Question
Write the definitions of the function processVotes and printResults of the Programming Example Election Results. (where it says see programming exercise 13). #include #include #include
Write the definitions of the function processVotes and printResults of the Programming Example Election Results. (where it says "see programming exercise 13).
#include
#include
#include
#include "candidateType.h"
#include "orderedArrayListType.h"
using namespace std;
const int NO_OF_CANDIDATES = 6;
void fillNames(ifstream& inFile,
orderedArrayListType
void processVotes(ifstream& inFile,
orderedArrayListType
void addVotes(orderedArrayListType
void printHeading();
void printResults(orderedArrayListType
int main()
{
orderedArrayListType
ifstream inFile;
inFile.open("candData.txt");
fillNames(inFile, candidateList);
candidateList.selectionSort();
inFile.close();
inFile.open("voteData.txt");
processVotes(inFile, candidateList);
addVotes(candidateList);
printHeading();
printResults(candidateList);
return 0;
}
void fillNames(ifstream& inFile,
orderedArrayListType
candidateType temp;
for (int i = 0; i < NO_OF_CANDIDATES; i++)
{
inFile >> firstN >> lastN;
temp.setName(firstN, lastN);
cList.insertAt(i, temp);
}
}
void processVotes(ifstream& inFile,
orderedArrayListType
{
cout << "See Programming Exercise 13" << endl;
}
void addVotes(orderedArrayListType
{
candidateType temp;
for (int i = 0; i < NO_OF_CANDIDATES; i++)
{
cList.retrieveAt(i, temp);
temp.calculateTotalVotes();
cList.replaceAt(i, temp);
}
}
void printHeading()
{
cout << " --------------------Election Results---------"
<< "-----------" << endl << endl;
cout << " Votes" << endl;
cout << " Candidate Name Region1 Region2 Region3 "
<<"Region4 Total" << endl;
cout << "--------------------- ------- ------- "
<< "------- ------- ------" << endl;
}
void printResults(orderedArrayListType
{
cout << "See Programming Exercise 13" << endl;
}
Files for reference:
CANDIDATE.h
#include
#include "personType.h"
using namespace std;
const int NO_OF_REGIONS = 4;
class candidateType: public personType
{
public:
const candidateType& operator=(const candidateType&);
//Overload the assignment operator for objects of the
//type candidateType
const candidateType& operator=(const personType&);
//Overload the assignment operator for objects so that
//the value of an object of type personType can be
//assigned to an object of type candidateType
void updateVotesByRegion(int region, int votes);
//Function to update the votes of a candidate for a
//particular region.
//Postcondition: Votes for the region specified by the
// parameter are updated by adding the votes specified
// by the parameter votes.
void setVotes(int region, int votes);
//Function to set the votes of a candidate for a
//particular region.
//Postcondition: Votes for the region specified by the
// parameter are set to the votes specified by the
// parameter votes.
void calculateTotalVotes();
//Function to calculate the total votes received by a
//candidate.
//Postcondition: The votes in each region are added and
// assigned to totalVotes.
int getTotalVotes() const;
//Function to return the total votes received by a
//candidate.
//Postcondition: The value of totalVotes is returned.
void printData() const;
//Function to output the candidate's name, the votes
//received in each region, and the total votes received.
candidateType();
//Default constructor.
//Postcondition: Candidate's name is initialized to blanks,
// the number of votes in each region, and the total
// votes are initialized to 0.
//Overload the relational operators.
bool operator==(const candidateType& right) const;
bool operator!=(const candidateType& right) const;
bool operator<=(const candidateType& right) const;
bool operator<(const candidateType& right) const;
bool operator>=(const candidateType& right) const;
bool operator>(const candidateType& right) const;
private:
int votesByRegion[NO_OF_REGIONS]; //array to store the votes
// received in each region
int totalVotes; //variable to store the total votes
};
#endif
PERSONTYPE.h
#include
#include
using namespace std;
class personType
{
//Overload the stream insertion and extraction operators.
friend istream& operator>>(istream&, personType&);
friend ostream& operator<<(ostream&, const personType&);
public:
const personType& operator=(const personType&);
//Overload the assignment operator.
void setName(string first, string last);
//Function to set firstName and lastName according to
//the parameters.
//Postcondition: firstName = first; lastName = last
string getFirstName() const;
//Function to return the first name.
//Postcondition: The value of firstName is returned.
string getLastName() const;
//Function to return the last name.
//Postcondition: The value of lastName is returned.
personType(string first = "", string last = "");
//constructor with parameters
//Set firstName and lastName according to the parameters.
//Postcondition: firstName = first; lastName = last
//Overload the relational operators.
bool operator==(const personType& right) const;
bool operator!=(const personType& right) const;
bool operator<=(const personType& right) const;
bool operator<(const personType& right) const;
bool operator>=(const personType& right) const;
bool operator>(const personType& right) const;
protected:
string firstName; //variable to store the first name
string lastName; //variable to store the last name
};
#endif
ORDEREDARRYLIST.h
#ifndef H_OrderedListType
#define H_OrderedListType
#include
#include "arrayListType.h"
using namespace std;
template
class orderedArrayListType: public arrayListType
{
public:
void insertOrd(const elemType&);
int binarySearch(const elemType& item);
orderedArrayListType(int size = 100);
};
template
void orderedArrayListType
{
int first = 0;
int last = length - 1;
int mid;
bool found = false;
if (length == 0) //the list is empty
{
list[0] = item;
length++;
}
else if (length == maxSize)
cerr << "Cannot insert into a full list." << endl;
else
{
while (first <= last && !found)
{
mid = (first + last) / 2;
if (list[mid] == item)
found = true;
else if (list[mid] > item)
last = mid - 1;
else
first = mid + 1;
}//end while
if (found)
cerr << "The insert item is already in the list. "
<< "Duplicates are not allowed." << endl;
else
{
if (list[mid] < item)
mid++;
insertAt(mid, item);
}
}
}//end insertOrd
template
int orderedArrayListType
(const elemType& item)
{
int first = 0;
int last = length - 1;
int mid;
bool found = false;
while (first <= last && !found)
{
mid = (first + last) / 2;
if (list[mid] == item)
found = true;
else if (list[mid] > item)
last = mid - 1;
else
first = mid + 1;
}
if (found)
return mid;
else
return 1;
}//end binarySearch
template
orderedArrayListType
: arrayListType
{
}
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
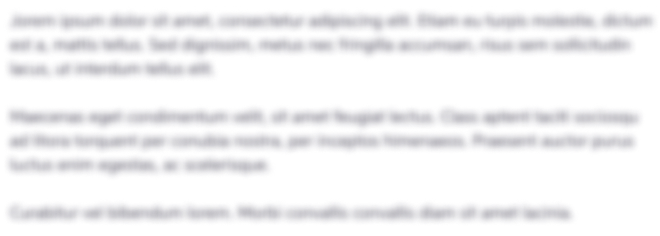
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started