156 stock: 3'
>>> x.getStock()
{215: [2.5, 0], 156: [3, 3]}
If all products are out of stock, deposit returns an out of stock message
>>> x.getStock()
{215: [2.5, 0], 156: [3, 0]}
>>> x.deposit(10)
'Machine out of stock. Take your $10 back'
purchase uses quantity=1 as a default value, which can be overwritten by providing the second
argument. Before completing a purchase, machine has to check if the item is valid, if it is on
stock and if the user has enough balance.
>>> x.getStock()
{215: [3, 1], 156: [3.5, 4]}
>>> x.purchase(56)
'Invalid item'
>>> x.purchase(215)
'Please deposit $3'
>>> x.deposit(1)
>>> x.purchase(215)
'Please deposit $2'
>>> x.deposit(10)
>>> x.purchase(215)
'Item dispensed, take your $5'
>>> x.purchase(215)
'Item out of stock'
>>> x.getStock()
{215: [3, 0], 156: [3.5, 4]}
getStock returns a dictionary where the key is the item and the value is the list [price, stock]
purchase will ask for the needed money to complete a purchase if enough items are in stock,
otherwise, it returns the current stock of the item (for example 3 items in stock and attempting
to purchase 4)
Once the user completes one purchase, machine returns remaining balance
For more examples of functionality, check the doctest in the starter code
Quotes mean method returned a string, no need to append them in your code
Return output with the sentences provided. Solution is not case sensitive, which means
Balance: $10 is the same as balance: $10, but is not the same as Balance= $10
class VendingMachine: ''' >>> x=VendingMachine() >>> x.purchase(215) 'Machine out of stock' >>> x.deposit(10) 'Sorry, out of stock. Take your $10 back' >>> x.restock(215, 9) 'Invalid item' >>> x.newStock(215,2.50,9) 'Current 215 stock: 9' >>> x.restock(215, 16) 'Current item stock: 25' >>> x.newStock(156,3,3) 'Current 156 stock: 3' >>> x.getStock() {215: [2.5, 25], 156: [3, 3]} >>> x.purchase(156) 'Please deposit $3' >>> x.purchase(156, 2) 'Please deposit $6' >>> x.purchase(156, 4) 'Current 156 stock: 3' >>> x.deposit(2) 'Balance: $2' >>> x.purchase(156) 'Please deposit $1' >>> x.purchase(156, 2) 'Please deposit $4' >>> x.deposit(5) 'Balance: $7' >>> x.purchase(156) 'Item dispensed, take your $4' >>> x.getStock() {215: [2.5, 25], 156: [3, 2]} >>> x.purchase(156) 'Please deposit $3' >>> x.deposit(6) 'Balance: $6' >>> x.purchase(156,2) 'Item dispensed' >>> x.purchase(156) 'Item out of stock' >>> x.deposit(62.5) 'Balance: $62.5' >>> x.purchase(215,25) 'Item dispensed' >>> x.deposit(6) 'Machine out of stock. Take your $6 back' >>> x.newStock(156,3,3) 'Item already registered' >>> x.restock(85, 10) 'Invalid item' ''' def __init__(self): #--- YOUR CODE STARTS HERE self.
def purchase(self, item, qty=1): #--- YOUR CODE STARTS HERE self.item_
def deposit(self, amount): #--- YOUR CODE STARTS HERE
def restock(self, item, stock): #--- YOUR CODE STARTS HERE
def newStock(self, item, price, stock): #--- YOUR CODE STARTS HERE
def getStock(self): #--- YOUR CODE STARTS HERE
Step by Step Solution
There are 3 Steps involved in it
Step: 1
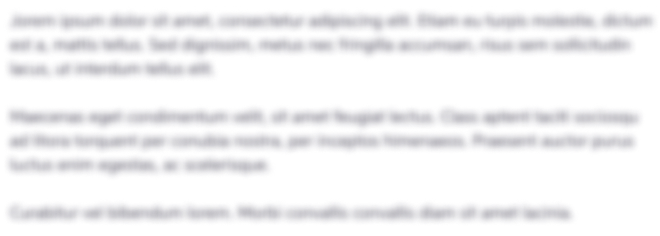
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started