Question
Writing in JAVA code: Using a random die-rolling technique such as those developed in class (available somewhere in the Files section, or see Exercise 2.2
Writing in JAVA code:
Using a random die-rolling technique such as those developed in class (available somewhere in the Files section, or see Exercise 2.2 in the book) write a program that simulates rolling a die 1,000,000 times, and prints the longest string of the same number.
Ex. It rolls a 1, 2, 2, 2, 4, 3, 5, 5, 1, 6, 2 and outputs "3", because it rolled three 2's in a row. (this would be a very unusual outcome)
Use methods appropriately, I recommend at least one for the 'rolling a die' part. As a note, you should expect values around 7. You don't need to print out every roll of the dice.
I have created a method for rolling the die:
public static int rollDie(int numSides) { Random myRandom = new Random(); double randomNumber = myRandom.nextDouble(); randomNumber *= numSides; int dieValue = (int)randomNumber + 1; return dieValue; }
and have the loop set up to roll it 1,000,000 times in my main method:
for (int k = 0; k < 1_000_000; k++) { rollDie(6); }
However, I do NOT understand how to print out the longest string of the same number. I think you need to check if each individual die value is equal to the one before, and then increase a counter to keep track of how many of the same numbers there are, but I do not understand how to work this into the code?? I believe this should be done in the main method, as well, but I am not sure?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
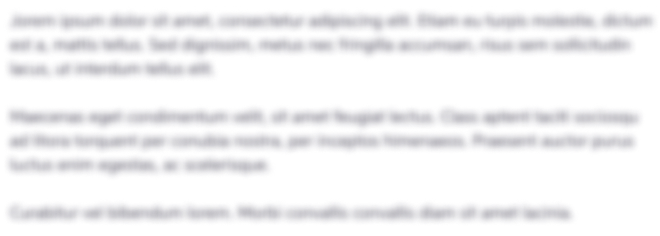
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started