Question
Writing and executing your first MIPS assembly language program: All MIPS assembly language programs that you write should have a .asm extension. For example, lab1.asm.
Writing and executing your first MIPS assembly language program:
-
All MIPS assembly language programs that you write should have a .asm extension. For example, lab1.asm. To create an assembly file on your PC do the following:
-
Click File -> New. An empty file will be opened in the Edit window. Type your program.
-
Save the file in the directory where you want it by clicking File -> Save.
-
-
Type the program given below in a file and save it. Please note the format and structure of the code. These programs are very difficult to read so try to format your code in the same way in the future.
############################################## # Program Name: Maximum of two integers # Programmer: (Your name) # Date (...) ############################################## # Functional Description:
# A program to read 2 integer numbers and print out the larger one ##############################################
.data #Data section
Prompt1: .asciiz " Enter the first number: " Prompt2: .asciiz "Enter the second number: " Result: .asciiz "The larger number is: "
.globl main .text #Code section
main:
li $v0, 4 la $a0, Prompt1 syscall
li $v0, 5 syscall move $t0, $v0
li $v0, 4 la $a0, Prompt2 syscall
li $v0, 5 syscall
#system call code for Print String #load address of Prompt1 into $a0 #print the Prompt1 message
#system call code for Read Integer #reads the value of 1st integer into $v0 #move the 1st integer to $t0
#system call code for Print String #load address of Prompt2 into $a0 #print the Prompt2 message
#system call code for Read Integer #reads the value of 2nd integer into $v0
move $t1, $v0 #move the 2nd integer to $t0
sgt $s0, $t0, $t1 bne $s0, $zero, Print_result move $t0, $t1
Print_result:
li $v0, 4 la $a0, Result syscall
li $v0, 1 move $a0, $t0 syscall
li $v0, 10 syscall
# END OF PROGRAM
#Check which integer is greater #Branch to Print_result if $t0 is larger #Else: $t1 is larger
#System call code for Print String #load address of Result into $a0 #print the string
#system call code for Print Integer #move value to be printed to $a0 #print sum of integers
#terminate program run and #return control to the system
3. Running the program:
-
First assemble the code by selecting Run -> Assembly from the menu bar.
The result is shown in the text display. You will see the addresses of the memory locations of instructions, the machine instructions in hex, the assembly language program source and some extra code automatically added by the assembler.
-
Run the code using either the Run -> Go option, which will execute the program to completion, or the Run -> Step option, which will execute only one instruction at a time. The start address must be 0x00400000. This is a hexadecimal value, and it is always the start address for your programs. This will cause the program counter register (PC) to get the address 0x00400000. Note: If your program goes into an infinite loop use the Stop button. Look at each of the display sections of the simulator. The register display shows the contents of all registers in the MIPS CPU. This display is updated when your program stops running.
The text display shows your instructions. A line in this display looks like this: Address Code Basic Source
0x00400000 0x8fa40000 lw $4, 0($29) 183: lw $a0 0($sp)
The first column shows the memory address of your instruction. Note that each instruction is one word or four bytes long. Note how the addresses change from one instruction to the next. The next column is the actual machine language
instruction in hex. The third is your instruction, changed a little so that the machine can use it. The last column is what you typed in as the instruction. The number before the colon is the actual line number from your assembly language program. Sometimes, you will see nothing in the last (source) column. This means that the instruction was produced by MARS as part of translating a pseudo instruction.
You can reset the memory and registers before running the program. You can run a program a second time without reinitializing or clearing registers, but I do not advise this because the current state of the program may cause it to run improperly.
To refer to ASCII codes: https://bluesock.org/~willg/dev/ascii.html. Answer the following questions about the program that you entered:
-
At what address does your code start?
-
At what line number does your code start?
-
At what address does your code finish?
-
At what address does the text segment start?
-
At what address does the data segment start?
-
For input values- 1st number: 987 and 2nd number: 321, Use F7 or the single-
step button to single-step through the program. Does your program jump from one line of code to another line other than the next line of the program? Give the address that the program jumps from and the address that it jumps to for the first such occurrence. Why do you think this happens?
-
Reset the memory and registers and assemble the program again. After assembling the program, but before executing it, record the values in the following registers: pc, $at, $v0, $a0, $t0 and $ra.
-
Execute the program, Enter the values of 1st number: 112 and 2nd number: 345, and record the values in the registers listed in question 7.
Note: You only need to submit the answers to the questions and screenshot of the Run I/O display with input values of Question 8.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
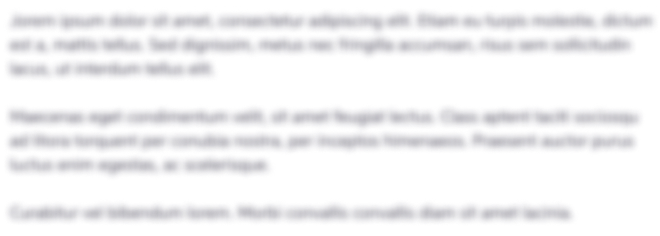
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started