Question
You are asked to write a Java program about the classic game called Mastermind. In this the character R, P, O, Y, G and B,
You are asked to write a Java program about the classic game called Mastermind. In this the character R, P, O, Y, G and B, are used to represent the colours red, pink, orange, yellow, green and blue of the code pegs respectively. At the beginning of the program, the code maker, i.e., the program itself, will randomly generate a secret code consists of 4 pegs. In each round, the code breaker, i.e., the player, will guess the secret code by inputting 4 characters separated by space. The code maker will then check if the code pegs in the guess are the same as the code pegs in the secret code. If the guess and the secret code are the same, the player win. Otherwise, the code maker will give hints using 0 or 4 key pegs to the code breaker as follows: The number of BLACK key pegs refers to the number of code pegs in the guess code that are the same to the code pegs in the secret code in terms of colour and position; The number of WHITE key pegs refers to the number of code pegs in the guess code that have the same colour as the code pegs in the secret code. For example, if the secret code is R O G Y and the guess code is R Y O B, the key pegs are 1 BLACK and 2 WHITE because the first peg is R which is the same as the first peg in the secret code; the second and third pegs are found in the secret code, but in a different position; the fourth peg is incorrect.
You have to implement two classes, namely, CodeMaker and CodeBreaker according to the members and method headers as listed below:
public class CodeMaker { // A member variable of Code to store the secret code private Code secretCode; // getter of secretCode public Code getSecretCode() { } // setter of secretCode public void setSecretCode(Code secretCode) { } // default constructor public CodeMaker() { } // this method generate the secret code and store the secret code // in the member variable secretCode. The secret code generated will // not have duplicated pegs. For example, it is not allowed to have // the secret code "R P P O" because "P" is a duplicate. public void makeSecretCode() { } }
import java.util.Scanner; public class CodeBreaker { // A member variable of Code to store the guess code Code guessCode; // default constructor public CodeBreaker() { } // getter of guessCode public Code getGuessCode() { } // setter of guessCode public void setGuessCode(Code guessCode) { } // This is a method to get the guess code from the player, and store // the input to guessCode. public void getGuessCodeInput() { }}
In order to run the program, two other classes, namely, MastermindGame and Code.
public class MastermindGame { // The number of possible by guess public static final int MAX_GUESS = 12; // The main program public static void main(String[] args) { // create the CodeMaker and CodeBreaker objects CodeMaker cm = new CodeMaker(); CodeBreaker cb = new CodeBreaker(); // generate the secret code cm.makeSecretCode(); System.out.println("Welcome to Mastermind."); System.out.print("I generated a secrete code. Please guess (R, P, O, Y, G, B): "); for (int i = 0; i < MAX_GUESS; i++) { // get player's input cb.getGuessCodeInput(); // match the input and the secret code if (cm.getSecretCode().compare(cb.getGuessCode())) { System.out.println("Congratulations! You win!"); return; } // find the hints by coder maker int numBlack = cm.getSecretCode().getNumBlackKeyPegs(cb.getGuessCode()); int numWhite = cm.getSecretCode().getNumWhiteKeyPegs(cb.getGuessCode()); System.out.println("Sorry! It is not correct."); System.out.println("Hints: " + numBlack + " Black and " + numWhite + " White"); if (i != MastermindGame.MAX_GUESS - 1) { System.out.print("Please guess again (" + (MastermindGame.MAX_GUESS - i - 1) + " guesses left): "); } } System.out.println("Sorry! You loss."); System.out.print("The secrete code is: "); cm.getSecretCode().printCode(); } }
public class Code { private static final int NUM_COLORS = 6; private static final int NUM_PEGS = 4; private static final char[] COLORS = { 'R', 'O', 'Y', 'G', 'B', 'P' }; // The four pegs private char[] pegs; // Default constructor public Code() { pegs = new char[4]; } // A constructor that a Code object using four pegs public Code(char p1, char p2, char p3, char p4) { pegs = new char[4]; setPeg(p1, 0); setPeg(p2, 1); setPeg(p3, 2); setPeg(p4, 3); } // Make a random code. The code may contain duplicated pegs. public void makeRandomCode() { for (int i = 0; i < NUM_PEGS; i++) { char color = COLORS[(int) (Math.random() * Code.NUM_COLORS)]; setPeg(color, i); } } // set the four pegs public void setPegs(char p1, char p2, char p3, char p4) { setPeg(p1, 0); setPeg(p2, 1); setPeg(p3, 2); setPeg(p4, 3); } // check if there is duplicated pegs in the code public boolean hasDuplicatedPegs() { for (int i = 0; i < NUM_PEGS - 1; i++) { for (int j = i + 1; j < NUM_PEGS; j++) { if (pegs[i] == pegs[j]) return true; } } return false; } // return true if the code of this object is the same as the code of c. // return false otherwise. public boolean compare(Code c) { for (int i = 0; i < NUM_PEGS; i++) { if (getPeg(i) != c.getPeg(i)) return false; } return true; } // return the number of black key pegs by comparing this object and c. public int getNumBlackKeyPegs(Code c) { int count = 0; for (int i = 0; i < NUM_PEGS; i++) { if (getPeg(i) == c.getPeg(i)) count++; } return count; } // return the number of white key pegs by comparing this object and c. public int getNumWhiteKeyPegs(Code c) { char[] temp1 = { pegs[0], pegs[1], pegs[2], pegs[3] }; char[] temp2 = { c.getPeg(0), c.getPeg(1), c.getPeg(2), c.getPeg(3) }; int count = 0; for (int i = 0; i < NUM_PEGS; i++) { for (int j = 0; j < NUM_PEGS; j++) { if (temp1[i] != '\0' && temp2[j] != '\0' && temp1[i] == temp2[j]) { count++; temp1[i] = '\0'; temp2[j] = '\0'; } } } return count - getNumBlackKeyPegs(c); } // print out the code public void printCode() { System.out.println(toString()); } // setter of pegs public void setPegs(char[] pegs) { this.pegs = pegs; } // getter of pegs public char[] getPegs() { return this.pegs; } // set an individual peg given the position pos (pos starts from 0) public void setPeg(char peg, int pos) { this.pegs[pos] = peg; } // get an individual peg give the position pos public char getPeg(int pos) { return this.pegs[pos]; } @Override public String toString() { String str = ""; for (int i = 0; i < NUM_PEGS; i++) { str += this.pegs[i] + " "; } return str.trim(); // remove the ending space } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
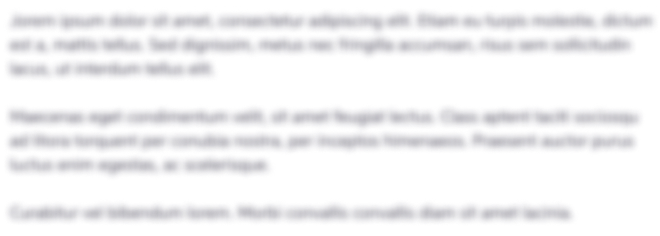
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started