Question
WRITING A VECTOR CLASS In this homework, youll get to write your very own vector-type class. The class will behave as a std::vector object, but
WRITING A VECTOR CLASS In this homework, youll get to write your very own vector-type class. The class will behave as a std::vector object, but you are not allowed to use the standard library vector templated class. In doing this assignment, youll be able to better understand how std::vector works behind the scenes.
You should write a class object of type VectorString, belonging to a pic10b namespace, that can store objects of type std::string.
The class should have the following:
member variables vec size, representing the number of elements in the vector, and vec capacity, storing the size of the underlying dynamic array in memory, which must always be at least as big as vec size (see later remarks);
a member variable storing a smart pointer variable to the dynamic array of std::string;
a default constructor VectorString() that allocates a dynamic array of std::string of one element (so vec capacity is 1), but which has a vec size of 0;
a constructor VectorString(size t) that allocates a dynamic array of twice the input size: vec size should be set to the input and vec capacity is twice the input with all of the string variables set to the empty string;
a constructor accepting a size and input string value, that does the same as above with the input size and capacity, but which initializes all the string variables to the input string;
a copy constructor VectorString(const VectorString&) and move constructor VectorSring(VectorString&&);
a size member function returning the size of the vector;
a capacity member function returning the capacity of the vector;
a push back member function accepting a std::string that adds an element to the end of the vector if the capacity allows and otherwise creates a new dynamic array of twice the former capacity, moving all the elements over and adding the new element;
a pop back member function that removes the last element of the vector by updating its vec size value;
a deleteAt member function accepting an index value that removes the element at the given index from the vector, shifting elements backwards as appropriate;
an insertAt member function accepting an index and std::string value that inserts an element at the input position, moving elements (including the element previously there) forward and when this would push the vector size above the capacity then all the elements should be moved over to a new dynamic array of twice the former capacity before this process;
and an at member function accepting an index value that returns the element at the given index by reference.
At this point in the course it should only work on nonconst VectorString objects. And do not worry about throwing exceptions as the real std::vector::at function does. Not yet...
Note that when the size exceeds the capacity then the elements are re-located to a larger block of memory with twice the former capacity! The vector is indexed from 0.
Your job is to write a header file VectorString.h and accompanying file VectorString.cpp with the implementations so that a .cpp file can use the VectorString class. An example set of code and output are given below: your actual homework will be graded against a different .cpp file.
Do not submit a main routine! The only header files that you may use are:
Once again: do not submit a main routine! You may assume the user will only enter valid parameters, i.e., use indices that are within range with at; they wont pop back on an
Example code is below
#include"VectorString.h"
#include
#include pic10b::VectorString foo() {
return pic10b::VectorString(2);
}
int main(){ pic10b::VectorString vs; std::cout << "vs stats: " << vs.size() << " " << vs.capacity() << ; vs.push_back("hello"); std::cout << "vs stores: " << vs.at(0) << ; pic10b::VectorString vs2(foo()); std::cout << "vs2 stats: " << vs2.size() << " " << vs2.capacity() << ; std::cout << "vs2 stores: "; for (std::size_t i = 0, total_size = vs2.size(); i < total_size; ++i) { std::cout << vs2.at(i) << ","; 3 } std::cout << ; pic10b::VectorString vs3(4, "beta"); vs3.pop_back(); vs3.push_back("delta"); vs3.push_back("epsilon"); vs3.at(1) = "gamma"; vs3.insertAt(0, "alpha"); vs3.push_back("zeta"); vs3.push_back("theta"); vs3.insertAt(vs3.size() - 1, "eta"); vs3.deleteAt(3); std::cout << "v3 stats: " << vs3.size() << " " << vs3.capacity() << ; std::cout << "v3 stores: "; for (std::size_t i = 0, total_size = vs3.size(); i < total_size; ++i) { std::cout << vs3.at(i) << ","; } std::cin.get(); ret
Remarks: Besides the facts that we havent templated the class (which will be covered later) and that our dynamic memory management can be improved (also later), this is very similar to how the real std::vector works. With a real std::vector, a special class object called an allocator allocates unconstructed heap memory, i.e., the memory cells, unless filled with legitimate vector elements, are not even constructed by a default constructor. Implementing the copy constructor is a little delicate. A real copy constructor making use of smart pointers needs to take into account not only the memory managed but also the deleter. This will be a detail this isnt pursued in this assignment. For the copy constructor, we will just worry about copying values in memory.
Some initial guidance to get you on your way...
1. First make sure you can create a dynamic array of std::strings and work with them with a smart pointer as a wrapper.
2. You are working with smart pointers so you dont need to worry about the destructor unless you do something really strange with your memory.
3. To copy, youll need to create a new dynamic array (with a smart pointer wrapping it) of the appropriate size and then copy each element over one at a time.
4. For push back, if the new size does not go beyond the capacity, all you need to do is subscript your smart pointer at the new size value and increase the size; if you need to create more memory, be sure to keep track of the location in memory where your smart pointer used to point (you may need to use release).
5. For pop back, you need only decrease the size. This effectively chops off the last element.
6. Draw yourself a diagram of how the insertAt and deleteAt functions work. Youll have to shuffle memory around the old fashioned way instead of using the insert and erase functions provided by std::vectors.
The PIC 10A notes on vectors may be helpful.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
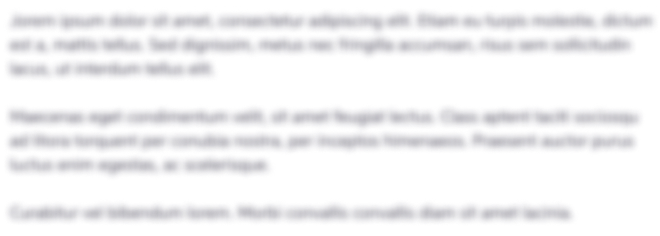
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started