Question
Write the definition of the linkedListKeepLast function. (Please write the following in C, Thank you) The purpose of the function is to create a new
Write the definition of the linkedListKeepLast function. (Please write the following in C, Thank you)
The purpose of the function is to create a new linked list containing
the last node in each linked list in the hash table beginning with the first linked list.
The rest of the nodes are to be displayed to the screen, then returned to the heap.
Example:
If the user enters the following keys:
201, 102, 233, 567, 456, 654, 465, 645, quit
list at index 0 is empty
list at index 1 is not empty:
201
102
567
list at index 2 is not empty:
233
list at index 3 is empty
list at index 4 is not empty:
456
654
465
645
Deleted nodes:
--> at index 0:
--> at index 1: 201 102
--> at index 2:
--> at index 3:
--> at index 4: 456 654 465
The final linked list contains:
567
233
645
Written by:
*/
#include
#include
#include
#define NUMPOINTERS 5
typedef struct node STUDENTREC;
struct node
{
char id[10];
struct node *next;
};
// Function Declarations
int hash(char id[]);
STUDENTREC *insert(char id[],
STUDENTREC *student_body[],
int hashval);
void traverse(STUDENTREC *student_body[]);
void displayLL(STUDENTREC *list, char *description);
STUDENTREC *linkedListKeepLast(STUDENTREC *student_body[]);
int main (void)
{
STUDENTREC *student_body[NUMPOINTERS] = {NULL};
STUDENTREC *person;
STUDENTREC *endList;
char id[10];
int hashval;
printf(" ~*~ Hashing using collision resolution by chaining ~*~ ");
printf("\t Enter Student ID (or quit): ");
scanf("%s", id);
while(strcmp(id, "quit"))
{
hashval = hash(id);
person = insert(id, student_body, hashval);
if (person) // not NULL => duplicate
{
printf("Duplicate record! ");
}
printf("\t Enter Student ID (or quit): ");
scanf("%s", id);
}
traverse(student_body);
endList = linkedListKeepLast(student_body);
displayLL(endList, "New List");
traverse(student_body);
return 0;
}
/*
The purpose of the function is to create a new linked list containing
the last node in each linked list in the hash table beginning with the first linked list.
The rest of the nodes are to be displayed to the screen, then returned to the heap.
*/
STUDENTREC *linkedListKeepLast(STUDENTREC *student_body[])
{
STUDENTREC *newList = NULL;
/* *********************************************************
Write your code here
Get the job done without calling other linked list functions
********************************************************* */
return newList;
}
/***************************************************
Hash Student ID by summing the cubes
of the ASCII value of characters and then take
the modulo of this sum.
*/
int hash(char id[])
{
long sum = 0;
while (*id) // != '\0'
{
sum += *id * *id * *id;
id++;
}
return sum % NUMPOINTERS;
}
/***************************************************
Insert a new Social Security number into the
array of student records, at index equal to
hashvalue
*/
STUDENTREC *insert(char id[],
STUDENTREC *student_body[],
int hashval)
{
STUDENTREC **mover; // Use ** to write elegant code
mover = &student_body[hashval];
while (*mover)
{
if (strcmp(id,(*mover)->id) == 0) return *mover;
mover = &((*mover)->next);
}
if ((*mover = (STUDENTREC *) malloc(sizeof(STUDENTREC))) == NULL)
{
printf("Malloc error in insert! ");
exit(1);
}
strcpy((*mover)->id, id);
(*mover)->next = NULL; // set the link of the new node to NULL
printf("%s has been placed in the list at location %d. ", (*mover)->id, hashval);
return NULL;
}
/***************************************************
Traversing the lists in a hash table
*/
void traverse(STUDENTREC *student_body[])
{
int i;
// STUDENTREC **mover; // Use ** for fun and practice
// not needed for traverse
for (i = 0; i < NUMPOINTERS; i++)
{
printf("Contents of list %2d ", i);
printf("-------------------- ");
// for (mover = &student_body[i]; *mover; mover = &(*mover)->next)
// { // &((*mover)->next)
// printf("%s ", (*mover)->ss);
// }
displayLL(student_body[i], NULL);
printf(" ");
}
}
/***************************************************
Traversing a linked list
*/
void displayLL(STUDENTREC *list, char *description)
{
if (list) // != NULL
{
if (description) // != NULL
{
printf ("%s ", description);
printf("-------------------- ");
}
STUDENTREC **mover; // Use ** for fun and practice
// not needed for traverse
for (mover = &list; *mover; mover = &(*mover)->next)
{ // &((*mover)->next)
printf("%s ", (*mover)->id);
}
}
else
printf ("Empty!");
printf(" ");
}
/*
~*~ Hashing using collision resolution by chaining ~*~
Enter Student ID (or quit): 201
201 has been placed in the list at location 1.
Enter Student ID (or quit): 102
102 has been placed in the list at location 1.
Enter Student ID (or quit): 233
233 has been placed in the list at location 2.
Enter Student ID (or quit): 567
567 has been placed in the list at location 1.
Enter Student ID (or quit): 456
456 has been placed in the list at location 4.
Enter Student ID (or quit): 654
654 has been placed in the list at location 4.
Enter Student ID (or quit): 465
465 has been placed in the list at location 4.
Enter Student ID (or quit): 645
645 has been placed in the list at location 4.
Enter Student ID (or quit): quit
Contents of list 0
--------------------
Empty!
Contents of list 1
--------------------
201
102
567
Contents of list 2
--------------------
233
Contents of list 3
--------------------
Empty!
Contents of list 4
--------------------
456
654
465
645
Deleted nodes:
--> at index 0:
--> at index 1: 201 102
--> at index 2:
--> at index 3:
--> at index 4: 456 654 465
New List
--------------------
567
233
645
Contents of list 0
--------------------
Empty!
Contents of list 1
--------------------
Empty!
Contents of list 2
--------------------
Empty!
Contents of list 3
--------------------
Empty!
Contents of list 4
--------------------
Empty!
*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
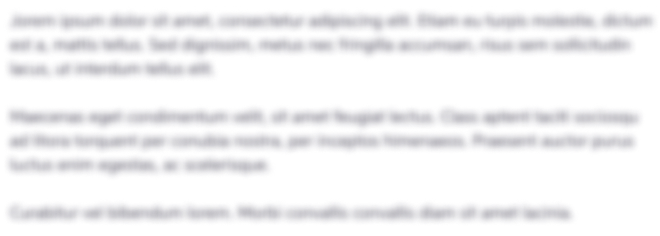
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started