Question
Written in C or C++ How does the Producer-Consumer problem work? We will have several consumer threads and several producer threads and one buffer. Each
Written in C or C++
How does the Producer-Consumer problem work?
We will have several consumer threads and several producer threads and one buffer. Each producer creates widgets and inserts them into the buffer, one at a time. Each consumer removes widgets from the buffer, one at a time. As the buffer is a fixed size, we need to ensure that:
(a) no producer tries to insert a widget into the buffer when it is full, and
(b) no consumer tries to remove a widget from the buffer when it is empty.
We will not actually deal in widgets and a buffer. Instead, we will simply maintain an integer called Counter to keep track of how many widgets are in the buffer at any moment. Thus, the action of inserting a widget is incrementing Counter and the action of removing a widget is decrementing Counter.
We do not want to have two threads attempting to change the value of the counter at the same time, so we will use a mutex to limit access to it. Thus we can describe the actions as:
Insert a widget:
Lock the mutex
Increment Counter
Unlock the mutex
Remove a widget:
Lock the mutex
Decrement Counter
Unlock the mutex
We will also use two semaphores, NotFull and NotEmpty. Before we start, NotFull is initalized to BUFFER_SIZE and NotEmpty is initialized to 0. (The textbook refers to NotFull and NotEmpty as Empty and Full, respectively.)
If NotFull is not 0, (think of that as "true") then the buffer is not full, so there is space left in the buffer and a producer can insert a widget. If NotFull is 0 (think of that as "false"), then the buffer is full, so the producer must wait until NotFull is positive before it can insert a widget and then decrement NotFull.
If NotEmpty is not 0 (think of that as "true"), then the buffer is not empty, so there is at least one Widget in the buffer and a consumer can remove a widget. If NotEmpty is 0 (think of that as "false"), then the buffer is empty and the consumer must wait until NotEmpty is not 0 before it can remove an object and then decrement NotEmpty.
Notice that whenever we insert or remove a widget, Counter and NotFull and NotEmpty are all affected.
It may sound as if we have three counters for the buffer. In a sense, we do, but they are for different purposes. Everyone shares Counter. The NotFull semaphore is oriented to the producer's point of view: is there space to insert a widget? The NotEmpty semaphore is oriented to the consumer's point of view: is there a widget to remove? The fact that these are semaphores gives us the convenience of the wait() function (go into a wait state instead of busy-waiting).
Here is what the producer is doing each time:
wait(NotFull)
Insert()
post(NotEmpty)
Here is what the consumer is doing each time:
wait(NotEmpty)
Remove()
post(NotFull)
---------------------------------------------------------------------
What do we need in the program?
The program should have #defines for 5 constants:
P_NUMBER = the number of producers = 6
C_NUMBER = the number of consumers = 4
BUFFER_SIZE = the maximum size of the buffer = 12
N_P_STEPS = the number of iterations for each producer thread = 4
SAMPLE OUTPUT
Simulation of Producer and Consumers
The semaphores and mutex have been initialized.
Producer 0 inserted one item. Total is now 1
Producer 5 inserted one item. Total is now 2
Producer 2 inserted one item. Total is now 3
Consumer 2 removed one item. Total is now 2
Producer 3 inserted one item. Total is now 3
Producer 1 inserted one item. Total is now 4
Consumer 0 removed one item. Total is now 3
Consumer 1 removed one item. Total is now 2
Producer 4 inserted one item. Total is now 3
Consumer 3 removed one item. Total is now 2
Producer 0 inserted one item. Total is now 3
Producer 2 inserted one item. Total is now 4
Consumer 2 removed one item. Total is now 3
Producer 3 inserted one item. Total is now 4
Producer 1 inserted one item. Total is now 5
Producer 5 inserted one item. Total is now 6
Consumer 0 removed one item. Total is now 5
Consumer 1 removed one item. Total is now 4
Producer 4 inserted one item. Total is now 5
Consumer 3 removed one item. Total is now 4
Producer 2 inserted one item. Total is now 5
Producer 0 inserted one item. Total is now 6
Producer 3 inserted one item. Total is now 7
Consumer 2 removed one item. Total is now 6
Consumer 0 removed one item. Total is now 5
Producer 1 inserted one item. Total is now 6
Consumer 1 removed one item. Total is now 5
Producer 5 inserted one item. Total is now 6
Producer 4 inserted one item. Total is now 7
Consumer 3 removed one item. Total is now 6
Producer 0 inserted one item. Total is now 7
Producer 3 inserted one item. Total is now 8
Consumer 2 removed one item. Total is now 7
Producer 2 inserted one item. Total is now 8
Producer 1 inserted one item. Total is now 9
Consumer 0 removed one item. Total is now 8
Consumer 1 removed one item. Total is now 7
Producer 5 inserted one item. Total is now 8
Producer 4 inserted one item. Total is now 9
Consumer 3 removed one item. Total is now 8
Consumer 2 removed one item. Total is now 7
Consumer 0 removed one item. Total is now 6
Consumer 1 removed one item. Total is now 5
Consumer 3 removed one item. Total is now 4
Consumer 2 removed one item. Total is now 3
Consumer 0 removed one item. Total is now 2
Consumer 1 removed one item. Total is now 1
Consumer 3 removed one item. Total is now 0
All the producer and consumer threads have been closed.
The semaphores and mutex have been deleted.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
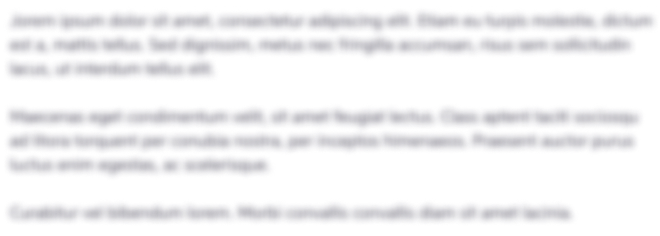
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started